Interface & application programming đ
đ Date / Time:
Hey there friends, I hope everything is going well. In this humble web page I'm going to document my journey through this task which topic is brand new to me.
The task was to create a Graphic User Interface (GUI) and use it to control one of my fab academy circuits.
Approach:
Since it is a brand new topic to me and that I had very little time, I chose to go simple this time (Totally unlike myself) :D
But also since I planned not to finish fab academy without learning a bit of python and applying what I learnt in at least one code. It was time to fulfill that desire.
Part I: Python
Learning Sources:
When I searched YouTube on how to create a GUI with python al lot of videos had the word "Tkinter" in there titles yet I did not know what this was. Untill I landed onto this tutorial playlist from a channel called "The new Boston" that was so useful to me in order to get started.
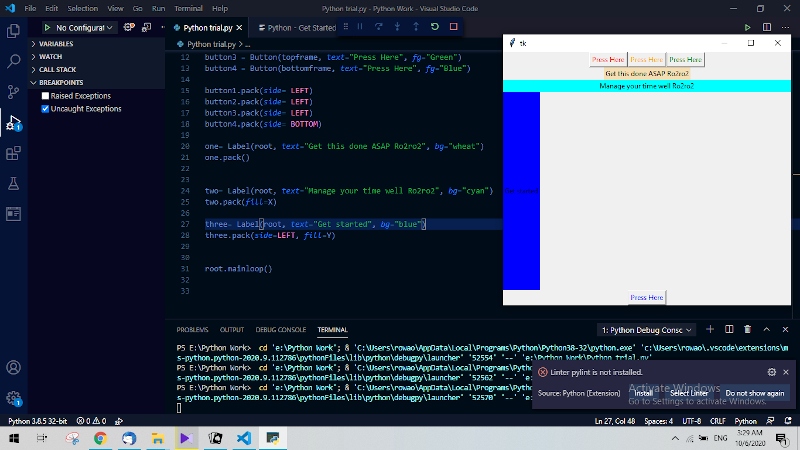
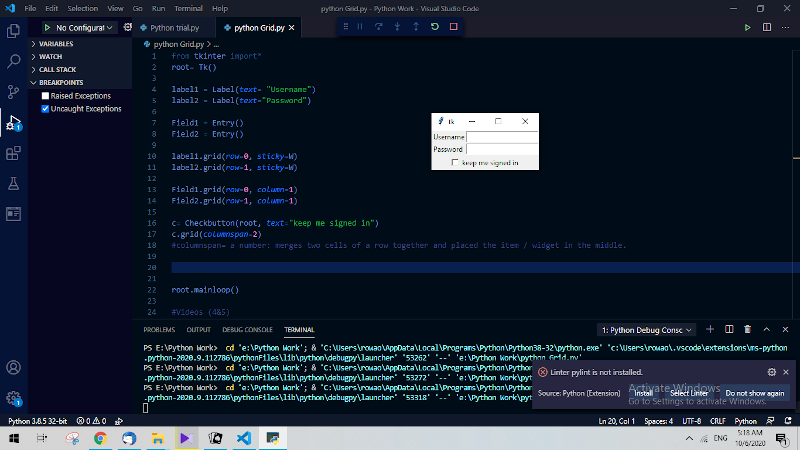
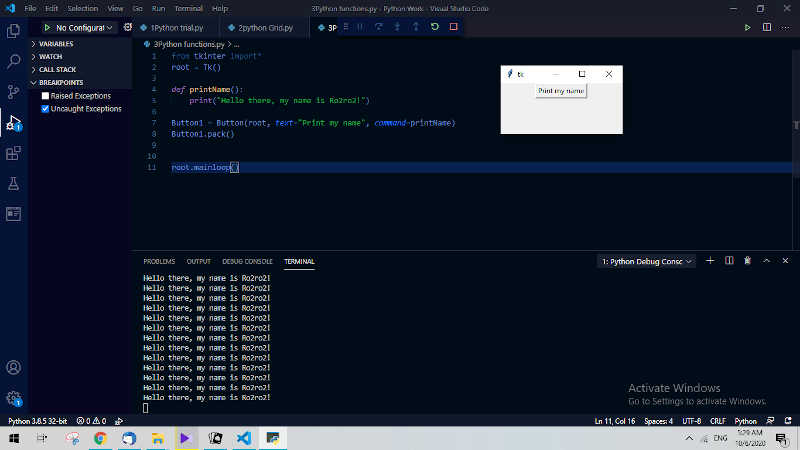
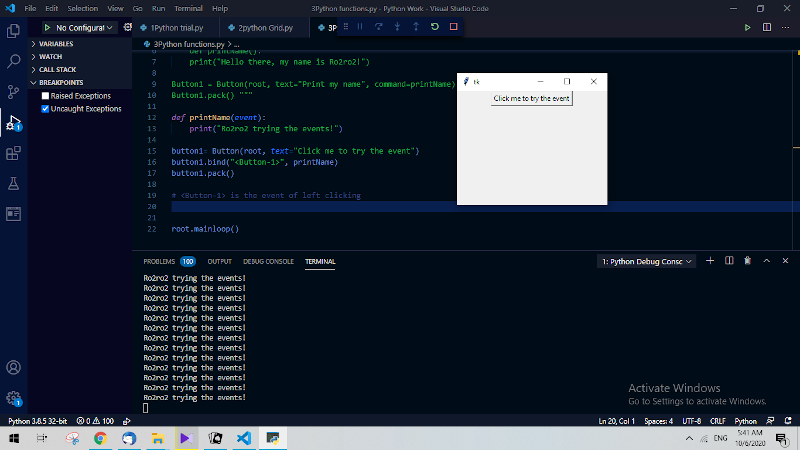
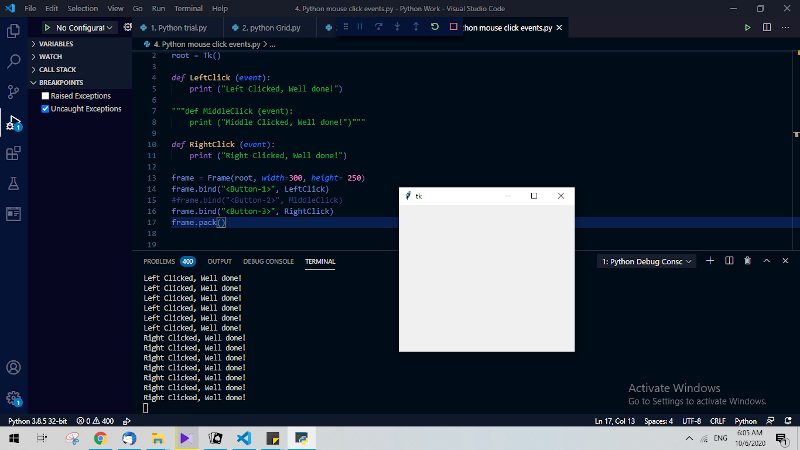
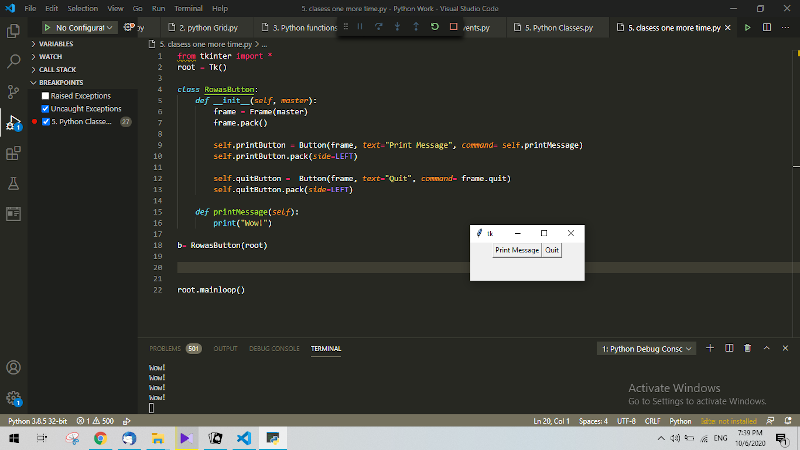
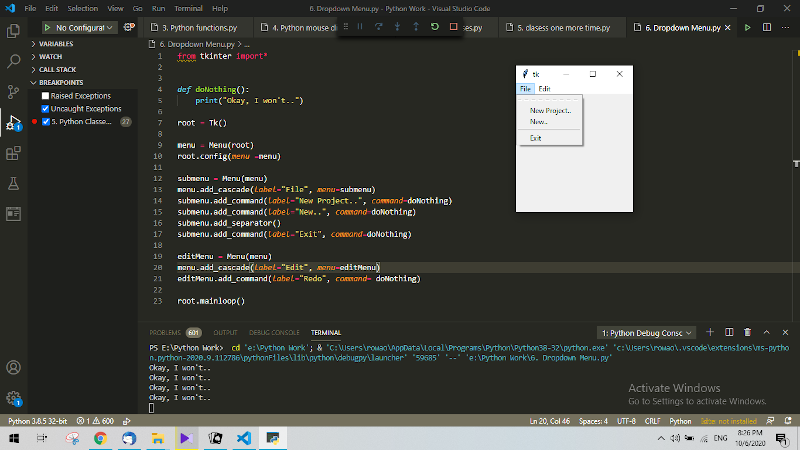
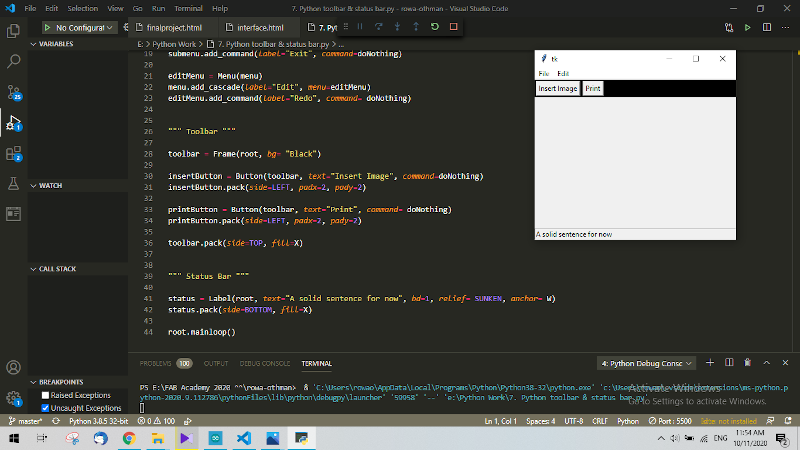
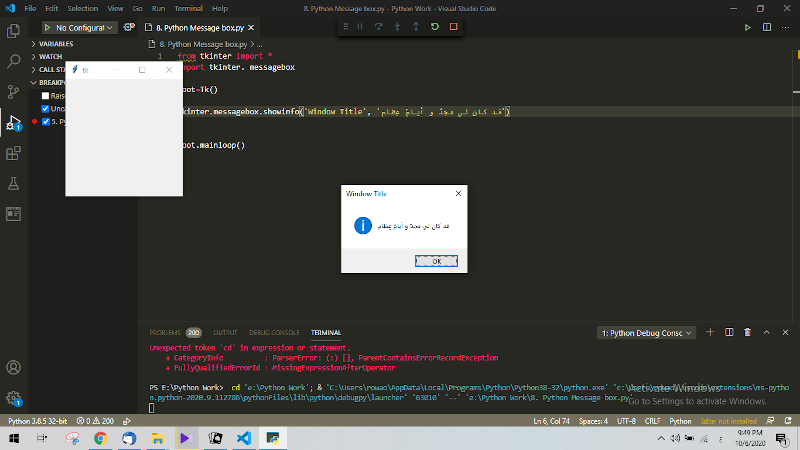
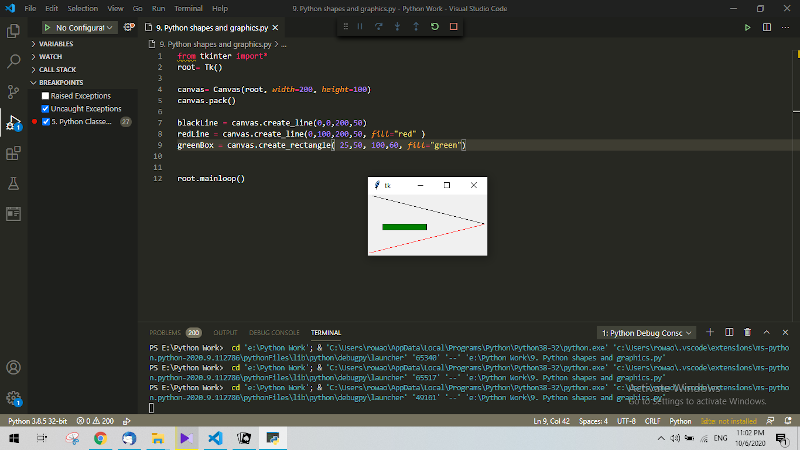
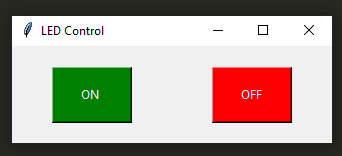
Python Code:
from tkinter import* import serial arduinoData = serial.Serial('COM5', 9600) def ledOn (): arduinoData.write(b'1') def ledOff (): arduinoData.write(b'0') root= Tk() root.title("LED Control") frame = Frame(root) frame.pack() buttonOn= Button (root, text="ON ", bg="Green", fg="White", width="10", height="3", command=ledOn) buttonOn.pack(side=LEFT, padx=40, pady=20) buttonOff= Button (root, text="OFF", bg="Red", fg="White",width="10", height="3", command=ledOff) buttonOff.pack(side=LEFT, padx=40, pady=20) root.mainloop()
Now that I've learnt some acceptable introduction to the GUI using Tkinter - Python, it is time to figure out how to link a python code with an arduino code to control an LED.
Part II: Arduino
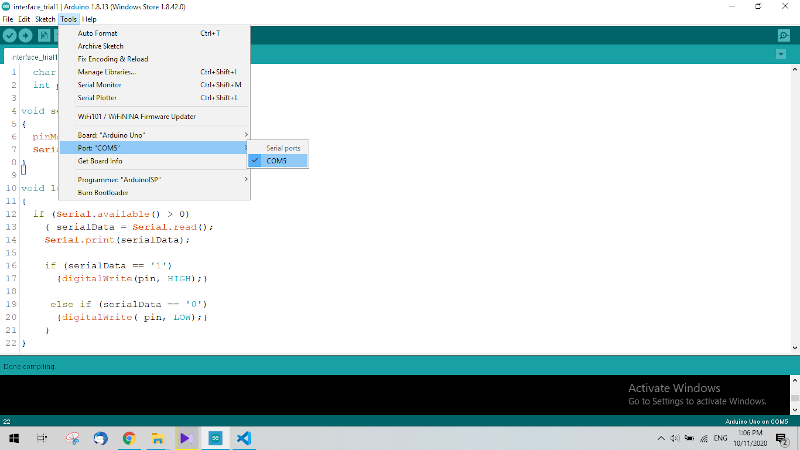
Arduino Code:
char serialData; int pin = 13; void setup() { pinMode(pin, OUTPUT); Serial.begin(9600); } void loop() { if (Serial.available() > 0) { serialData = Serial.read(); Serial.print(serialData); if (serialData == '1') {digitalWrite(pin, HIGH);} else if (serialData == '0') {digitalWrite( pin, LOW);} } }
Part III: Circuit Intrface
Using the output circuit aka "Awesome PCB 03" I applied my LED control interface.
Steps:
1- I uploaded the code below.
2- I changed the common port to the current "COM7" in the python code.
3- I connected RX of Arduino to TX of the circuit and vice versa.
#include < SoftwareSerial.h> #define RX 0 #define TX 1 SoftwareSerial MySerial(RX, TX); char serialData; int pin = 8; void setup() { pinMode(pin, OUTPUT); MySerial.begin(9600); } void loop() { if (MySerial.available() > 0) { serialData = MySerial.read(); MySerial.print(serialData); if (serialData == '1') {digitalWrite(pin, HIGH);} else if (serialData == '0') {digitalWrite( pin, LOW);} } }
Downloadables: đž
- Python file.pyHere
- Arduino interface file.inoHere
- Circuit interface file.inoHere
___________________________________________
ÂŠī¸ Row'a M. M. Othman - Fab Academy 2020