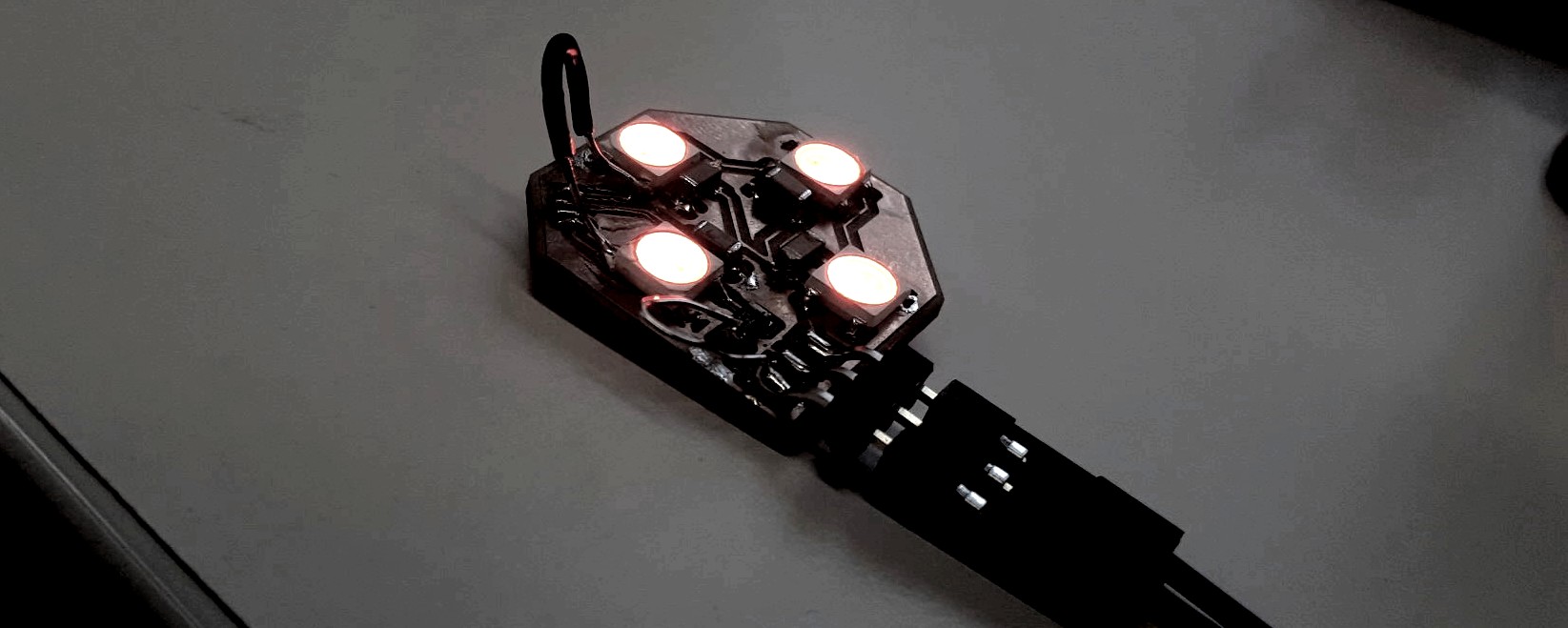
Week 10: Output Devices
Table of Contents
This week, we were introduced to different output devices (such as various types of motors, addressable LEDs, etc.), and how to work with them. In my personal assignment, I designed and built small addressable LED matrix based on WS2812B LEDs and set it up to be controlled by the gyroscope I worked with in the input-devices week.
This Week’s Tasks
…
Track of the Week
Measuring Power
For the power measurement task, I planned to first make the output device and then measuring its power. Therefore, I put this part to the end of the documentation. You can find the corresponding section here.
Attaching Snap Fasteners to PCBs



- soldering snap buttons works
- now create footprint for snap pins:
- want to have direction (i.e. it woudl not be possible to attach diode in the wrong way) so i wanted to have 6mm snaps for one side and 7mm for the other one.
- following this: https://www.pcbway.com/blog/PCB_Design_Tutorial/How_to_make_a_footprint_in_KiCad_.html
- creating a new symbol (for schematics)
- reference designator: j or p (bc we are plugging)
- i just chose j since it seemed to be most reasonable.
- https://en.wikipedia.org/wiki/Reference_designator
- number of units per package: how many are in one pocket of the roll (relevant for toolchanger). 1. for me not relevant.
- rest default
- renamed to “…_male”
- close editor. open footprint editor
- new footprint
- set name
- create pad (the most important part)
- the pad should be round of diameter of connector + via to connect to the other side.
- choose largest via possible (easier to handle)
- measure -> outer diameter of 1mm via -> 1.3mm
- as hole choose 1.4mm
Making an Small Pixel Matrix
Designing the Finger Part








-
ws2812 vs ws2812b
- leds should be the same
- different chips
- i take ws2812b. footprint should be the same also we dont know from the ordering.
- package actually is also different, but the package we have are 4 pads -> take that one
-
VSS is gnd
-
adding any vorwiderstand?
- no, on the strip there is also none.
- https://randomnerdtutorials.com/guide-for-ws2812b-addressable-rgb-led-strip-with-arduino/
-
but adding a widerstand to the first data in (this will be done on an extra pcb, to have all led modules the same): https://arduinogetstarted.com/tutorials/arduino-neopixel-led-strip
- spikes im strom reduzieren mit widerständen… ne geht nicht
- kein glätten.
- current limiting
- impedanz gleichhoch. weiß nicht wieso..
330
-
https://learn.adafruit.com/adafruit-neopixel-uberguide/best-practices
-
adding the resistor. put it onto the led pcb. following adafruit.
-
https://docs.kicad.org/master/it/eeschema/eeschema_assigning_footprints.html
-
create custom footprint
-
for the led with the diode, have 7mm and
-
has example schematic https://www.digikey.com/en/htmldatasheets/production/1829370/0/0/1/ws2812b
-
adjust schematic
-
bigger capacitor at power source necessary only when noting
-
… (check adafruit notes)
-
ws2812 be are powered with 5v. esp outputs 3.3v as logic. -> need level shifter https://www.digikey.com/en/products/detail/texas-instruments/TXB0104DR/1629101
-
however, it is not needed according to bene and ferdi (they tried it with 3v3)
Designing the Main PCB












Assembling









Programming: Testing the LEDs
- now programming
- https://arduinogetstarted.com/tutorials/arduino-neopixel-led-strip
- i then adjusted the sketch for it to blink faster
- it worked!
Programming: Combining With Gyro
- then wanted to try fade in
- then new sketch to couple this with the input of gyroscope
- assembled new sketch from gyro example and LED example
- ignore rotation around z axis (yaw)
- units are in rad (-PI, +PI)




1// before setup()
2float rad_to_brightness(float x) {
3 // x is in [-PI, +PI]
4 int heaviside = x >= 0;
5 return heaviside * (sin(2*x)/2 + 1);
6}
7
8// ...
9
10// loop()
11 // ...
12 // retrieving pitch, roll into struct `ypr`
13 // ...
14 // Make each LED lid up when the gyro is tilted in the corresponding direction.
15 pixels.clear();
16
17 // ..North/South (pitch)
18 pixels.setPixelColor(LED_NORTH, pixels.Color(0, rad_to_brightness(ypr.pitch)*255, 0));
19 pixels.setPixelColor(LED_SOUTH, pixels.Color(0, rad_to_brightness(-ypr.pitch)*255, 0));
20
21 // ..Eats/West (roll)
22 pixels.setPixelColor(LED_EAST, pixels.Color(0, 0, rad_to_brightness(ypr.roll)*255));
23 pixels.setPixelColor(LED_WEST, pixels.Color(0, 0, rad_to_brightness(-ypr.roll)*255));
24
25 pixels.show();
26// ...

Measuring the Matrix’ Maximum Power Consumption


-
dci, small currento
-
wrote sketch for powering all
-
4.87V
-
132.7mA = 132.7*1e-3A
-> 0.65W
Notes & TODOs
try magnetometer
pcb for vibration motor
- voltage
- cr2032/lir2032
- batterien selbst bauen
- halterung selbst designen + drucken
- bestellen: ladegeräte
got
- neopixel:
- add capacitor next to every led.
- check datasheet
- add large capacitor if you are using the big capacitor.
- check what else is there for every led to do.
- if capacitor too big it might need to charge first, that might lead to a lag.
- https://www.digikey.com/en/htmldatasheets/production/1829370/0/0/1/ws2812b
- motor driver
- check arduino cnc schield
npn vs pnp
- npn most of the time, pnp only for bridges & common anode.
schottky vs zener
- zener:
- werden in sperrichtung geschaltet, damit sie bei einer bestimmten spannung durchbrechen. verwended man indem man
- haben eine präzise durchbruchspannung bei der sie in die “sperrichtung” schaltet. in durchlassrichtung schaltet die schottkytiode normalerweise nicht (also sollte sie nicht)
- ???
Todos
- oled display
- mill programmer
- make the mobile light thing from tiago
- check sensors built by people in open time.
Reflections
What I Learned
- how to create copper planes in kicad
- how to integrate ws2812b leds into a pcb
- the wider pads/traces are designed the more stable the soldered connection will be.
What Went Wrong
What Went Well
- integrating sensor + leds
What I Would Do Differently
Digitial Files
- …
Use of Language Models
During writing this report, I used the following prompts asking ChatGPT 4o mini to …