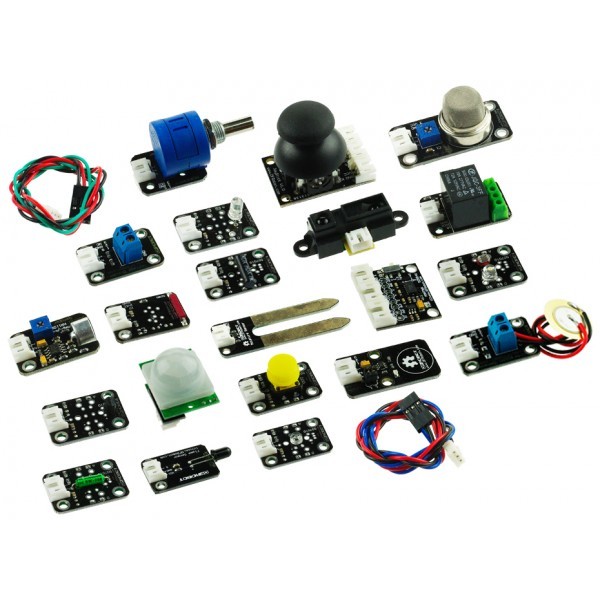
Input Devices: Collect data!
In this week we are supposed to design, fabricate, and test an electronic input device. Input devices are the sensors that collects the required data for whatever application it is for, example of that is ultrasonic sensore, which is used to colloect data of a certain distance.
For this assignment I decided to work on something related to my final project, which is the Joystick Module input device. The joystick module, like a controller for a game console, allows you to control X, Y, and Z dimensions. Data types X and Y serve as analog input signals, allowing them to measure different levels of resistance. I am going to use the Joystick module to control the 3D scanner arm rotation around the object.
Schematic Design
The first thing to do for this assignment is to analyze the schematic for the Joystick module to know exactly how it is going to be connected to the microcontroller.
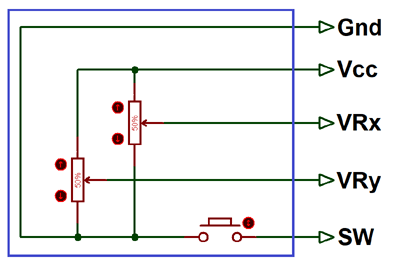
The above image is the internal diagram of a Joystick Module. It consists of two Potentiometer, each for one axis (X and Y). Both 10k potentiometer are independent to move in their particular direction. SW (Switch) pin is connected to a push button internally.
Joystick Module can be used with Arduino, Raspberry Pi and any other Micro-controllers. We just have to connect the axis Pins VRx and VRy to the ADC Pins of the micro-controller. And if we want to use the switch then we should connect it to the digital Pin of the Micro-controller.
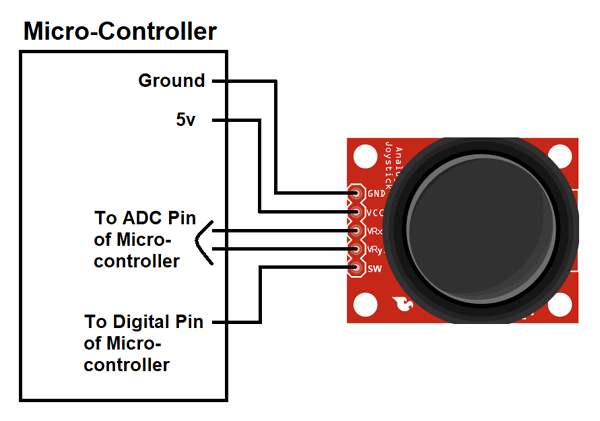
The interfacing diagram of Joystick Module with the Arduino is shown below. This diagram helps to connect the joystick Module with Arduino and get the analog output based on the direction of movement of Joystick Knob.
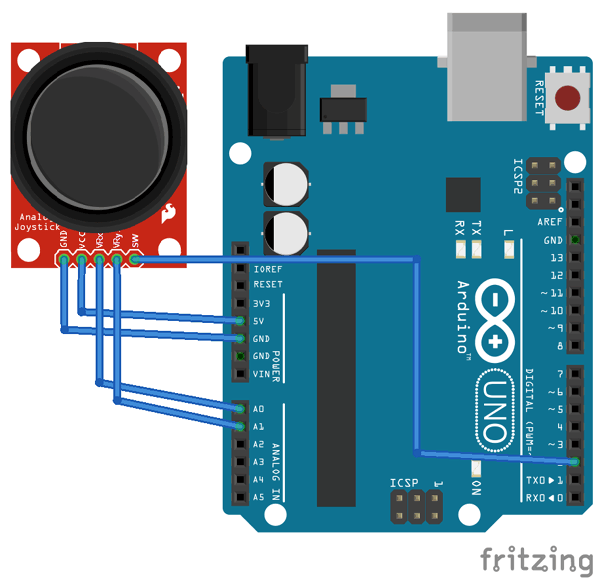
After Interfacing Joystick Module with the Arduino, we will get the analog output. The output range is fixed for each direction. The below image shows, the value of analog output for X and Y axis based on the movement of Joystick Module in all four directions (+X, -X, +Y, -Y). Based on this we will also get some analog value when moving the knob diagonally.
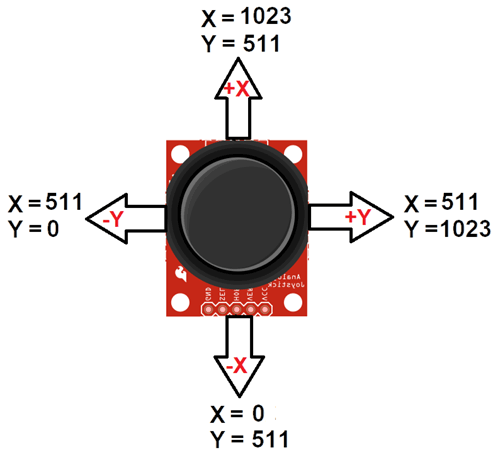
To test the joystick I used commercial arduino board to test my connection and the output results from the Joystick module as an input device, before designing and fabricating your own PCB board. The code was the following:
// Arduino pin numbers const int SW_pin = 2; // digital pin connected to switch output const int X_pin = 0; // analog pin connected to X output const int Y_pin = 1; // analog pin connected to Y output void setup() { pinMode(SW_pin, INPUT); digitalWrite(SW_pin, HIGH); Serial.begin(115200); } void loop() { Serial.print("Switch: "); Serial.print(digitalRead(SW_pin)); Serial.print("\n"); Serial.print("X-axis: "); Serial.print(analogRead(X_pin)); Serial.print("\n"); Serial.print("Y-axis: "); Serial.println(analogRead(Y_pin)); Serial.print("\n\n"); delay(500); }
Running the code will give us the following results:
1. Moving the Joystick to +x direction.
2. Moving the Joystick to -x direction.
3. Moving the Joystick to +y direction.
4. Moving the Joystick to -y direction.
After making sure that the Joystick module is working perfectly with the arduino commercial board, it is know time to design my own fabduino using eagle software.
To design my Fabaduino I decided to use the Atmega328-P microcontroller that has the following schematic diagram:
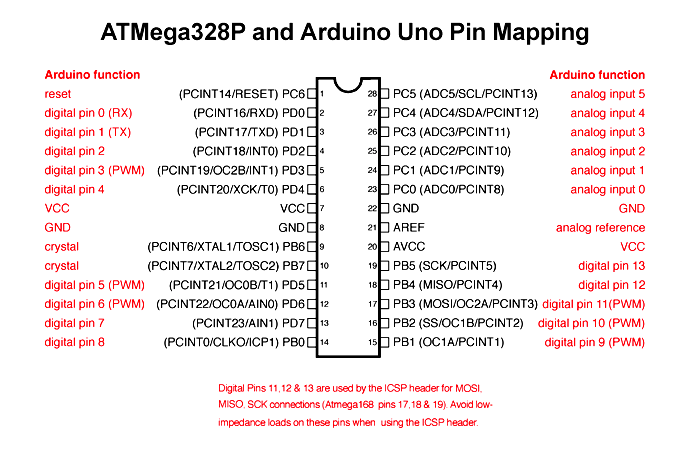
Since I did not have the library for the Atmega328-P microcontroller, I had to download it from Adafruit-Eagle-Library-master and I added the library from the library manager in Eagle.
After adding the library, the component can be found under Adafruit -> ATMEGA168*P -> ATMEGA168-20P. You will notice, it is not exactly the Atmega328, but the Atmega168 has the exact same package type and pinout so they can be used interchangeably for Eagle design purposes.
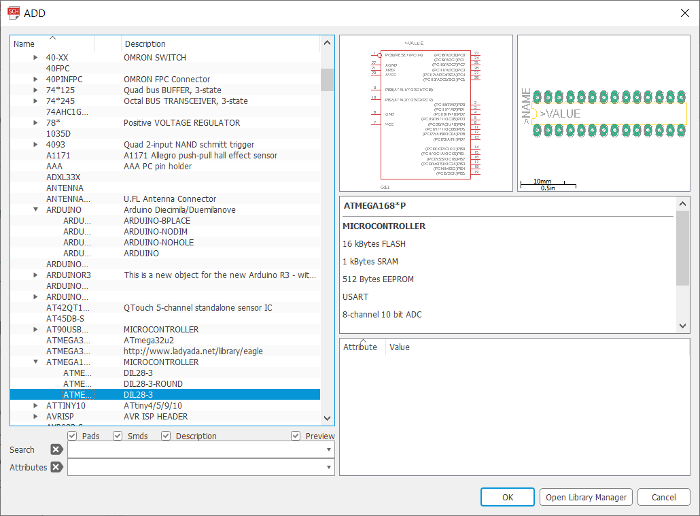
Then after that I started to add components, like crystal oscilator because Atmega328-P crystals are used to help when the microconroller is dealing with time issues, for example if we build a project that turns a switch A OFF after 15 minutes ON and turn ON switch B, C & D one after another with a 20 minutes step. We can use Arduino software to program the chip to do that, but how Atmega328-P calculate the time is by using this crystal, the number on the top of the crystal is 16.000H9H this gives us information about the frequency which is 16,000,000 Hertz or 16 Mhz, this small component can make 16 millions cycles per second so using this concept Arduino can calculate time. The crystal is used along with two capacitor of 22pF value, and connected to pin PB6 and PB7 in the atmega328-p.
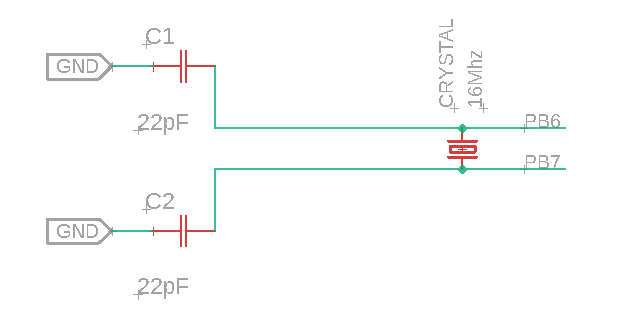
Then I connected a pull up resistor, and switch button with the reset pin of atmega328-p, this is important to be able to program the board.
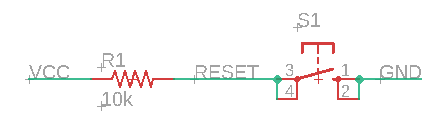
After that I created the pins I require for my connections, like the joystick pins, programming pins, and power supply pin. Also I added pins for NRF24L01 module, which is something I am going to use in the future for my final project.
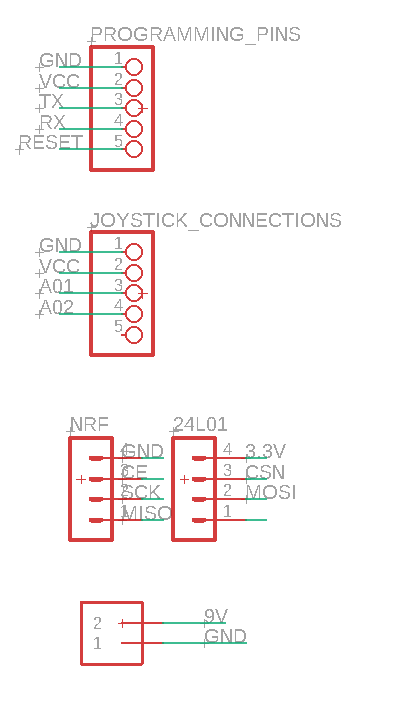
I also added two voltage regulators, one to regulate the 9V to 5V to supply the entire circuit, and the other one is to regulate 5V to 3.3V to supply the NRF24L01 module.
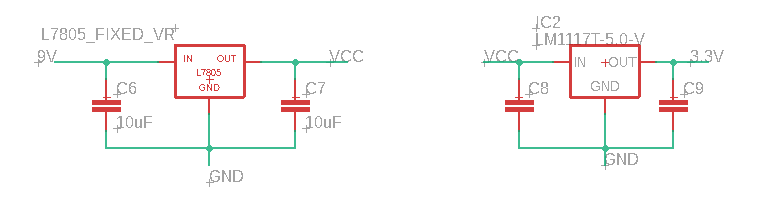
Finally I added LED connected to VCC to indicate when the circuit is supplied by voltage.
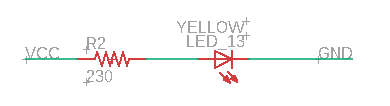
After I checked that my connections are all fine I started doing the routing for the PCB.
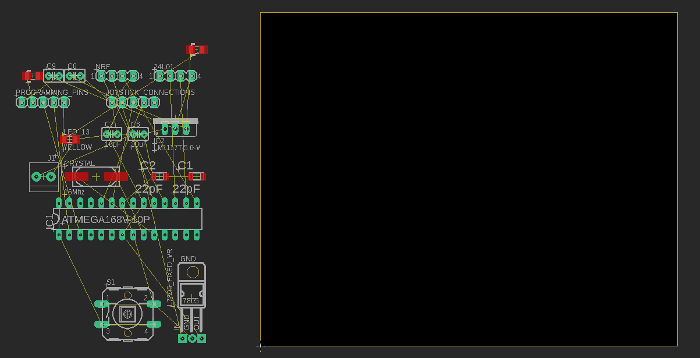
After around one hour of trying I was able to finish the routing of the circuit and it is know ready to be fabricated and programmed.
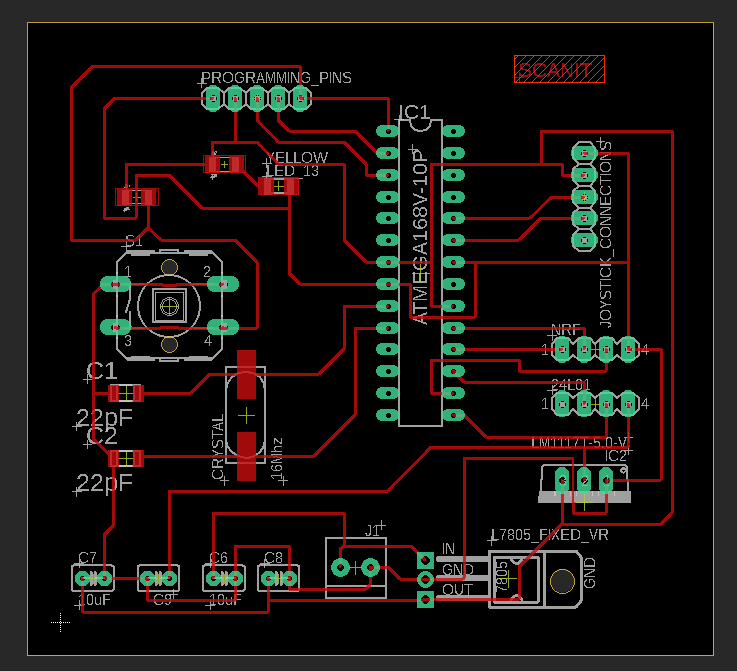
Circuit Fabrication
Before Fabricating the circuit, we should first put it in FabModules to prepare the files for the milling process. With that being said, I first prepared the traces milling file as shown below:
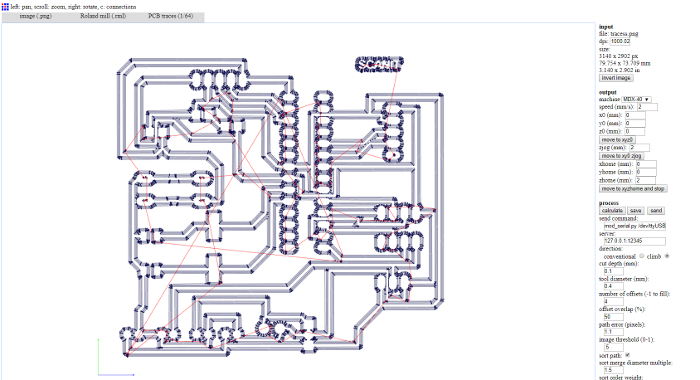
The drilling file:
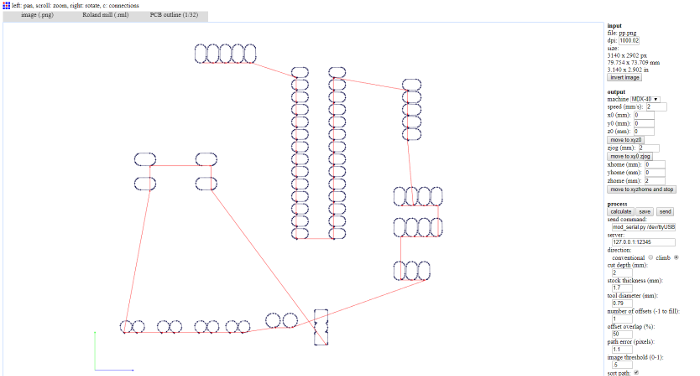
And finally the outline file:
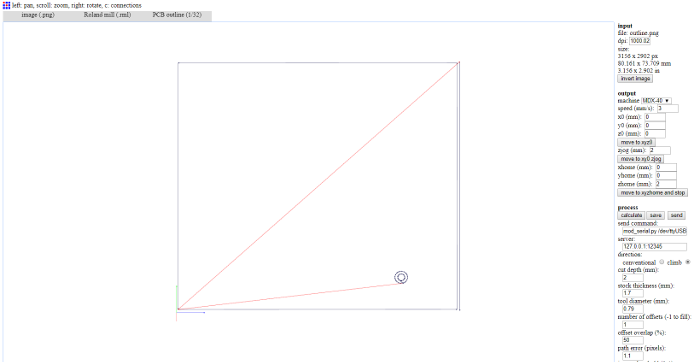
Then after I prepared my files, I uploaded them in Roland MDX to start the fabrication process. The PCB took around 45 minutes in fabrication, but it turned out good from the first shot.
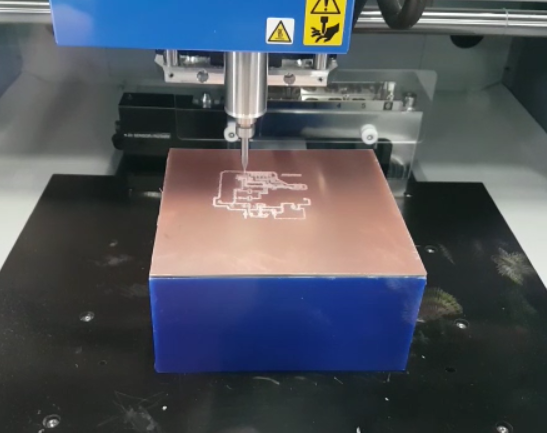
The components and the fabricated PCB of the input device is the following:
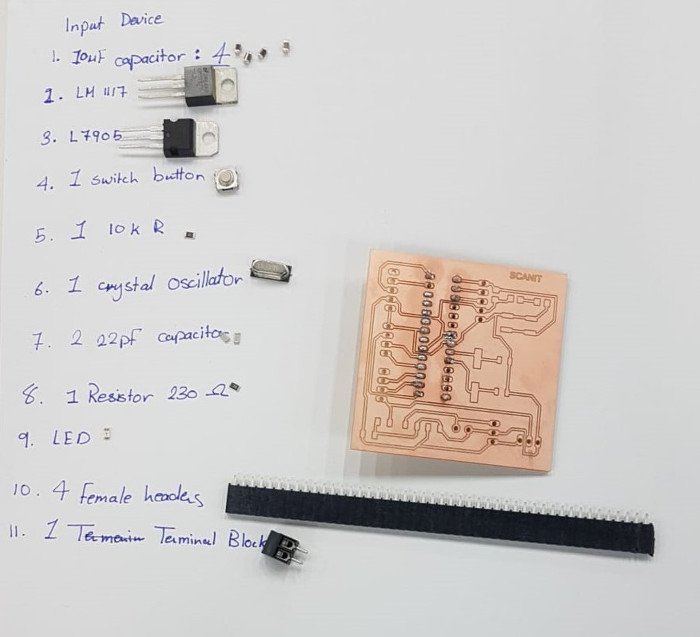
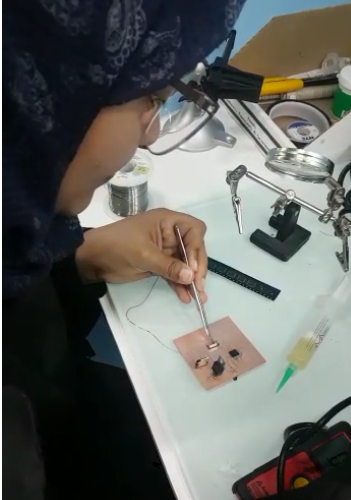
Testing
The final testing of the input device worked perfectly with the fabricated PCB:
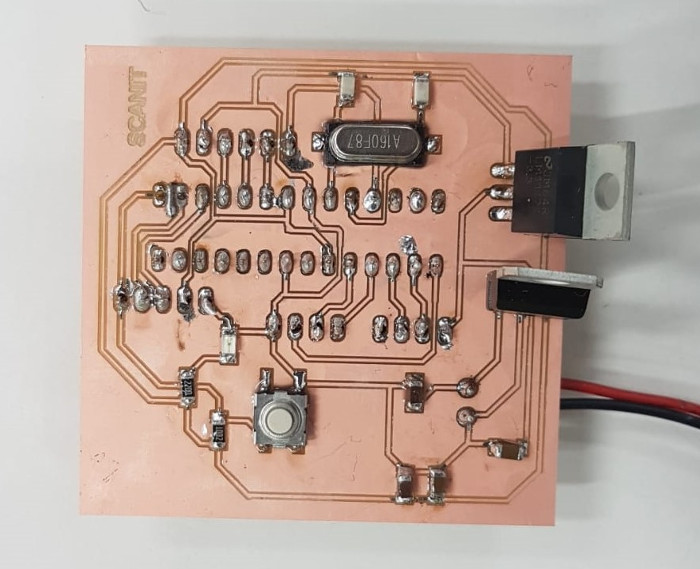