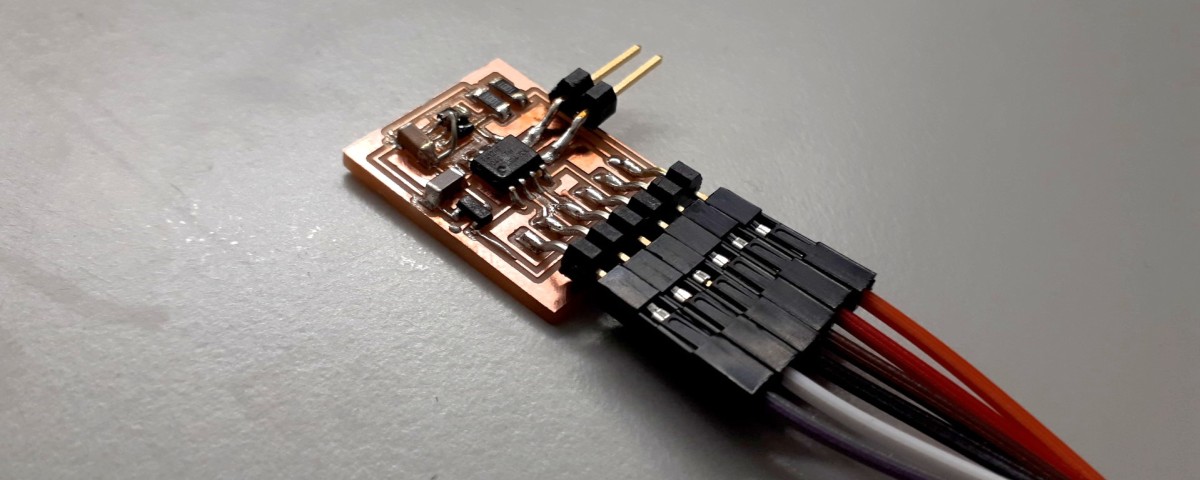
Week 9: Input Devices
Table of Contents
This week, we set up different sensors to send data to an MCU. In particular, I investigated an inertial measurement unit (IMU) as well as a vector magnetometer. Furthermore, I had more practice in SMD soldering.
This Week’s Tasks
- Group assignment:
- Probe an input device(s)’s analog levels and digital signals
- Document your work on the group work page and reflect on your individual page what you learned
- Individual assignment:
- Measure something: add a sensor to a microcontroller board that you have designed and read it.
Introduction to Input Devices
In this week’s lecture we learned about different types of sensors. This overview can be seen in this week’s lecture notes. What I learned new was among others that switches need to be debounced, that there are sensors that can measure not only magnetic field strength, but also direction, and that capacity can be measured using the step response of the two electrodes.
In the global open time, I learned that when you listen to sensors, averaging a few values might be good, since there might be noise in the input signals. Also, for analog measurements, the ATTiny1624 is to be preferred, since its ADCs are better in some sense.
Probing an Input Device
This week, we decided for the group assignment, to just take one of the sensors we look at this week and probing that. I probed the analog levels and the digital signals of the TLE493D. The documentation for that can be found under the vector magnetometer section. The group assignment page can be found here.
9-DoF Inertial Measurement Unit (IMU) & SH2
The first sensor I investigated was the 9-DoF IMU BNO085. More precisely, I looked at the corresponding board by Adafruit. The board contains an accelerometer, a gyroscope and a magnetometer. It can be used to measure pitch, roll, and yaw, as well as acceleration in X, Y, and Z direction. The different sensors can be used to calibrate each other. The board comes with a library for the arduino environment. Either in the library or on the chip, there is some sensor fusion happening.
Now there are different sensors on the board. It could be that one only wants to read the accelerometer, or only the gyroscope, or that one wants to read the calibrated values of the gyroscope. How to write this down in software? Adafruit implemented a (software) standard called SH2. One can read the SH2 reference manual for details. This standard defines so-called virtual sensors. These sensors are abstract sensors that do not exist physically, but in software. They behave like sensors to the programmer in the way that they also output values at some sample rate. Under the hood, however, the output values of one virtual sensor are retrieved by taking the values of one or more physical sensor and process them. In that way, one can perform all kinds of preprocessing, such as calibration or fusion. As an example, one could fuse the output values of two physical sensors both measuring the same thing but in a different way to get a more precise measurement. These refined values are then reported as an output of a virtual sensor with e.g. the name more_precise_sensor
. I found about this by checking the source code of the library for the board.

The following explanations are taken from the SH2 reference manual linked earlier. I reported it here so one can understand more easily what each sensor does. Unfortunately, I did not find out, what Q point means.
Virtual Sensor | Description |
---|---|
Linear Acceleration (0x04) | The linear acceleration sensor reports the acceleration of the device minus gravity. The units are m/s^2. The Q point is 8. The report ID is 0x04. |
Raw Gyroscope (0x15) | The gyroscope sensor reports raw readings from the physical gyroscope MEMS sensor. The units are ADCs. Interpretation of the reported values is sensor dependent. The report ID is 0x15 |
Gyroscope Calibrated (0x02) | The gyroscope calibrated sensor reports drift-compensated rotational velocity. The units are rad/s. The Q point is 9. The report ID is 0x02. |
Gyroscope Uncalibrated (0x07) | The gyroscope uncalibrated sensor reports rotational velocity without drift compensation. An estimate of drift is also reported. The units for both values are rad/s. The Q point for both values is 9. The report ID is 0x07. |
Raw Magnetometer (0x16) | The magnetometer sensor reports raw readings from the physical magnetometer sensor. The units are ADCs. Interpretation of the reported values is sensor dependent. The report ID is 0x16. |
Magnetic Field Calibrated (0x03) | The magnetic field calibrated sensor reports the geomagnetic field calibrated for hard and soft iron effects such that the vector is aligned with the declination and heading of Earth’s magnetic field. The units are uTesla. The Q point is 4. The report ID is 0x03. |
Magnetic Field Uncalibrated (0x0F) | The magnetic field uncalibrated sensor reports the geomagnetic field calibrated for soft iron effects only. Estimates for the hard iron bias are also reported. The units for both values are uTesla. The Q point for both values is 4. The report ID is 0x0F |
Rotation Vector (0x05) | The rotation vector sensor reports the orientation of the device. The format of the rotation vector is a unit quaternion. The Q point is 14. In addition an estimate of the heading accuracy is reported. The units for the accuracy estimate are radians. The Q point is 12. The report ID is 0x05. |
Game Rotation Vector (0x08) | The rotation vector sensor reports the orientation of the device. The format of the rotation vector is a unit quaternion. The Q point is 14. The report ID is 0x08. |
Geomagnetic Rotation Vector (0x09) | The geomagnetic rotation vector sensor reports the orientation of the device. The format of the rotation vector is a unit quaternion. The Q point is 14. In addition an estimate of the heading accuracy is reported. The units for the accuracy estimate are radians. The Q point is 12. The report ID is 0x09. |
ARVR-Stabilized Rotation Vector (0x28) | The ARVR-stabilized rotation vector sensor reports the orientation of the device. Accumulated errors are corrected while the device is in motion, which limits the appearance of discontinuities or jumps in data. The format of the rotation vector is a unit quaternion. The Q point is 14. In addition an estimate of the heading accuracy is reported. The units for the accuracy estimate are radians. The Q point is 12. The report ID is 0x28. |
ARVR-Stabilized Game Rotation Vector (0x29) | The ARVR-Stabilized game rotation vector sensor reports the orientation of the device. |
Gyro-Integrated Rotation Vector (0x2A) | The Gyro-Integrated Rotation Vector sensor reports the absolute orientation of the device as determined by integrating gyroscope data at every gyroscope sample and correcting to the more-accurate Rotation Vector periodically. This sensor can support higher data rates than the more-accurate Rotation Vector can. The format of the output report is a unit quaternion and calibrated gyroscope data. The Q point of the angular position is 14 and angular velocity is 10. The report ID is 0x2A. |
The corresponding names for the virtual sensors as they are defined in the Adafruit library can be found in the sh2_SensorValue.h. From the above descriptions it seems most reasonable to use the SH2_GYRO_INTEGRATED_RV for high-frequency pitch-roll-yaw reports and the SH2_ARVR_STABILIZED_RV for reports with lower frequency, but a higher accuracy. For XYZ-acceleration, the SH2_LINEAR_ACCELERATION seems to be the most reasonable one.
Then, I set up the sensors for I2C communication with the ESP32S3.

For testing, I the board, I used one of the provided example programs. The values I plotted using the serial plotter. In the example code, there is a part where you can define, which exact virtual sensor you would like to receive data from. The following code is taken from the rotation_vector
example. Thereby, the bno08x
object is initialized earlier. This is an abstraction of the Adafruit board and contains the communication utilities.
1// in `setup()`
2// ...
3// Here is where you define the sensor outputs you want to receive
4void setReports(void) {
5 Serial.println("Setting desired reports");
6 if (! bno08x.enableReport(SH2_GAME_ROTATION_VECTOR)) {
7 Serial.println("Could not enable game vector");
8 }
9}
10// ...
The sensor value can then be read by the following code (adapted from the same example):
1// in `loop`
2// ...
3if (bno08x.getSensorEvent(&sensorValue)) {
4 Serial.print("Game Rotation Vector - r: ");
5 Serial.print(sensorValue.un.gameRotationVector.real);
6 Serial.print(" i: ");
7 Serial.print(sensorValue.un.gameRotationVector.i);
8 Serial.print(" j: ");
9 Serial.print(sensorValue.un.gameRotationVector.j);
10 Serial.print(" k: ");
11 Serial.println(sensorValue.un.gameRotationVector.k);
12}
13// ...
In the same way, the quaternion_yaw_pitch_roll
example works, but it uses another virtual sensor. I flashed this example to the MCU and displayed the values. A demonstration can be seen below.
Additionally, I displayed the values of the accelerometer. I compared the linear accelerometer with the accelerometer to see if there is a major difference in signal quality. Again, the code was the same as above. Different was that now, I enabled and read multiple reports.
1// in `setup`
2// ...
3// Here is where you define the sensor outputs you want to receive
4void setReports(void) {
5 Serial.println("Setting desired reports");
6 if (!bno08x.enableReport(SH2_ACCELEROMETER)) {
7 Serial.println("Could not enable accelerometer");
8 }
9 if (!bno08x.enableReport(SH2_LINEAR_ACCELERATION)) {
10 Serial.println("Could not enable linear acceleration");
11 }
12}
13// ...
14
15// in `loop`
16// ...
17if (!bno08x.getSensorEvent(&sensorValue)) {
18 return;
19}
20
21switch (sensorValue.sensorId) {
22 case SH2_ACCELEROMETER:
23 // print values
24 break;
25 case SH2_LINEAR_ACCELEROMETER:
26 // print values
27 break;
28// ...
Below, a demonstration of the program can be seen.
lac\n
is printed and every time, a report from the accelerometer arrives, a acc
is printed. The output looked like this.

Vector Magnetometer
The second sensor I tried was the vector magnetometer. This sensor can measure the strength of its surrounding magnetic field strength, just like a hall sensor, and also its direction. The exact name of the IC was TLE493DA2B6HTSA1 SENSOR HALL EFFECT I2C. My plan was to use the PCB design that includes an ATTiny412 for conversion of the sensor data from I2C to UART to be sent to the main MCU and the code to put on that ATTiny which both was provided by Neil. I was looking forward to do this, because I liked fabricating PCBs and I wanted to learn how to program an ATTiny which is soldered onto a custom board.

Fabricating the PCB
Since at this point, we only had two-sided FR1 at the lab, I took an FR1 plate and removed the copper foil from one side.








Setting Up the Sensor
For programming the ATTiny, I used the Quentorres board one of my peers brought from the FAB24 conference and followed this tutorial for setting it up.


At this point, I wondered which of the two provided files to flash: the .ino file, or the .c file? Comparing the two program I found that both programs did the same, but one is using the Arduino library and the other not. This can be seen most easily by understanding the main loops of both programs. I chose to flash the .ino
file.
Note, that with the Quentorres, there might be an issue if you are using linux. For this, scroll down the Quentorres documentation page and read the corresponding section. One needs to update pymcuprog
for it to work.
(venv) jakob@t480 ~/Documents/studium/courses/ongoing/fabacademy/jakob-lerch/website/content/assignments/week-09_input-devices/files/20-vec-mag/tle_controller/build/megaTinyCore.megaavr.atxy2 ±main⚡ » pymcuprog write -t uart -u /dev/ttyACM0 -d attiny412 -f tle_controller.ino.hex --erase --verify
Connecting to SerialUPDI
Pinging device...
Ping response: 1E9223
Erasing device before writing from hex file...
Writing from hex file...
Writing flash...
Verifying flash...
OK
I then continued connecting the sensor to the ESP32S3.



1#include <HardwareSerial.h>
2
3HardwareSerial MagFieldSerial(0);
4
5void setup() {
6 Serial.begin(115200);
7 MagFieldSerial.begin(115200, SERIAL_8N1, 6, 7); // Configure pins TX=D6, RX=D7.
8 while (!Serial);
9 Serial.println("Ready");
10}
11
12void loop() {
13 if (MagFieldSerial.available()) {
14 char byte_rcvd = MagFieldSerial.read();
15 char byte_string[3];
16 sprintf(byte_string, "%02X", byte_rcvd);
17 Serial.println(byte_string);
18 }
19}
I then noticed that there is a signal on the pin labelled “RX” on the sensor’s side and the pin labelled “TX” was of static voltage.


.c
version). This code did the same as the .ino
code, but without using the Arduino libraries. Hence, it was more elaborate. The bytes are written to the outside of the chip by this line put_char(&serial_port_out,serial_pin_out,data[0]);
where serial_pin_out
had the value 6. Following the ATTiny library, pin 6 was the one that was labelled RX in the board layout of the sensor board. I therefore connected the RX pin of the ESP32S3 with the pin labelled “RX” of the sensor board.

I then received hex values from the sensor. However, now the list of outputs stopped after a certain amount of bytes (128 many).

Open Questions
The following questions I was not able to answer within the week.
- Do i need to calibrate the accelerometer? As far as I read from the SH2 reference, this is not done automatically.
- How do i get multiple sensor reports? As far as I understand, one can only get one of those values in one cycle.
1void loop() {
2 delay(10);
3
4 if (bno08x.wasReset()) {
5 Serial.print("sensor was reset ");
6 setReports();
7 }
8
9 if (!bno08x.getSensorEvent(&sensorValue)) {
10 return;
11 }
12
13 switch (sensorValue.sensorId) {
14
15 case SH2_ACCELEROMETER:
16 Serial.print("acc");
17 //Serial.print("acc_z:"); Serial.println(sensorValue.un.accelerometer.z); Serial.print(",");
18 break;
19 case SH2_LINEAR_ACCELERATION:
20 Serial.print("lac");
21 Serial.print("\n");
22 //Serial.print("linacc_z:"); Serial.println(sensorValue.un.linearAcceleration.z); Serial.print(",");
23 break;
24 }
25}
Reflections
What I Learned
- I learned a lot about SMD soldering
- I learned about the SH2 standard for sensor reports.
- I learned how to use the logic analyzer.
- Flux conducts heat very well, take heat of soldering iron down when using it.
What Went Wrong
- Soldering the magnetometer was a long process. I learned much there.
- I did not get the vector magnetometer to work.
What Went Well
- This week, I refined my final project page to finally have some requirement list.
Digitial Files
- Inertial measurement unit (Adafruit BNO085 board)
- Removing copper from two-sided PCB stock
- Vector magnetometer
- Neil’s design files
- hello.TLE493D.t412.interior.png
- hello.TLE493D.t412.traces.png
- mag_board_.2traces_.8edgecut.nc (the tool path I created from the design)
- Code for the ATTiny
- Neil’s design files
- Code for receiving UART messages and printing them to the serial monitor
- Logic analyzer output
Use of Language Models
I used no language models for writing this report.