Group Assignment 4 - Embedded Programming
What is Embedded Programming?
One kind of programming language used to create system code based on a microcontroller or microprocessor is called embedded programming. There are certain hardware requirements for this programming language. A common form of Internet of Things and electronic consumer applications are embedded systems, which are utilized inside industrial machinery, bicycle charging systems, and residential appliances.
Where is Embedded Programming Used?
Embedded programming is widely used across various industries.
Industrial Automation: Used for production monitoring and machine control.
Consumer Electronics: Found in devices like digital cameras, washing machines, and smart TVs.
Internet of Things (IoT): Powers smart home appliances and connected devices.
Automotive Systems: Plays a crucial role in airbags, ABS brakes, and engine control.
Medical Equipment: Used in devices such as ventilators, insulin pumps, and heart rate monitors.
About Microcontroller
A circuit called a microcontroller is made to regulate particular operations in an embedded system. On a single chip, it combines a CPU, memory (RAM, ROM, and Flash), and input/output peripherals. Because of their low power consumption and real-time processing capabilities, microcontrollers are widely utilized in consumer electronics, automation, robotics, and the Internet of Things.
Microcontroller Comparison
Microcontroller |
Architecture |
Bit Size |
Clock Speed |
Memory (RAM/Flash) |
Power Consumption |
Key Features |
Primary Use |
AVR |
RISC |
8-bit, 32-bit |
Up to 20 MHz |
2KB RAM / 32KB Flash |
Low |
Simple, cost-effective, widely used in Arduino |
Basic embedded systems, DIY projects, robotics |
ARM |
RISC |
32-bit, 64-bit |
Up to 2 GHz |
Up to 2GB RAM / 64GB Flash |
Low to moderate |
High performance, energy-efficient, scalable |
Smartphones, tablets, automotive, industrial control |
ESP32 |
Xtensa Dual-Core |
32-bit |
Up to 240 MHz |
520KB RAM / 16MB Flash |
Low to moderate |
Wi-Fi, Bluetooth, dual-core processing, IoT-ready |
IoT, smart devices, AIoT, wireless communication |
STM32 |
ARM Cortex-M |
32-bit |
Up to 550 MHz |
Up to 1MB RAM / 2MB Flash |
Low to moderate |
High-performance, low power, widely used in industrial applications |
Industrial automation, robotics, medical devices |
1. AVR Microcontrollers
AVR microcontrollers are commonly used in small embedded systems and are based on the RISC (Reduced Instruction Set Computer) architecture.
They are widely popular among beginners and hobbyists, often found in Arduino boards.
Known for their low power consumption, simplicity, and ease of programming, AVR chips are a preferred choice for basic embedded applications.
2. ARM Microcontrollers
ARM microcontrollers are used in a wide range of high-performance applications.
They are known for their scalability and power efficiency, making them ideal for industrial automation, automotive control, and mobile devices.
The ARM Cortex-M family is widely utilized in various embedded systems.
3. ESP Microcontrollers
The ESP8266 and ESP32 are popular microcontrollers designed for Internet of Things (IoT) applications.
These microcontrollers are ideal for cloud-based applications, wireless communications, and smart home automation due to their built-in Wi-Fi and Bluetooth capabilities.
Among IoT projects, the ESP32 is widely used for its advanced features and versatility.
4. STM32 Microcontroller
STMicroelectronics' STM32 microcontrollers are based on the ARM Cortex-M core.
They are widely used in automotive, medical, and industrial automation applications.
These microcontrollers offer real-time processing, multiple peripherals, and high computational power, making them suitable for a variety of advanced embedded systems.
Reference by Search Engine
Toolchains and Development Workflows
The key instruments and procedures required for embedded system development are the subject of research on toolchains and development workflows. A compiler, assembler, linker, debugger, and integrated development environment (IDE) comprise a toolchain that facilitates the efficient writing, compilation, and debugging of code. Different toolchains are used by different microcontrollers, including AVR, ARM, ESP32, and STM32, to guarantee the seamless creation and operation of embedded applications. A clear workflow increases productivity, lowers errors, and boosts system performance as a whole.
Toolchain Components
- Compiler: Transforms high-level code (C, C++) into machine code. Examples include GCC, Clang, IAR, and Keil.
- Assembler: Converts assembly language into binary instructions. Common assemblers include GNU Assembler and NASM.
- Linker: Combines compiled code and libraries to construct an executable. Examples include GNU LD and Keil Linker.
- Debugger: Enables step-by-step execution for troubleshooting. Popular debuggers include GDB, OpenOCD, and J-Link Debugger.
- Integrated Development Environment (IDE): Provides a complete development environment. Examples include VS Code, Eclipse, Keil, STM32CubeIDE, and Arduino IDE.
Development Workflow
- Requirement Analysis: Select the appropriate hardware and define system objectives.
- Code Development: Write embedded code using C, C++, or Assembly.
- Compilation & Linking: Utilize the toolchain to convert source code into machine code.
- Flashing to Target: Use a programmer or debugger to upload the compiled application onto the microcontroller.
- Testing & Debugging: Employ tools like Serial Monitor, JTAG, or SWD for real-time debugging.
- Optimization: Enhance power efficiency, minimize memory usage, and improve overall performance.
- Maintenance & Deployment: Finalize and install the firmware on operational devices.
Example Toolchain for Microcontrollers
Microcontroller |
Compiler |
IDE |
Debugger |
Programmer |
AVR (ATmega328) |
AVR-GCC |
Atmel Studio, Arduino IDE |
AVR GDB |
USBasp, AVR ISP |
ARM (STM32) |
ARM GCC |
STM32CubeIDE, Keil |
OpenOCD, GDB |
ST-Link |
ESP32 |
Xtensa GCC |
VS Code, ESP-IDF |
GDB, OpenOCD |
ESPTool |
Which Tools Were Easier to Use?
Tool |
Ease of Use |
Arduino IDE |
Simple interface, good for beginners, supports Arduino boards and basic programming. |
PlatformIO |
More advanced, offers integration with different microcontrollers and libraries, easier debugging and testing. |
STM32CubeIDE |
Good for STM32 boards, provides visual configuration tools for peripherals and middleware, but may be complex for beginners. |
Arduino UNO & Arduino Nano
We are using the Arduino UNO and Arduino Nano for microcontroller programming. These boards are easy to use and beginner-friendly. We are testing them for LED control, motor driving and sensor interfacing using the Arduino IDE. Their simplicity makes them ideal for learning digital and analog I/O, PWM and serial communication.
Arduino UNO
Arduino UNO
LED Blink
void setup() {
pinMode(13, OUTPUT);
}
void loop() {
digitalWrite(13, HIGH);
delay(1000);
digitalWrite(13, LOW);
delay(1000);
}
Pin Configuration: pinMode(13, OUTPUT); sets pin 13 as an output.
LED ON: digitalWrite(13, HIGH); turns the LED ON.
LED OFF: digitalWrite(13, LOW); turns the LED OFF.
Time Delay: delay(1000); creates a one-second pause between ON and OFF states.
Loop Execution: The loop() function repeats indefinitely, making the LED blink continuously.
Basic Arduino Concept: Demonstrates digital output control and the fundamental structure of an Arduino program.
Arduino Nano
LED Blink
void setup() {
pinMode(13, OUTPUT);
}
void loop() {
digitalWrite(13, HIGH);
delay(1000);
digitalWrite(13, LOW);
delay(1000);
}
Pin Configuration: pinMode(13, OUTPUT); sets pin 13 as an output.
LED ON: digitalWrite(13, HIGH); turns the LED ON.
LED OFF: digitalWrite(13, LOW); turns the LED OFF.
Time Delay: delay(1000); creates a one-second pause between ON and OFF states.
Loop Execution: The loop() function repeats indefinitely, making the LED blink continuously.
Basic Arduino Concept: Demonstrates digital output control and the fundamental structure of an Arduino program.
ESP32
We explored a small basic blinking code in ESP32 in which we got to know how to determine the pin out, how to write the code and what libraries will be added in it and also using C++..
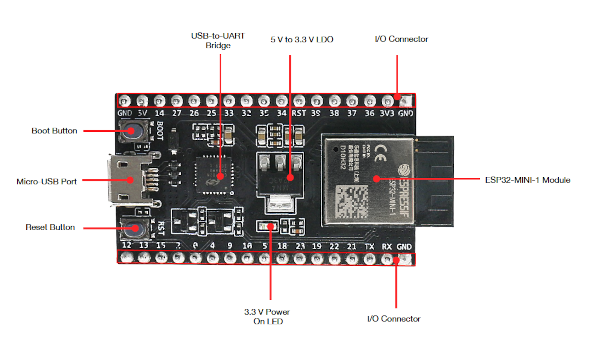 |
LED Blink (GPIO 2)
void setup() {
pinMode(2, OUTPUT);
}
void loop() {
digitalWrite(2, HIGH);
delay(1000);
digitalWrite(2, LOW);
delay(1000);
}
|
Pin Mode(2, OUTPUT); configures pin 2 as an output.
LED ON: pin 2 is turned on by digitalWrite(2, HIGH).
Delay: delay(1000); produces a pause of one second.
LED OFF: pin 2 is turned off by digitalWrite(2, LOW).
Loop Execution: The loop() method continuously repeats the procedure.
Raspberry Pi
We got information about Raspberry, but we found out about it through the Internet: what is used in it, what type of language is used, we saw a small block of code from the Internet and how it works.
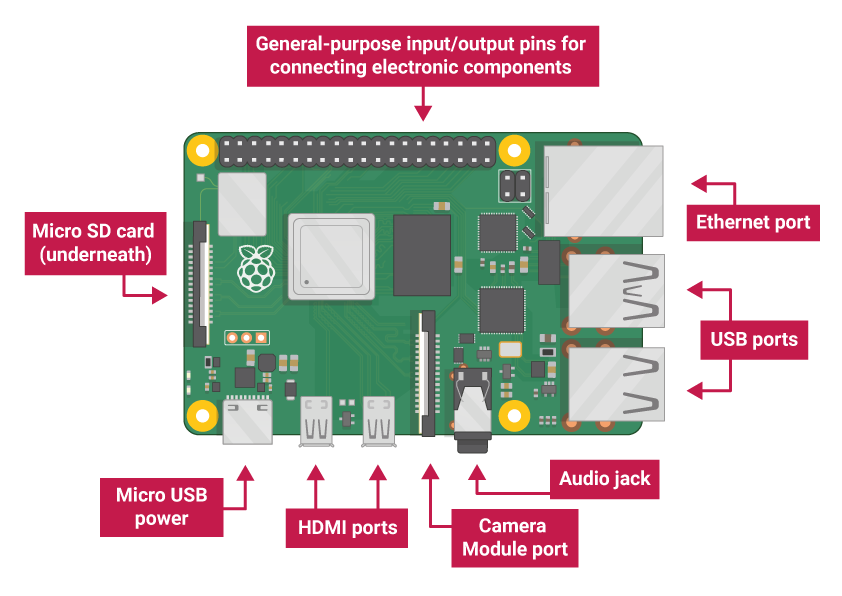 |
LED Blink (GPIO 17)
import RPi.GPIO as GPIO
import time
GPIO.setmode(GPIO.BCM)
GPIO.setup(17, GPIO.OUT)
while True:
GPIO.output(17, GPIO.HIGH)
time.sleep(1)
GPIO.output(17, GPIO.LOW)
time.sleep(1)
|
RPi is the library import.Time for delays and GPIO for GPIO control.
GPIO is the pin mode.The Broadcom (BCM) pin numbering is set by setmode(GPIO.BCM).
GPIO is the pin setup.GPIO 17 is configured as an output by using setup(17, GPIO.OUT).
GPIO is alternated with LED blinking.GPIO.LOW (OFF) and HIGH (ON) with time.sleep (1).
Infinite Loop: while True: keeps the LED flickering by running continuously.
Micro:bit
We learned about the Micro:bit With its integrated Bluetooth, sensors, and LEDs, it's a great tool for interactive projects and rapid prototyping. Python and Microsoft MakeCode programming can be used to construct small game applications.
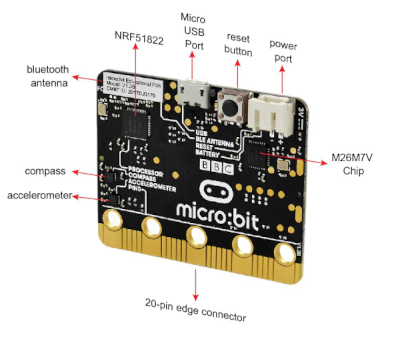 |
LED Blink
from microbit import *
while True:
display.show(Image.HEART)
sleep(1000)
display.clear()
sleep(1000)
|
Library Import: imports all required Micro:bit routines from microbit import.
While True guarantees the code executes constantly, Infinite Loop does the opposite.
Show Heart: show.The LED matrix displays a heart icon when show(Image.HEART) is used.
Sleep(1000) stops for a single second.
Clear Display: show.Before repeating, clear() disables the LED matrix.
Benefits of these boards at Fab Academy
We are learning how to select the appropriate board for various applications by working on this assignment. The Raspberry Pi works well for computing-based applications, the ESP32 is best for the Internet of Things, the Arduino is excellent for basic automation, and the Micro:bit is perfect for educational projects. Knowing these boards enables us to create more inventive and effective embedded systems and digital manufacturing solutions.
Comparison sheet
Board |
Microcontroller / Processor |
Operating Voltage |
Digital I/O Pins |
Analog Input Pins |
Flash Memory |
Connectivity |
Special Features |
Arduino UNO |
ATmega328P |
5V |
14 (6 PWM) |
6 |
32 KB |
USB |
Beginner-friendly, widely used |
Arduino Nano |
ATmega328P |
5V |
14 (6 PWM) |
8 |
32 KB |
USB (Mini-USB) |
Compact, breadboard-friendly |
ESP32 |
Tensilica Xtensa LX6 (Dual-core) |
3.3V |
36 |
18 |
4 MB |
WiFi, Bluetooth |
Great for IoT applications |
Raspberry Pi |
Broadcom BCM2711 (Quad-core Cortex-A72) |
5V (USB-C) |
40 GPIO |
N/A |
MicroSD Storage |
WiFi, Bluetooth, Ethernet |
Full Linux OS support |
BBC Micro:bit |
Nordic nRF52833 (ARM Cortex-M4) |
3.3V |
25 |
3 |
512 KB |
Bluetooth, USB |
LED matrix, sensors, compact |
I Learn all Board bySearch Engine
Servo Motor with Bluetooth
For our group assignment, we chose to control a servo motor via Bluetooth.
This project allows us to wirelessly rotate the servo motor using a smartphone app.
Below are the steps we followed to achieve this.
Components Required
- Arduino Uno
- HC-05 Bluetooth Module
- Servo Motor
- Jumper Wires
- Bredbord
- Sirial Bluetooth Controller App
Pinout and Connections
We connected the components as follows:
Component |
Arduino Pin |
Servo Motor Signal (Yellow Wire) |
D9 |
Servo Motor VCC (Red Wire) |
5V |
Servo Motor GND (Black Wire) |
GND |
HC-05 Bluetooth VCC |
5V |
HC-05 Bluetooth GND |
GND |
HC-05 TX |
D10 (Software Serial RX) |
HC-05 RX |
D11 (Software Serial TX) (Use a 1KΩ & 2KΩ voltage divider) |
Code
#include <SoftwareSerial.h>
#include <Servo.h>
SoftwareSerial BTSerial(10, 11); // RX, TX for Bluetooth
Servo myServo; // Servo object
char command; // Variable to store Bluetooth data
void setup() {
Serial.begin(9600); // Serial Monitor for debugging
BTSerial.begin(9600); // Bluetooth communication
myServo.attach(9); // Servo connected to Pin 9
myServo.write(90); // Start at neutral position (90°)
Serial.println("Bluetooth Ready! Send 'L' for Left, 'R' for Right.");
}
void loop() {
if (BTSerial.available()) {
command = BTSerial.read();
Serial.print("Received: ");
Serial.println(command);
// Control servo based on Bluetooth input
if (command == 'L') {
myServo.write(0); // Move Servo to 0° (Left)
Serial.println("Servo Moved Left");
}
else if (command == 'R') {
myServo.write(180); // Move Servo to 180° (Right)
Serial.println("Servo Moved Right");
}
}
}
Conclusion
This application uses an Arduino to enable Bluetooth-based servo control. Depending on the Bluetooth input ('L' or 'R'), the servo moves either left (0°) or right (180°). For debugging, the code offers real-time feedback on the Serial Monitor. By adding more commands, speed control, or automation sensors, this can be improved even more.
Buzzer with Bluetooth
We decided to integrate an alarm that can be controlled via Bluetooth to operate the servo motor.
While researching online, we discovered a device called a Buzzer, which produces sound.
This led us to consider using Bluetooth to turn the buzzer on and off.
We explored its functionality further, studied its code, and analyzed how it works.
After understanding the code, we realized that the buzzer could be controlled in a similar manner to a servo motor.
Components Required
- Arduino Uno
- HC-05 Bluetooth Module
- Buzzer
- Jumper Wires
- Sirial Bluetooth Controller App
Pinout and Connections
We connected the components as follows:
Component |
Arduino Pin |
Buzzer Positive (+) |
D9 |
Buzzer Negative (-) |
GND |
HC-05 Bluetooth VCC |
5V |
HC-05 Bluetooth GND |
GND |
HC-05 TX |
D10 (Software Serial RX) |
HC-05 RX |
D11 (Software Serial TX) (Use a 1KΩ & 2KΩ voltage divider) |
Arduino Code
The following Arduino code enables Bluetooth control for the buzzer. Sending -'L'- turns the buzzer -ON-, and sending -'R'- turns it -OFF-.
#include
SoftwareSerial BTSerial(10, 11); // RX, TX pins for Bluetooth communication
int buzzerPin = 9; // Pin connected to the buzzer
char command; // Variable to store the Bluetooth command
void setup() {
pinMode(buzzerPin, OUTPUT); // Set buzzer pin as output
Serial.begin(9600); // Start serial communication
BTSerial.begin(9600); // Start Bluetooth communication
}
void loop() {
if (BTSerial.available()) {
command = BTSerial.read(); // Read the Bluetooth command
if (command == 'L') {
digitalWrite(buzzerPin, HIGH); // Turn the buzzer on
Serial.println("Buzzer ON");
} else if (command == 'R') {
digitalWrite(buzzerPin, LOW); // Turn the buzzer off
Serial.println("Buzzer OFF");
}
}
}
Conclusion
This application makes it possible to use an Arduino to control a buzzer over Bluetooth. Using Bluetooth commands, the buzzer, which is attached to pin 9, can be turned on or off:
The buzzer is activated by sending 'L'.
The buzzer is turned off when 'R' is sent.
This straightforward but efficient system can be extended for a number of uses, including security systems, home automation, and remote alarms.
Conclusion:
Every microcontroller has a unique set of debugging tools, libraries, and functionalities. The Arduino UNO is a fantastic option for easy and novice projects, while the ESP32 shines in Internet of Things applications thanks to its extensive library and debugging features. Depending on the project's complexity, each microcontroller has unique benefits.