Machaine build : D(draw)-wallbot
Here is our group works : week17
Reference : Link
https://github.com/MertArduino/Make-Arduino-XY-Plotter-Drawing-Robot
What I have contributed
1.Motor Operation with A4988 Driver
2.Set Arduino Code
- Test it until it works.
3.Test motor operation using Arduino Unoboard
4. Put a Vactor file in the processing to link with Arduino
5. Create a code for connecting the main board and two motor drives to a network
First, we checked how the stepping motor, sub-motor and motor driver were connected and tested using the Arduino Unoboard.
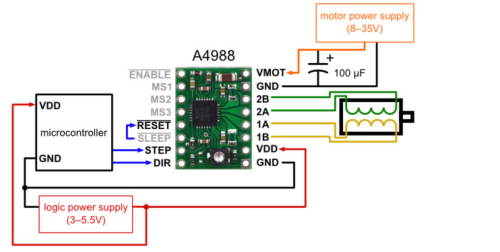
I was able to check each pinout required for the stepping motor's drive.If the pin connected to the motor is reversed, it can turn the other way around, so it had to be checked carefully.
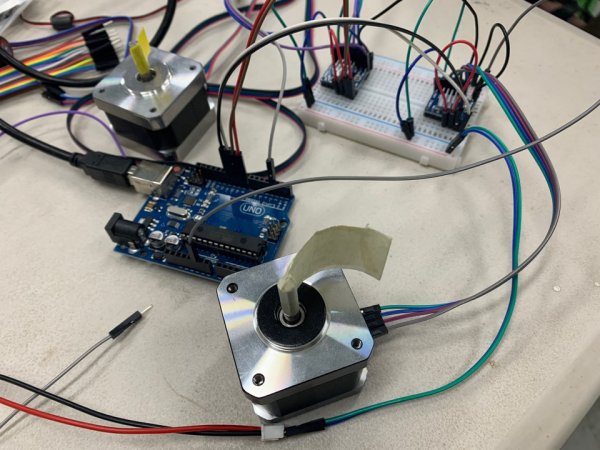
I checked if the code is uploaded first through the Arduino board.
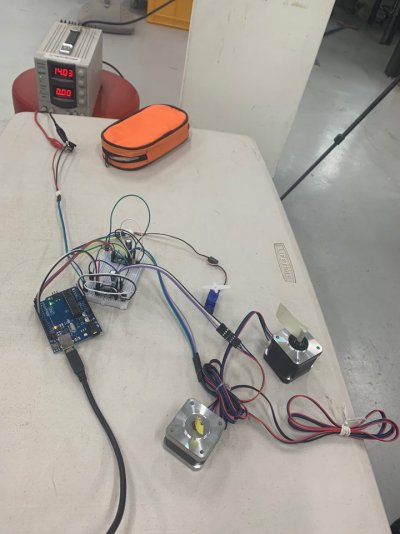
The power supply was delivered through the power supply, and the power required for the minimum motor was tested by giving at least 9V (maximum 24V) 14V. Always shut off and run the power when fixing the pin!
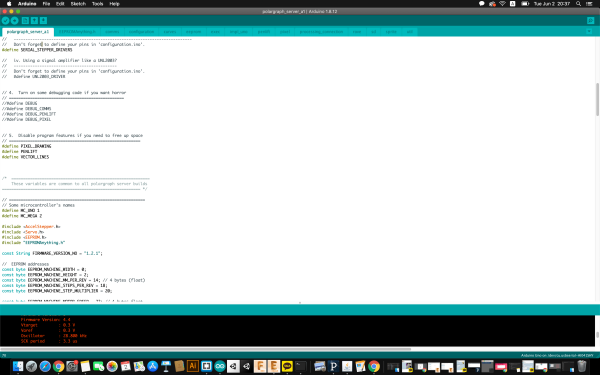
And I uploaded the code and proceeded with the test. The example code can be found on our group page. First is the test code in which the motor rotates.
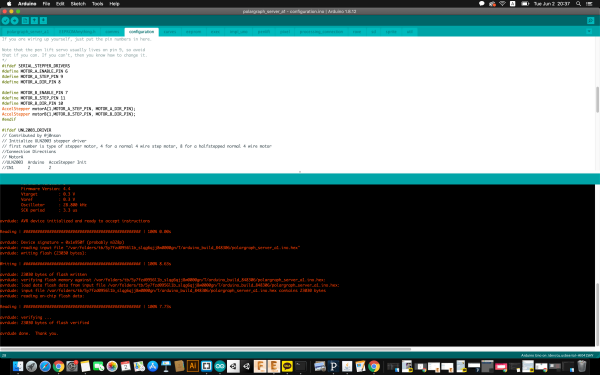
he pinout numbers were set differently, so we kept trying, resetting them to fit the pin numbers we set.
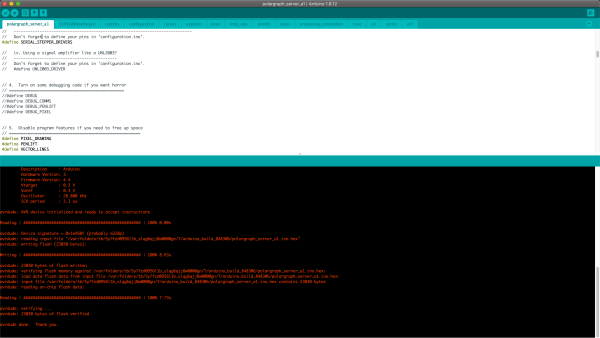
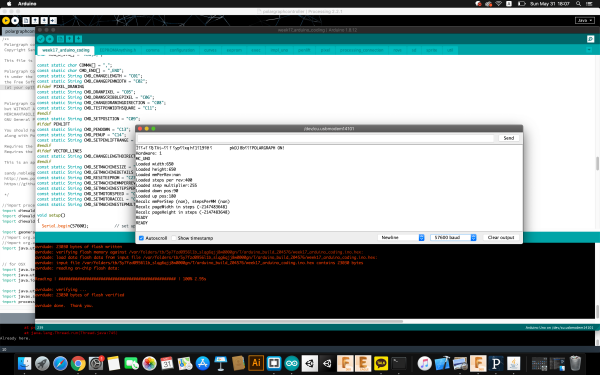
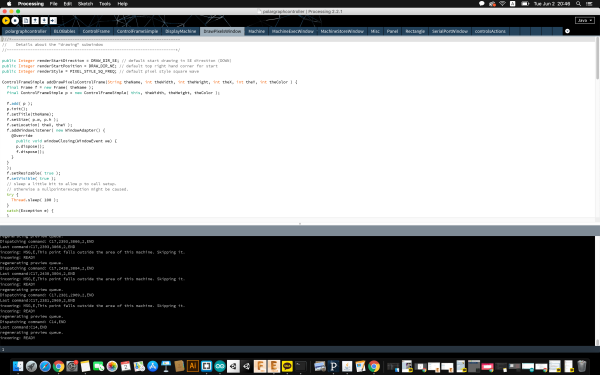
The following used a processing program for the interface.
In order to understand the flow/structure of the Arduino code, we briefly read through code along with the grey text that describes what each part does and is for. There was a part where we could tell the code about which motor driver we are using. We changed that part to make the code work with our motor driver.
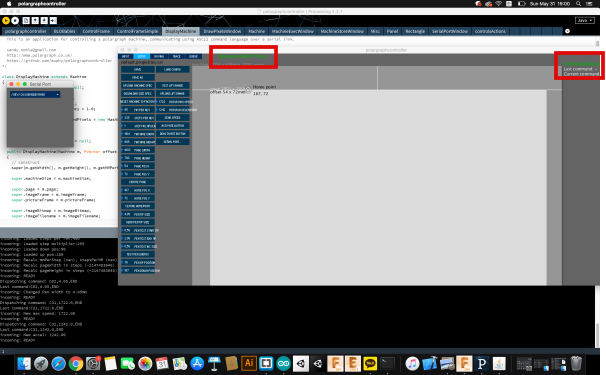
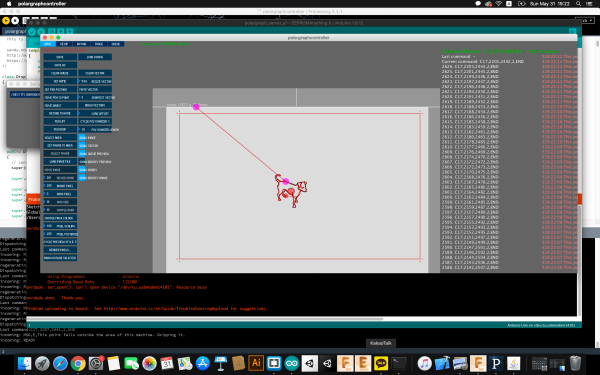
In order to understand the flow/structure of the Arduino code, we briefly read through code along with the grey text that describes what each part does and is for. There was a part where we could tell the code about which motor driver we are using. We changed that part to make the code work with our motor driver.
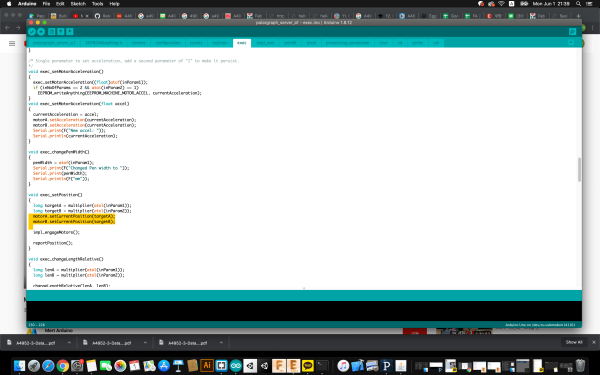
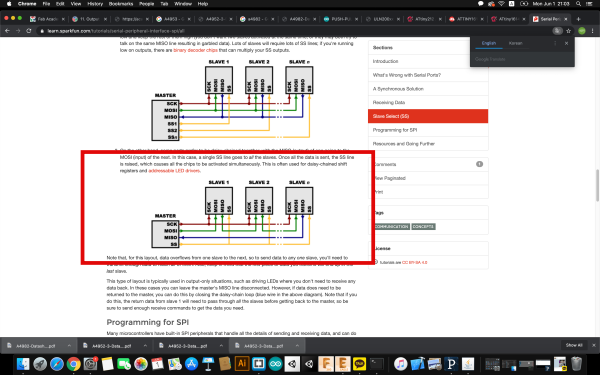
The motor moved. Problem solved. #Interface 11.To move forward and work on our interface, we downloaded the Polar Graph Controller. (Why this?) We looked at whether or not this interface is compatible with the motor drive we were using. After checking they are compatible, we moved on to the next step.
Arduino Code
This is the Arduino code we used. Here we could create a link with the Processing software.
/** * Polargraph Server for Arduino UNO and MEGA compatible boards. * Written by Sandy Noble * Released under GNU License version 3. * http://www.polargraph.co.uk * https://github.com/euphy/polargraph_server_a1 CONFIGURATION!! Read this! Really. ================================== Kung fu is like a game of chess. You must think first! Before you move. This is a unified codebase for a few different versions of Polargraph Server. You can control how it is compiled by changing the #define lines below. There are five config sections: 1. Specify what kind of controller board you are using 2. Add some libraries if you have a MEGA 3. Specify what kind of motor driver you are using: i. Adafruit Motorshield v1 ii. Adafruit Motorshield v2 iii. Discrete stepper drivers (eg EasyDriver, stepstick, Pololu gear).* iv. Signal amplifier like a UNL2003* 4. Turn on some debugging code 5. Disable program features if you need to free up space * For motor drivers iii and iv, you will need to change the values in configuration.ino to set the exact pins the drivers are wired up to. */ // 1. Specify what kind of controller board you are using // ====================================================== // UNO or MEGA. Uncomment the line for the kind of board you have. #ifndef MICROCONTROLLER #define MICROCONTROLLER MC_UNO //#define MICROCONTROLLER MC_MEGA #endif // 2. Add some libraries if you have a MEGA // ======================================== // Uncomment the SPI and SD lines below if you have a MEGA. // http://forum.arduino.cc/index.php?topic=173584.0 //#include//#include // 3. Specify what kind of motor driver you are using // ================================================== // Only ONE set of lines below should be uncommented. // i. Adafruit Motorshield v1. The original, and still the best. // ------------------------------------------------------------- //#define ADAFRUIT_MOTORSHIELD_V1 //#include // ii. Adafruit Motorshield v2. It's all squealy. // ---------------------------------------------- //#define ADAFRUIT_MOTORSHIELD_V2 //#include //#include //#include "utility/Adafruit_PWMServoDriver.h" // iii. Using discrete stepper drivers? (eg EasyDriver, stepstick, Pololu gear) // ---------------------------------------------------------------------------- // Don't forget to define your pins in 'configuration.ino'. #define SERIAL_STEPPER_DRIVERS // iv. Using a signal amplifier like a UNL2003? // -------------------------------------------- // Don't forget to define your pins in 'configuration.ino'. // #define UNL2003_DRIVER // 4. Turn on some debugging code if you want horror // ================================================= //#define DEBUG //#define DEBUG_COMMS //#define DEBUG_PENLIFT //#define DEBUG_PIXEL // 5. Disable program features if you need to free up space // ======================================================== #define PIXEL_DRAWING #define PENLIFT #define VECTOR_LINES /* =========================================================== These variables are common to all polargraph server builds =========================================================== */ // ========================================================== // Some microcontroller's names #define MC_UNO 1 #define MC_MEGA 2 #include #include #include #include "EEPROMAnything.h" const String FIRMWARE_VERSION_NO = "1.2.1"; // EEPROM addresses const byte EEPROM_MACHINE_WIDTH = 0; const byte EEPROM_MACHINE_HEIGHT = 2; const byte EEPROM_MACHINE_MM_PER_REV = 14; // 4 bytes (float) const byte EEPROM_MACHINE_STEPS_PER_REV = 18; const byte EEPROM_MACHINE_STEP_MULTIPLIER = 20; const byte EEPROM_MACHINE_MOTOR_SPEED = 22; // 4 bytes float const byte EEPROM_MACHINE_MOTOR_ACCEL = 26; // 4 bytes float const byte EEPROM_MACHINE_PEN_WIDTH = 30; // 4 bytes float const byte EEPROM_MACHINE_HOME_A = 34; // 4 bytes const byte EEPROM_MACHINE_HOME_B = 38; // 4 bytes const byte EEPROM_PENLIFT_DOWN = 42; // 2 bytes const byte EEPROM_PENLIFT_UP = 44; // 2 bytes // Pen raising servo Servo penHeight; const int DEFAULT_DOWN_POSITION = 90; const int DEFAULT_UP_POSITION = 180; static int upPosition = DEFAULT_UP_POSITION; // defaults static int downPosition = DEFAULT_DOWN_POSITION; static int penLiftSpeed = 3; // ms between steps of moving motor const byte PEN_HEIGHT_SERVO_PIN = 9; //UNL2003 driver uses pin 9 boolean isPenUp = false; // Machine specification defaults const int DEFAULT_MACHINE_WIDTH = 650; const int DEFAULT_MACHINE_HEIGHT = 650; const int DEFAULT_MM_PER_REV = 95; const int DEFAULT_STEPS_PER_REV = 400; const int DEFAULT_STEP_MULTIPLIER = 1; // working machine specification static int motorStepsPerRev = DEFAULT_STEPS_PER_REV; static float mmPerRev = DEFAULT_MM_PER_REV; static byte stepMultiplier = DEFAULT_STEP_MULTIPLIER; static int machineWidth = DEFAULT_MACHINE_WIDTH; static int machineHeight = DEFAULT_MACHINE_HEIGHT; static float currentMaxSpeed = 800.0; static float currentAcceleration = 400.0; static boolean usingAcceleration = true; int startLengthMM = 800; float mmPerStep = 0.0F; float stepsPerMM = 0.0F; long pageWidth = machineWidth * stepsPerMM; long pageHeight = machineHeight * stepsPerMM; long maxLength = 0; //static char rowAxis = 'A'; const int INLENGTH = 50; const char INTERMINATOR = 10; const char SEMICOLON = ';'; float penWidth = 0.8F; // line width in mm boolean reportingPosition = true; boolean acceleration = true; extern AccelStepper motorA; extern AccelStepper motorB; boolean currentlyRunning = true; static char inCmd[10]; static char inParam1[14]; static char inParam2[14]; static char inParam3[14]; static char inParam4[14]; static byte inNoOfParams; char lastCommand[INLENGTH+1]; boolean commandConfirmed = false; int rebroadcastReadyInterval = 5000; long lastOperationTime = 0L; long motorIdleTimeBeforePowerDown = 600000L; boolean automaticPowerDown = true; boolean powerIsOn = false; long lastInteractionTime = 0L; #ifdef PIXEL_DRAWING static boolean lastWaveWasTop = true; // Drawing direction const static byte DIR_NE = 1; const static byte DIR_SE = 2; const static byte DIR_SW = 3; const static byte DIR_NW = 4; static int globalDrawDirection = DIR_NW; const static byte DIR_MODE_AUTO = 1; const static byte DIR_MODE_PRESET = 2; static byte globalDrawDirectionMode = DIR_MODE_AUTO; #endif #if MICROCONTROLLER == MC_MEGA #define READY_STR "READY_100" #else #define READY_STR "READY" #endif #define RESEND_STR "RESEND" #define DRAWING_STR "DRAWING" #define OUT_CMD_SYNC_STR "SYNC," char MSG_E_STR[] = "MSG,E,"; char MSG_I_STR[] = "MSG,I,"; char MSG_D_STR[] = "MSG,D,"; const static char COMMA[] = ","; const static char CMD_END[] = ",END"; const static String CMD_CHANGELENGTH = "C01"; const static String CMD_CHANGEPENWIDTH = "C02"; #ifdef PIXEL_DRAWING const static String CMD_DRAWPIXEL = "C05"; const static String CMD_DRAWSCRIBBLEPIXEL = "C06"; const static String CMD_CHANGEDRAWINGDIRECTION = "C08"; const static String CMD_TESTPENWIDTHSQUARE = "C11"; #endif const static String CMD_SETPOSITION = "C09"; #ifdef PENLIFT const static String CMD_PENDOWN = "C13"; const static String CMD_PENUP = "C14"; const static String CMD_SETPENLIFTRANGE = "C45"; #endif #ifdef VECTOR_LINES const static String CMD_CHANGELENGTHDIRECT = "C17"; #endif const static String CMD_SETMACHINESIZE = "C24"; const static String CMD_GETMACHINEDETAILS = "C26"; const static String CMD_RESETEEPROM = "C27"; const static String CMD_SETMACHINEMMPERREV = "C29"; const static String CMD_SETMACHINESTEPSPERREV = "C30"; const static String CMD_SETMOTORSPEED = "C31"; const static String CMD_SETMOTORACCEL = "C32"; const static String CMD_SETMACHINESTEPMULTIPLIER = "C37"; void setup() { Serial.begin(57600); // set up Serial library at 57600 bps Serial.println("POLARGRAPH ON!"); Serial.print("Hardware: "); Serial.println(MICROCONTROLLER); #if MICROCONTROLLER == MC_MEGA Serial.println("MC_MEGA"); #elif MICROCONTROLLER == MC_UNO Serial.println("MC_UNO"); #else Serial.println("No MC"); #endif configuration_motorSetup(); eeprom_loadMachineSpecFromEeprom(); configuration_setup(); motorA.setMaxSpeed(currentMaxSpeed); motorA.setAcceleration(currentAcceleration); motorB.setMaxSpeed(currentMaxSpeed); motorB.setAcceleration(currentAcceleration); float startLength = ((float) startLengthMM / (float) mmPerRev) * (float) motorStepsPerRev; motorA.setCurrentPosition(startLength); motorB.setCurrentPosition(startLength); for (int i = 0; i