09 - Input Devices
individual assignment:
measure something: add a sensor to a microcontroller board
that you have designed and read it
So, my board is currently being cut in our wonderful Roland machine so here are some playtime moments using the Barduino board the barcelona lab has provided us with.
The input devises investigated on this page are:
Variable resistors / Potentiometer
Variable resistors
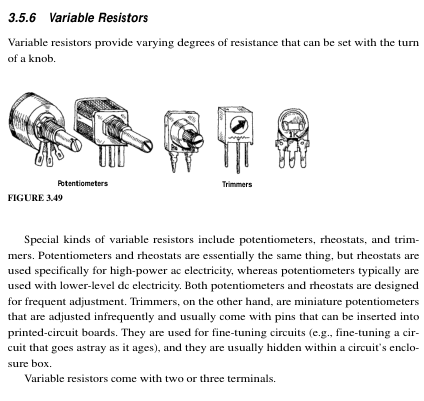
We made a little circuit with a potentiometer and wrote some code to give us out a voltage reading.
void setup() {
// put your setup code here, to run once:
pinMode(17, INPUT);
Serial.begin(115200);
}
void loop() {
// put your main code here, to run repeatedly:
int lecture = analogRead(17);
Serial.println(lecture);
delay(50);
}
The potentiometer works using a voltage divider, varying the resistance of a resistor in a resistor in series in order to change the output sent to the pin.
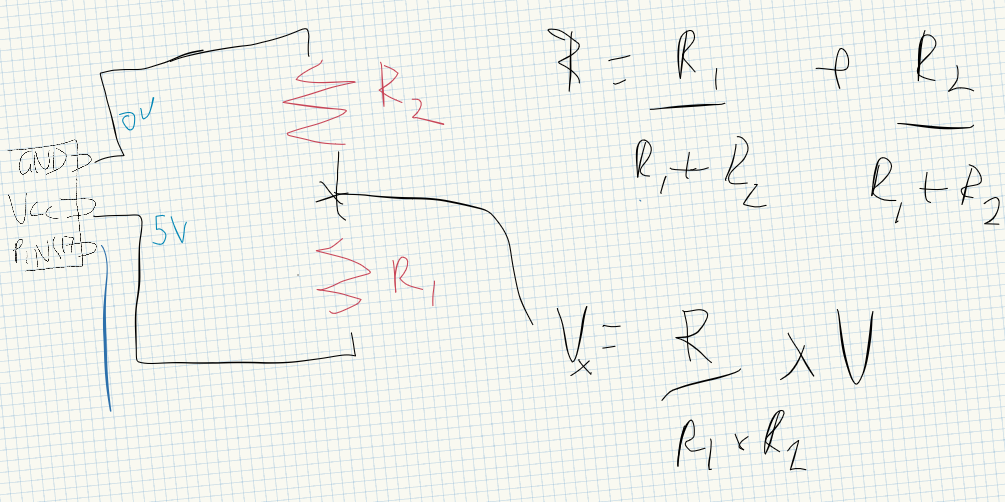
Playing around with the potentiometer and turning the knob gives us a variable volage output. 4095V associated with the 3.3V output from our barduino board.
KY035 Analog Hall Magnetic Sensor
We used the KY035 hall magnetic sensor to see how it is affected by a metal bolt.
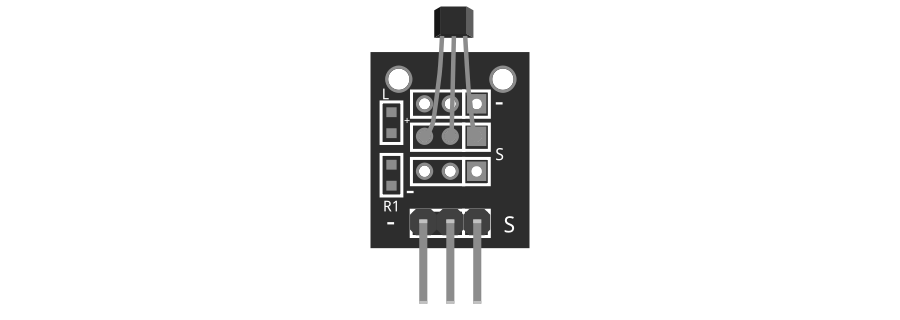
https://arduinomodules.info/download/ky-035-analog-hall-magnetic-sensor-fritzing-part/
You can find the arduino code below followed with the variable voltage response as we move it closer and further away from the sensor.
int sensorPin = 17; // interface pin with magnetic sensor
int val; // variable to store read values
void setup() {
pinMode(17, INPUT); // set analog pin as input
Serial.begin(115200); // initialize serial interface
}
void loop() {
val = analogRead(sensorPin); // read sensor value
Serial.println(val); // print value to serial
delay(100);
}
The standing voltage was half of 4095 value corresponding to 3.3V. The voltage drops or increases depending on the polarity.
KY027 magic light cup effect
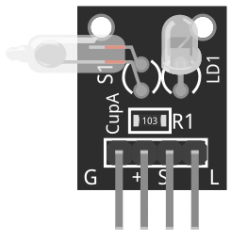
The following is copied from https://arduinomodules.info/ky-027-magic-light-cup-module/
Using PWM to drive the LEDs on each module, you can achieve the effect of light being “magically” transferred from one module to the other when tilting them, similar to pouring water from one cup to the other, hence the name.
In this Arduino sketch we’ll use both modules to create the magic light cup effect. The mercury switches in each module provide a digital signal that is used to regulate the brightness of the LEDs using using PWM.
Place the modules so the mercury switches on each other are facing in the opposite directions. Tilting the modules will decrease the brightness on one module while increasing it on the other one, creating the illusion of light magically passing from one module to the other.
We set up our barduino with two KY027s on separate sides of a breadboard and modified the arduino code to correspond to our pins. The Arduino code is as follows:
int ledPinA = 15;
int switchPinA = 13;
int switchStateA = 0;
int ledPinB = 8;
int switchPinB = 10;
int switchStateB = 0;
int brightness = 0;
void setup()
{
pinMode(ledPinA, OUTPUT);
pinMode(ledPinB, OUTPUT);
pinMode(switchPinA, INPUT);
pinMode(switchPinB, INPUT);
}
void loop()
{
switchStateA = digitalRead(switchPinA);
if (switchStateA == HIGH && brightness != 255)
{
brightness ++;
}
switchStateB = digitalRead(switchPinB);
if (switchStateB == HIGH && brightness != 0)
{
brightness --;
}
analogWrite(ledPinA, brightness); // A slow fade out
analogWrite(ledPinB, 255 - brightness); // B slow bright up
delay(20);
}
You can see in the video below, how the light “transfers” from one bulb to another as one slowly fades out. This is of course only an illusion as it corresponds to the position of the mercury.
Experimenting with the SHT31 with my Koji Board v3
To be continued…