Project
Raspberry Pi Tips
Install OpenCV
Raspberry Pi 3 Model B V1.2
$ cat /etc/debian_version 9.11
1. Install library dependencies
$ sudo apt-get install cmake git libgtk2.0-dev pkg-config libavcodec-dev libavformat-dev libswscale-dev python-dev python-numpy libtbb2 libtbb-dev libjpeg-dev libpng-dev libtiff-dev libjasper-dev libdc1394-22-dev
2. Install OpenCV and OpenCV-contrib-python over pip
OpenCV-contrib-python is external module of OpenCV.
$ sudo pip3 install opencv-python Looking in indexes: https://pypi.org/simple, https://www.piwheels.org/simple Requirement already satisfied: opencv-python in /usr/local/lib/python3.5/dist-packages (3.4.4.19) Requirement already satisfied: numpy>=1.12.1 in /usr/lib/python3/dist-packages (from opencv-python) (1.12.1) $ sudo pip3 install opencv-contrib-python Looking in indexes: https://pypi.org/simple, https://www.piwheels.org/simple Collecting opencv-contrib-python Downloading https://www.piwheels.org/simple/opencv-contrib-python/opencv_contrib_python-4.1.1.26-cp35-cp35m-linux_armv7l.whl (16.0 MB) |████████████████████████████████| 16.0 MB 48 kB/s Requirement already satisfied: numpy>=1.12.1 in /usr/lib/python3/dist-packages (from opencv-contrib-python) (1.12.1) Installing collected packages: opencv-contrib-python Successfully installed opencv-contrib-python-4.1.1.26
3. Install libraries for executing OpenCV
$ sudo apt install libhdf5-103 libqtgui4 libatlas3-base libjasper1 libqt4-test パッケージリストを読み込んでいます... 完了 依存関係ツリーを作成しています 状態情報を読み取っています... 完了 E: パッケージ libhdf5-103 が見つかりません
HDF(Hierarchical Data Format) is a set of file formats (HDF4, HDF5) designed to store and organize large amounts of data in Hierarchical model. I found a thread in stackoverflow forum about install hdf5 for python3. It's the case for ubuntu, but I tried to go with the procedure as follows. This works fine.
$ sudo apt-get install libhdf5-serial-dev netcdf-bin libnetcdf-dev
4. A simple python code for capturing image by Camera module
cv2_1.pyimport cv2 cap = cv2.VideoCapture(0) while True: # capture for each 1 frame ret, frame = cap.read() # if cannot cappture, close the window if not ret: break # output to the window cv2.imshow("Frame", frame) key = cv2.waitKey(1) # if interrupted, stop the process if key == 27: break cap.release() cv2.destroyAllWindows()
Camera worked and streamed video to the window frame
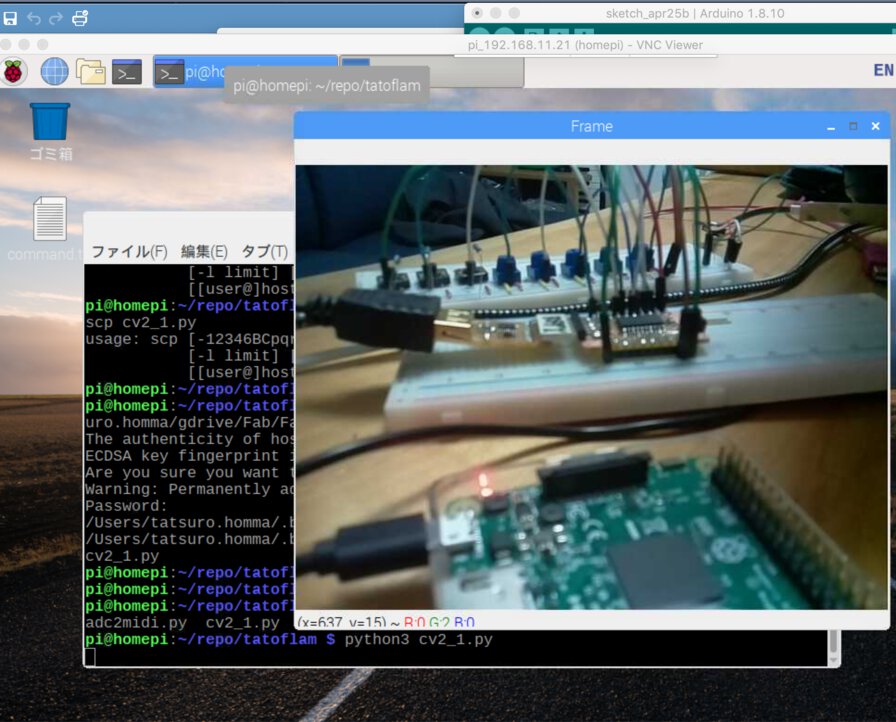
Refs: