On This Page
Week 4: Embedded Programming
Programming Microcontrollers
Date: February 12 - February 19, 2025
Quick Summary
- Skills Learned: PCB design, milling machine operation, electronic components
- Hardware Used: Arduino Uno, UPDI Programmer, Multimeter
- Software Used: Arduino IDE, PlatformIO, VS Code
- Files: Resources, Presentation, Resource Review, Assessment Criteria, Tutorials
- Recitation: Introduction to Programming
- Key Concepts: Microcontroller architectures (RISC-V, ARM), Development environments (IDE, Toolchains), Programming languages (Python, MicroPython, C++), Simulation, Debugging, Processor families (ESP32, nRF), Wireless protocols (WiFi, Bluetooth), Embedded ML
- Challenges Faced: N/A
- Topics: Architecture, Memory Peripherals, Word Size, Processor Families, Vendors, Packages, Embedded Languages, In-system Development, Development Environments, Development Boards, Operating Systems, Clocks, Serial Communication, Debugging, AI
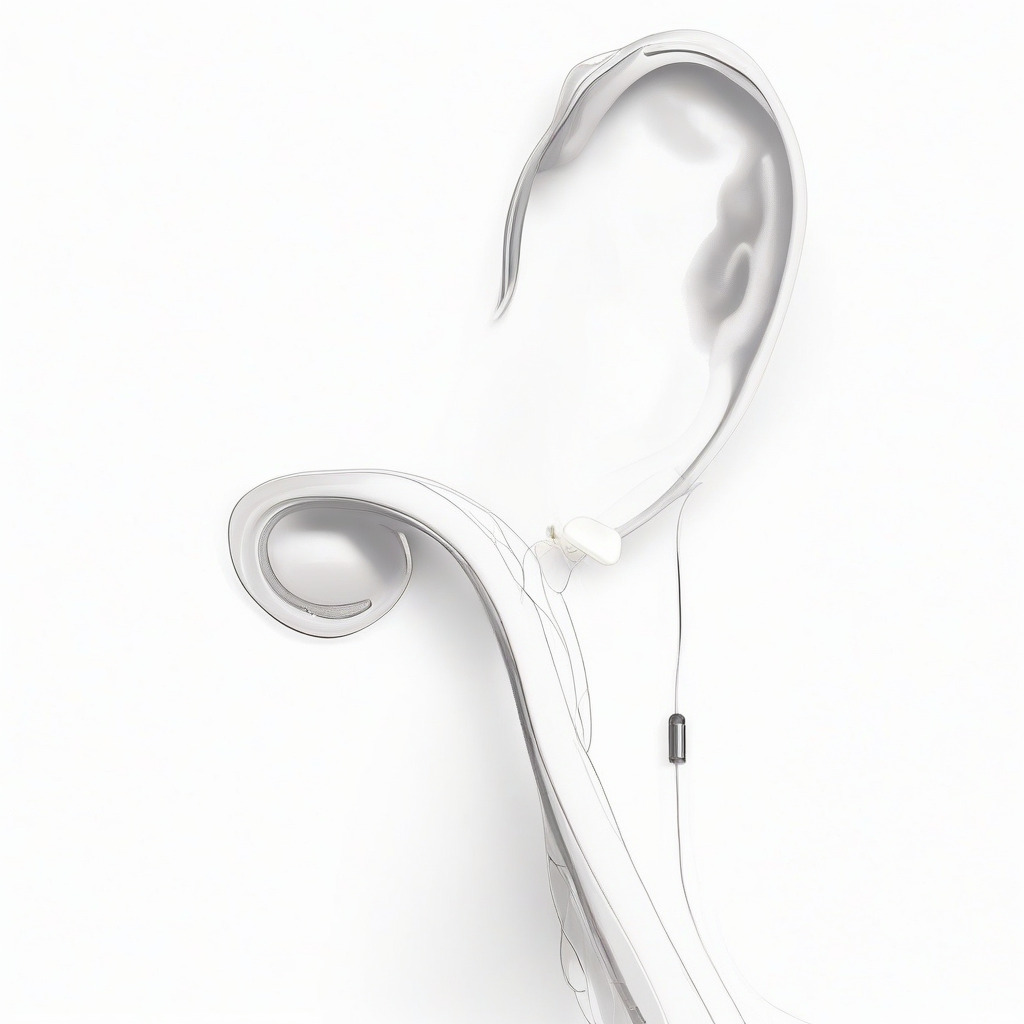
The ear hears
Introduction
This week I explored embedded programming - the art of writing software for microcontrollers. In this week's global lecture, we covered processor architectures, development environments, and programming languages, with a focus on real-time applications and hardware interaction.
Process Documentation
Programming micro:bit in Python
Development Workflow
For my part of the group project (which dictated which microcontroller I worked with) I was assigned the BBC Micro:bit (nRF52833 processor - datasheet). To test my understanding of the microcontroller, and to test input/output, I created a simple program that displays a scrolling message and plays a tune once powered on.
Step 1: Setup
First, visit Python Editor for micro:bit to access the online Python editor.
micro:bit Python Editor Interface
Step 2: Writing the Code
The IDE facilitates developing patches in Python and communication with the micro:bit: This Python code creates an interactive program that scrolls a message and plays music:
# Imports go at the top
from microbit import *
import music
# Code in a 'while True:' loop repeats forever
while True:
display.show(Image.HEART)
display.scroll('FabLabulous', delay=90, loop=True, wait=False)
music.play(music.PYTHON)
sleep(1000)
Python code in micro:bit editor
Step 3: Testing in the IDE Simulator
Before uploading to the physical device, we can test our code in the built-in simulator:
Testing the code in the micro:bit simulator.
Step 4: Testing on the micro:bit
Connect your micro:bit to your computer using the supplied USB cable. The LED matrix will light up, indicating that the device is powered on. Once connected, follow these steps:
- Click the 'Connect' button to pair with your micro:bit
- Click 'Send to micro:bit' to flash the code
- Press button A to see the message scroll and hear the tune
micro:bit connected via USB, ready for flashing.
Step 5: See it in Action!
The micro:bit running the demo code.
Definitions
(As explained by LLM claude-3.5-sonnet - February 2025)
Toolchains
A toolchain is a set of programming tools used to create a software product. In embedded systems, this typically includes:
- Compiler: Converts source code into machine code
- Linker: Combines object files into executable programs
- Debugger: Helps find and fix code problems
- Programmer: Uploads code to the microcontroller
Example: The micro:bit Python toolchain includes:
- MicroPython compiler (converts Python to machine code)
- Web-based Python editor (development environment)
- Built-in simulator (testing and debugging)
- DAPLink firmware (handles USB connection and programming)
- micro:bit runtime (provides hardware abstraction)
Development Workflows
A development workflow is the process and steps taken to create, test, and deploy embedded software. A typical workflow includes:
- Planning: Define requirements and design
- Development: Write and organize code
- Testing: Verify functionality
- Deployment: Program the device
- Maintenance: Debug and update as needed
Example: A typical micro:bit Python development workflow:
- Write code in the online Python editor (python.microbit.org)
- Test code in the built-in simulator
- Connect micro:bit via USB
- Flash code to device using WebUSB
- Debug using LED display and REPL console
- Iterate based on testing results
- Share .hex file or Python source code
Notes
Processors and Development Boards
An overview of microcontroller families and their key features, focusing on popular platforms for embedded development.
Benchmarks
Reference benchmarks: Hardware | Software
Espressif - ESP Family
GitHub - Repository for Espressif's projects and libraries.
Arduino Core for ESP32 - Arduino core for ESP32 development.
Comparing the ESP32 Family - A comprehensive overview of the different ESP32 microcontrollers, their features, and upgrade paths.
ESP32 Family Comparison
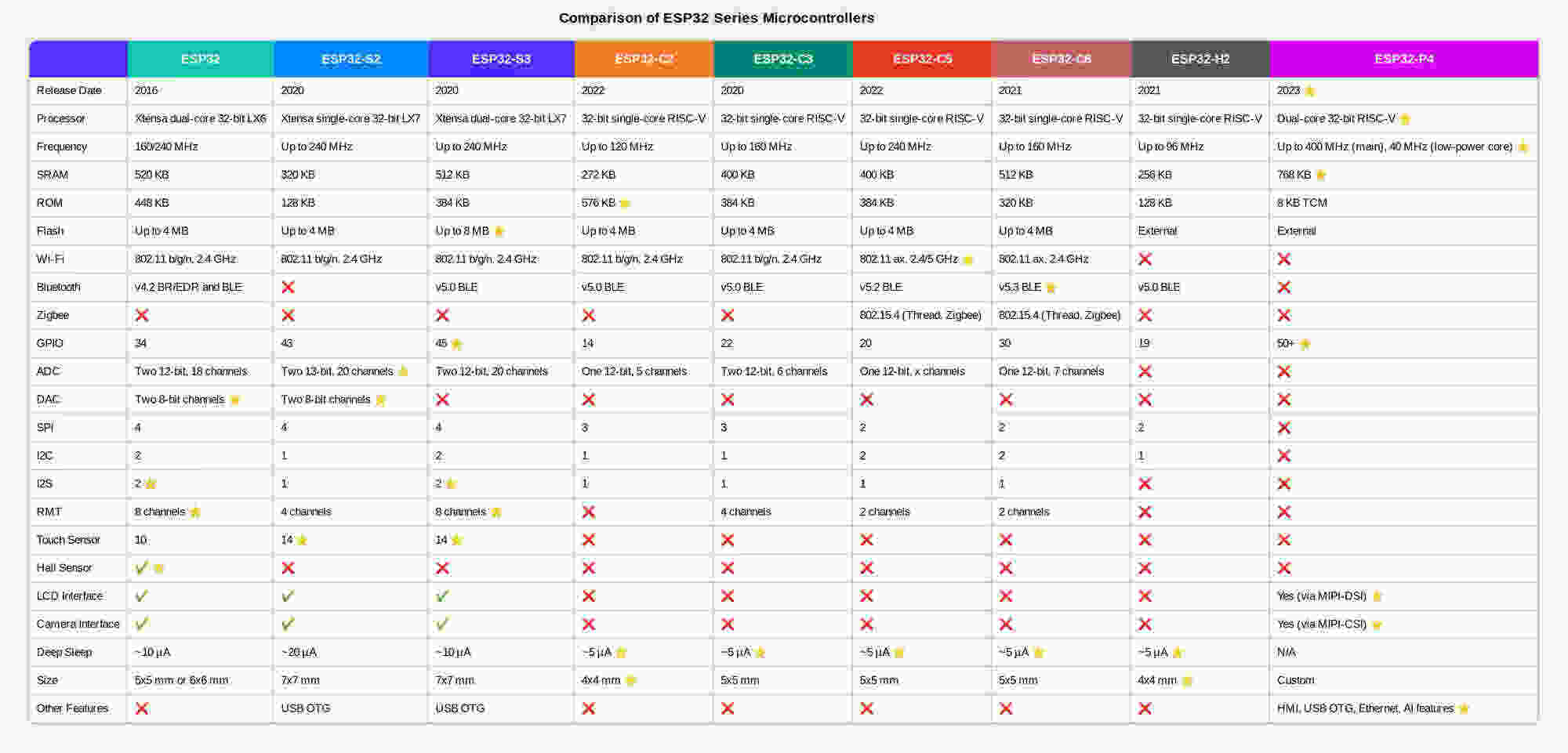
Detailed comparison table of ESP32 microcontroller family features
ESP32-C3
Product Website: ESP32-C3 SoC
A cost-effective RISC-V MCU featuring Wi-Fi and Bluetooth 5 (LE) connectivity. Key features include: 32-bit RISC-V core with 160 MHz clock speed, 400 KB RAM, 22 GPIOs, secure boot capability, and hardware acceleration for cryptographic algorithms. Ideal for secure IoT applications.
Seeed Studio - XIAO Series
The Seeed Studio XIAO Series represents a collection of thumb-sized, powerful microcontroller units designed for space-conscious projects requiring high performance and wireless connectivity. These Arduino-compatible boards incorporate popular hardware platforms like ESP32, RP2350, RP2040, nRF52840, and SAMD21, making them ideal for TinyML and edge computing applications.
The XIAO series stands out for its compact form factor while maintaining rich features including:
- Multiple processor options (ARM Cortex-M, RISC-V)
- Wireless capabilities (WiFi, Bluetooth, Matter)
- Extensive development platform support (Arduino, CircuitPython, MicroPython)
- Ultra-low power consumption modes
Compatible Software & Development Platforms
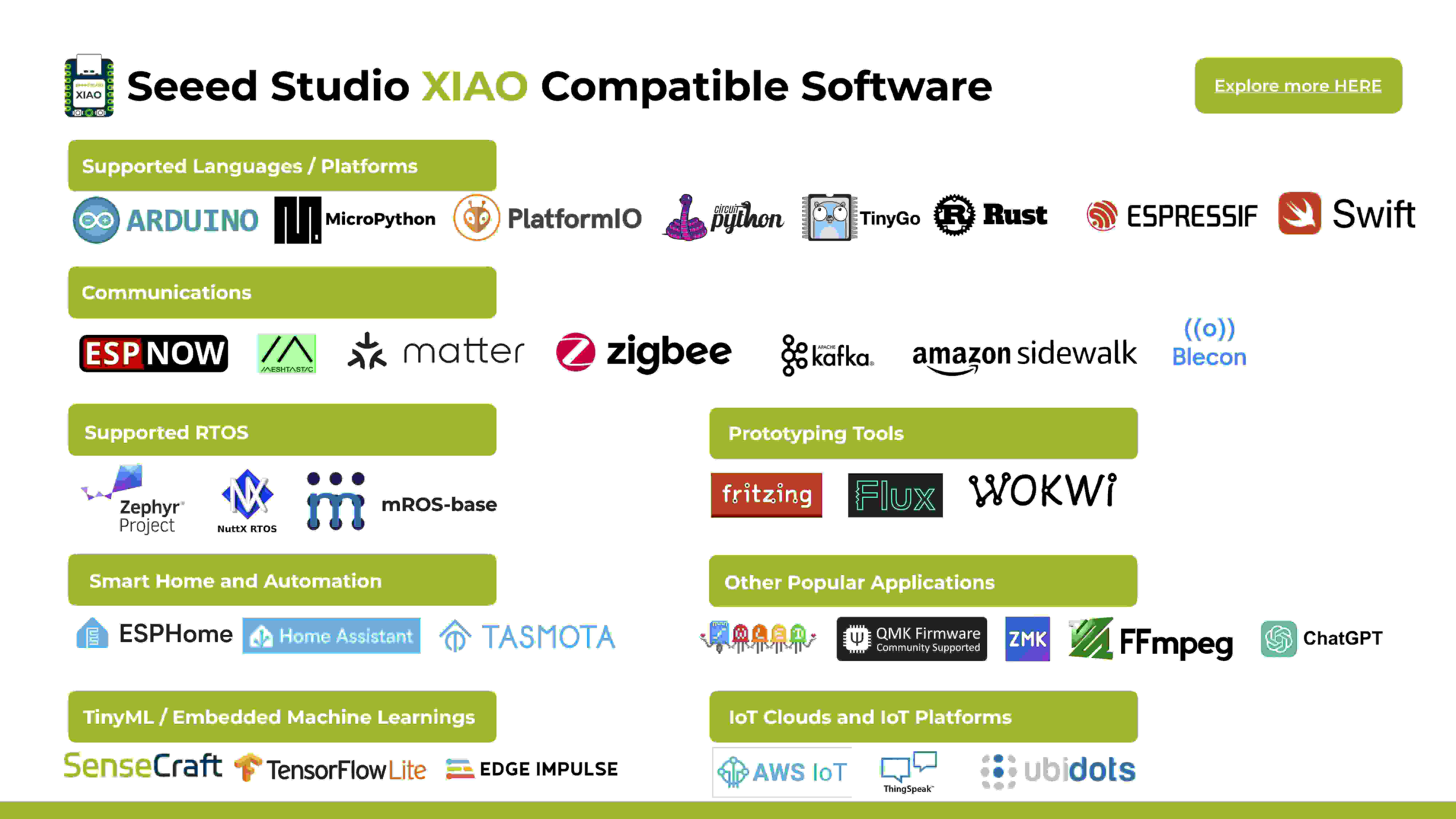
Seeed Studio XIAO compatible software platforms overview
Seeed Studio XIAO nRF52840 Series
Seeed Studio XIAO nRF52840 - A powerful wireless microcontroller featuring Nordic's nRF52840 MCU with Bluetooth 5.0 connectivity. This compact board combines a 32-bit ARM Cortex-M4 processor running at 64MHz with rich interfaces and ultra-low power consumption, making it ideal for IoT and wearable projects.
XIAO nRF52840 Family
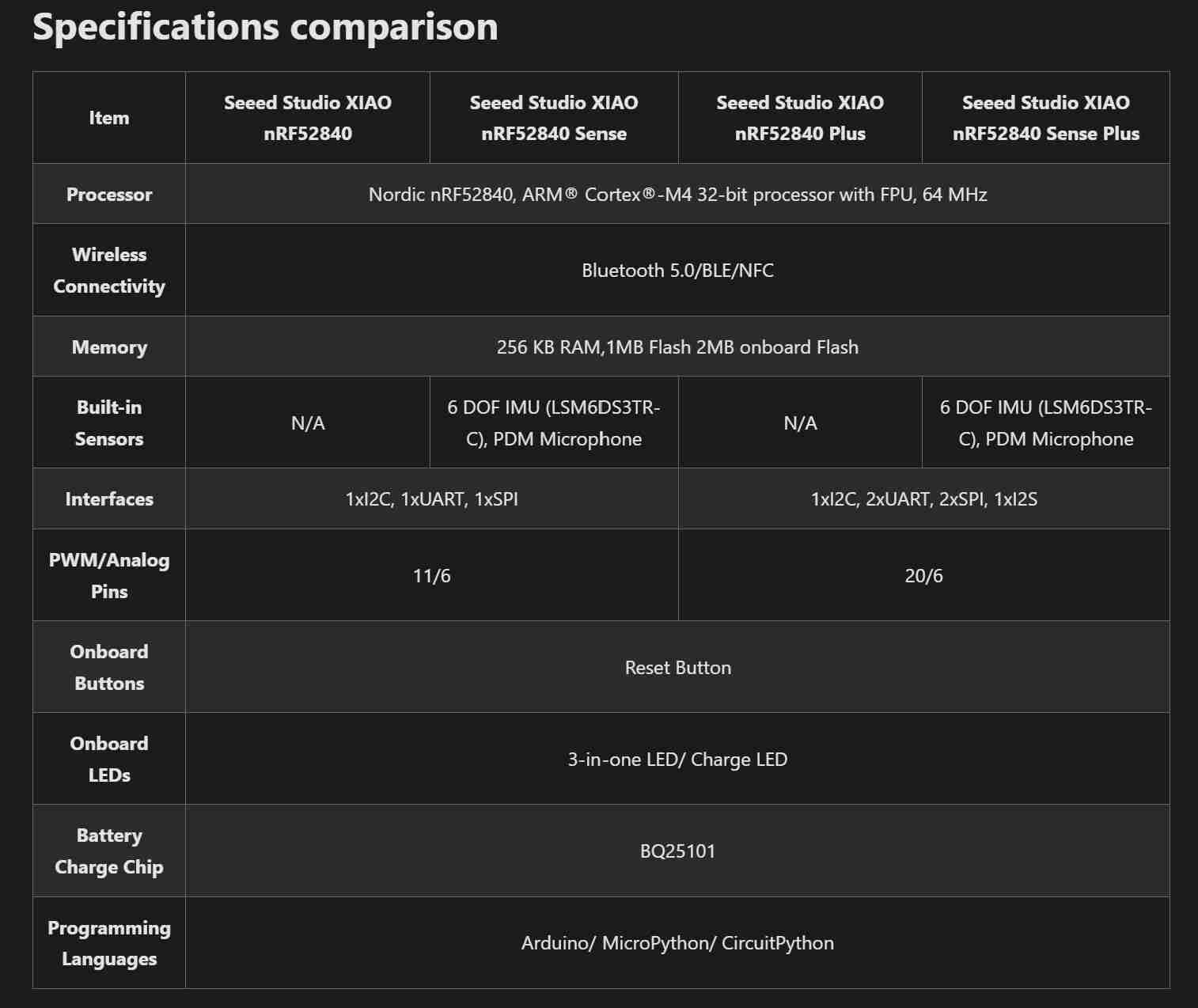
Comparison table of XIAO nRF52840 variants
Seeed Studio XIAO RP2040
Seeed Studio XIAO RP2040 - A powerful yet compact microcontroller featuring the Raspberry Pi RP2040 chip. This thumb-sized board combines a dual-core ARM Cortex M0+ processor running at up to 133MHz with 264KB of SRAM and 2MB of Flash memory, making it ideal for space-constrained projects requiring significant processing power.
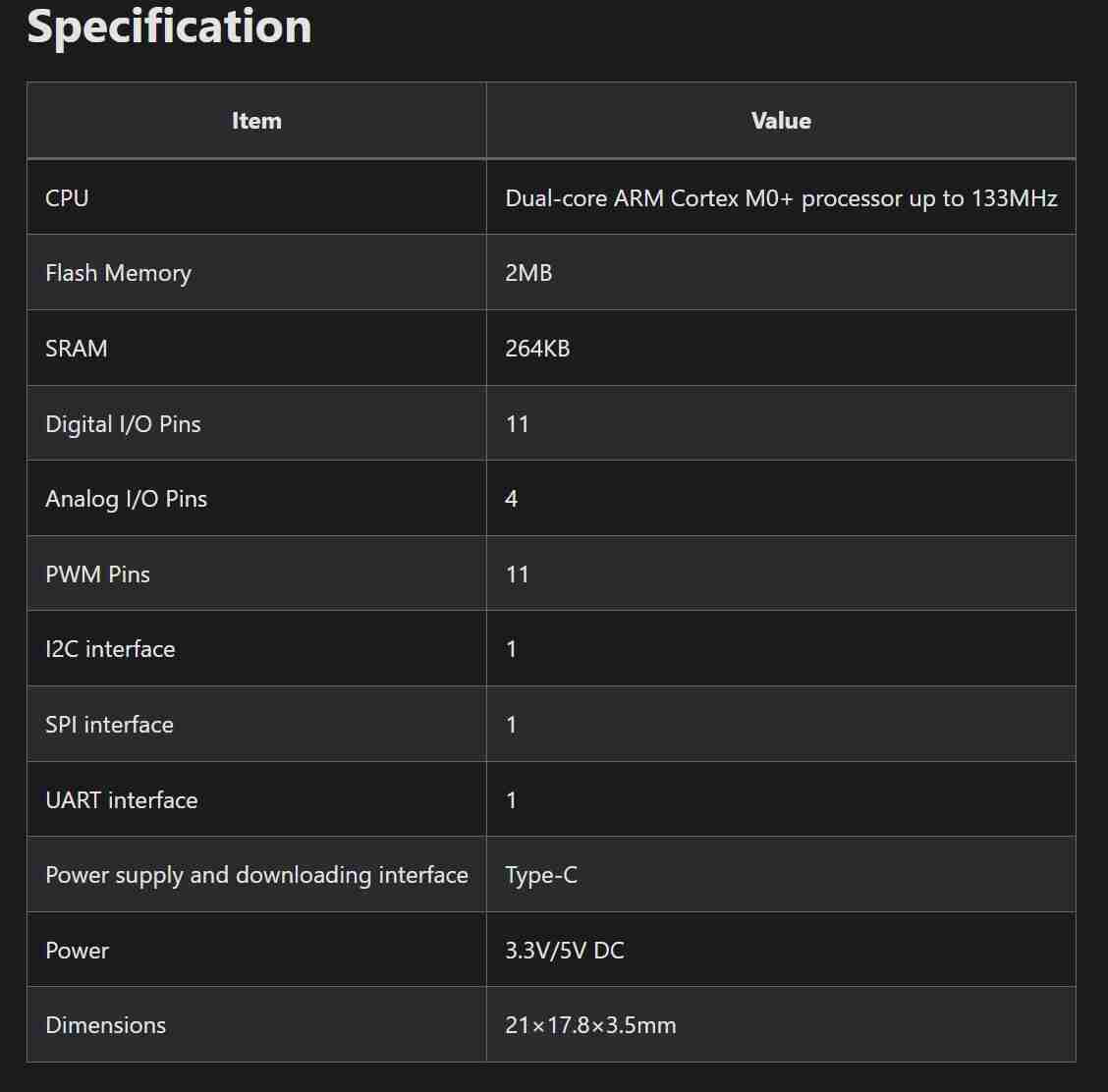
XIAO RP2040 hardware overview
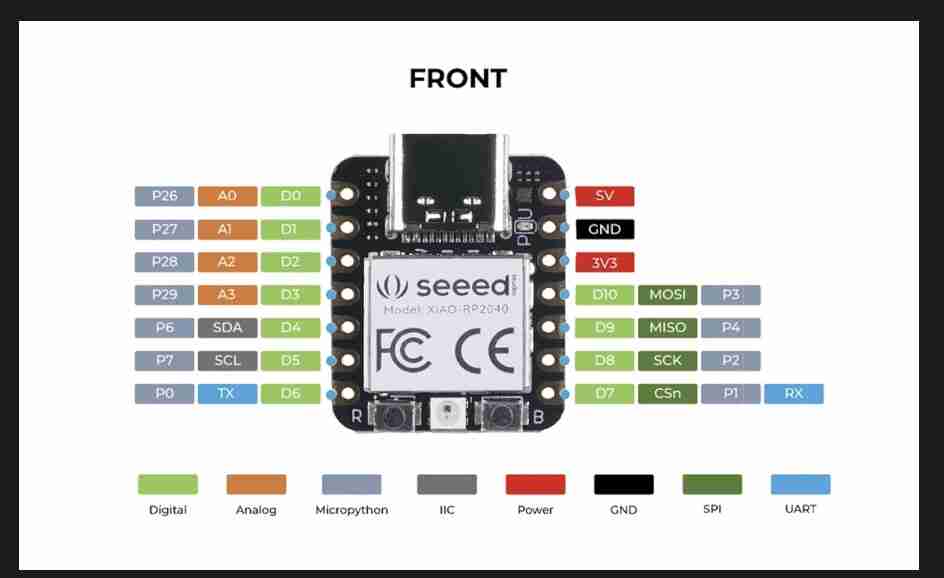
XIAO RP2040 pinout diagram
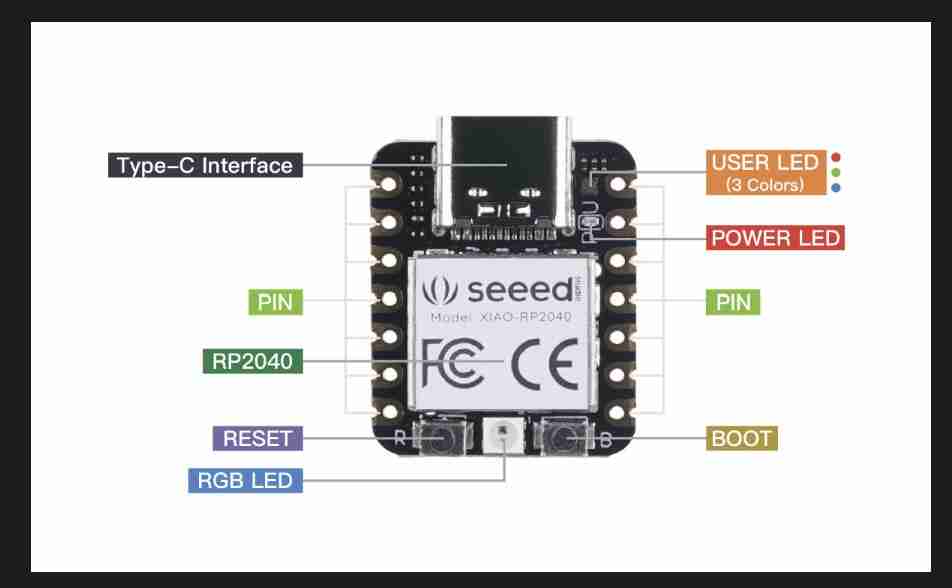
XIAO RP2040 dimensions
BBC - micro:bit
The micro:bit is a pocket-sized computer designed by the BBC for use in computer education. Originally created for UK schools to inspire digital creativity in young people, it has become a global educational tool ideal for students aged 8-16 years old and beginners in programming and electronics. Full technical specifications and hardware details can be found in the micro:bit Hardware Documentation.
nRF52833 Datasheet
This is the processor used on the BBC micro:bit. You can download the datasheet here.
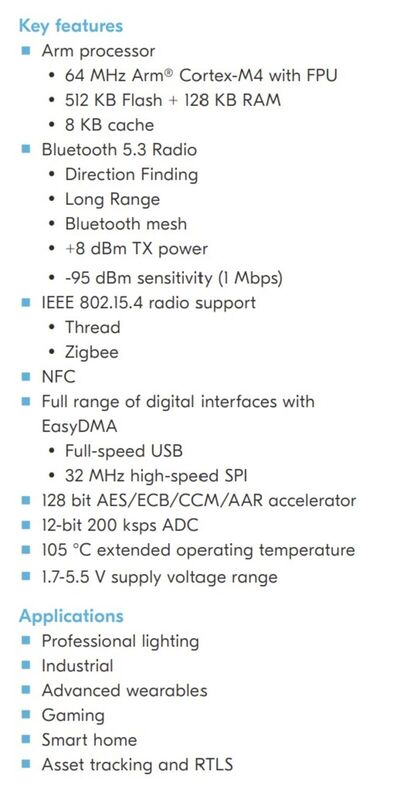
Key Features of nRF52833
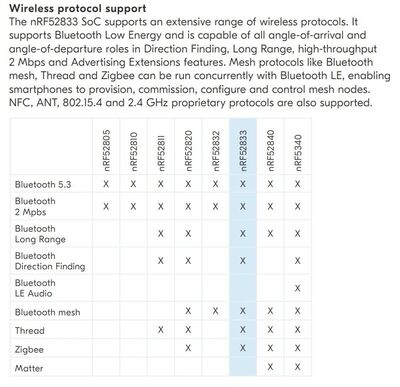
Wireless Protocol Support for nRF52833
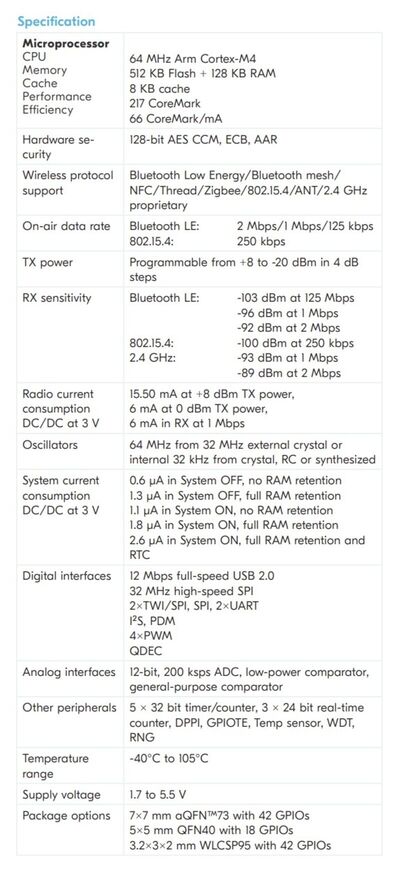
Specifications of nRF52833
Key strengths:
- Educational Focus - Designed specifically for learning, with an intuitive interface and gradual learning curve
- Immediate Feedback - Built-in LED display and sensors provide instant visual feedback for code execution
- No Setup Required - Works straight out of the box with web-based programming interface
- Robust Design - Durable construction suitable for classroom environments and young users
- Progressive Learning Path - Supports transition from block-based to text-based programming
- Affordable - Cost-effective solution for schools and individual learners
Key features include:
- Nordic nRF52833 processor with Bluetooth 5.1 BLE - A general-purpose multiprotocol SoC with a Bluetooth Direction Finding capable radio, qualified for operation at an extended temperature range of -40°C to 105°C. It is the 5th addition to the industry leading nRF52 Series and is built around a 64 MHz Arm Cortex-M4 with FPU, and has 512 KB flash and 128 KB RAM memory available for higher value applications.
- 25 LED matrix display
- 2 programmable buttons
- Motion sensors (accelerometer and compass)
- Temperature and light sensors
- Edge connector with large pins for external connections
- USB interface for programming
Programming Options
- Microsoft MakeCode - Block-based and JavaScript programming environment
- Python Programming Environment for micro:bit
- Python (via MicroPython) - For text-based programming
- Scratch integration - Familiar environment for young coders
Educational Resources
- Complete, editable computing units for ages 7-11
- First Steps with micro:bit
- Advanced projects for ages 11-14 covering computing fundamentals, cyber security, and cryptography
- Cross-curricular links for art, science, and geography
- Global sustainability and problem-solving projects
Reflection
This week provided a broad overview of the embedded programming landscape. Reading datasheets for various microcontrollers like the nRF52833 (used in the micro:bit) offered insight into specific hardware capabilities, architectures (ARM vs RISC-V), and communication protocols. Programming the micro:bit using the online Python editor and its simulator demonstrated a user-friendly development workflow suitable for beginners. Exploring different processor families (ESP32, XIAO series) and development tools (MicroPython, Thonny, PlatformIO, Faust, TensorFlow Lite) highlighted the diversity of options available for different project needs, from basic blinking LEDs to more complex tasks involving wireless communication or embedded machine learning. Understanding toolchains and development workflows is crucial for efficiently moving from code to functional hardware.
Project Files
- micro:bit Demo Code (Scroll & Sound) - Zip archive containing the .hex file for the micro:bit demo program that scrolls "FabLabulous" and plays a tune.
- nRF52833 Datasheet (Zip) - Detailed technical documentation for the Nordic nRF52833 SoC used in the micro:bit.
Weekly Assignments
Group Assignment
This week features a collaborative group assignment where we:
- Demonstrate and compare the toolchains and development workflows for available embedded architectures
- Document our work to the group work page and reflect on our individual page what we learned
View our group's collaborative work here: Creative Spark Group Assignment - Embedded Programming