11 - Network and Communications
Group assignment
The group assignment is documented here https://academany.fabcloud.io/fabacademy/2025/labs/barcelona/students/group-assignments/week11.html
We demonstrated:
- The ability to set up one microcontroller as a TCP server and another as a client.
- Custom protocol handling via string-based messaging.
- Sequencing and logic flow for initiating and terminating a connection.
It was really nice connecting two devices through wifi, something I will be using a lot in the future
Individual Assignment and Learnings
We have our barduino development board
https://fablabbcn-projects.gitlab.io/electronics/barduino-docs/GettingStarted/pinout/#outputs
I’ve designed a board with a Xiao esp32s3, grove connectors, DuPont connectors and screw terminal connections for the higher voltage elements.
- 12V heating mat, 12 V fan, and 5V servo motor for temperature control, heating and ventialation
- 4 Grove connectors for T/H sensor, Load Cell, Humidifier/Atomizer and LCD screen
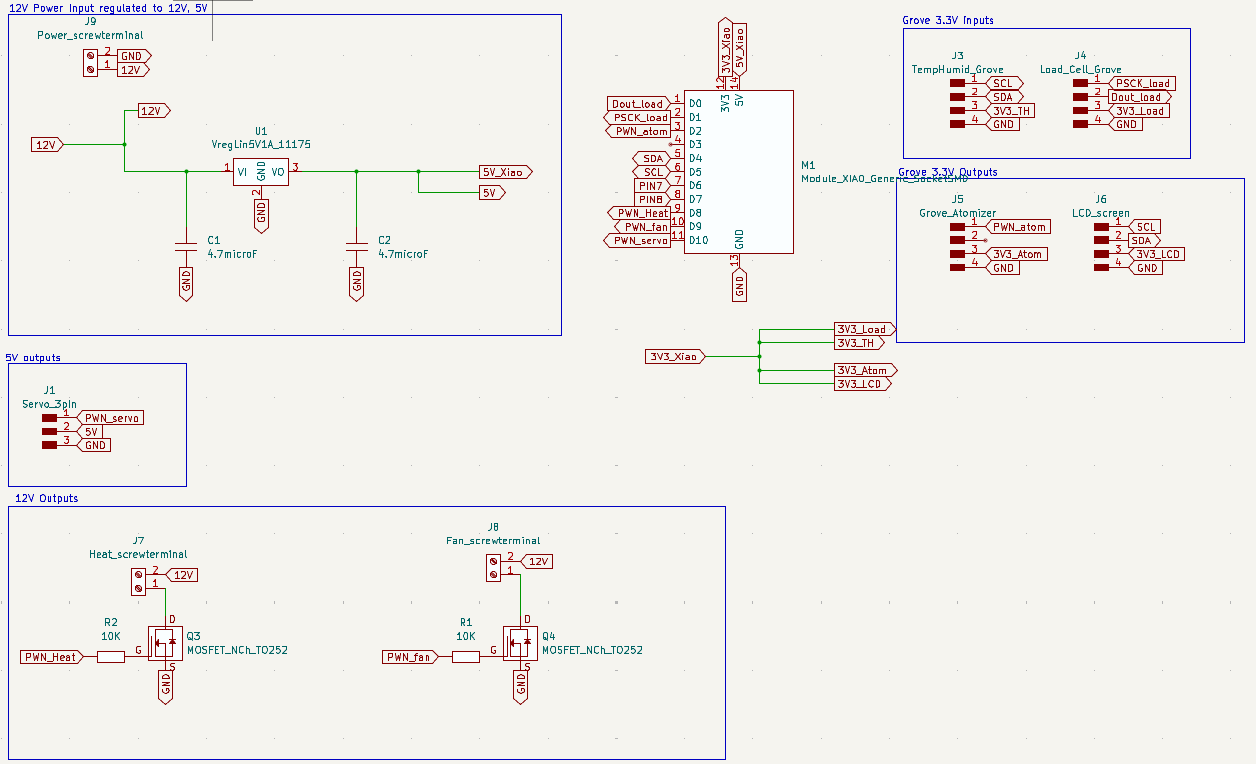
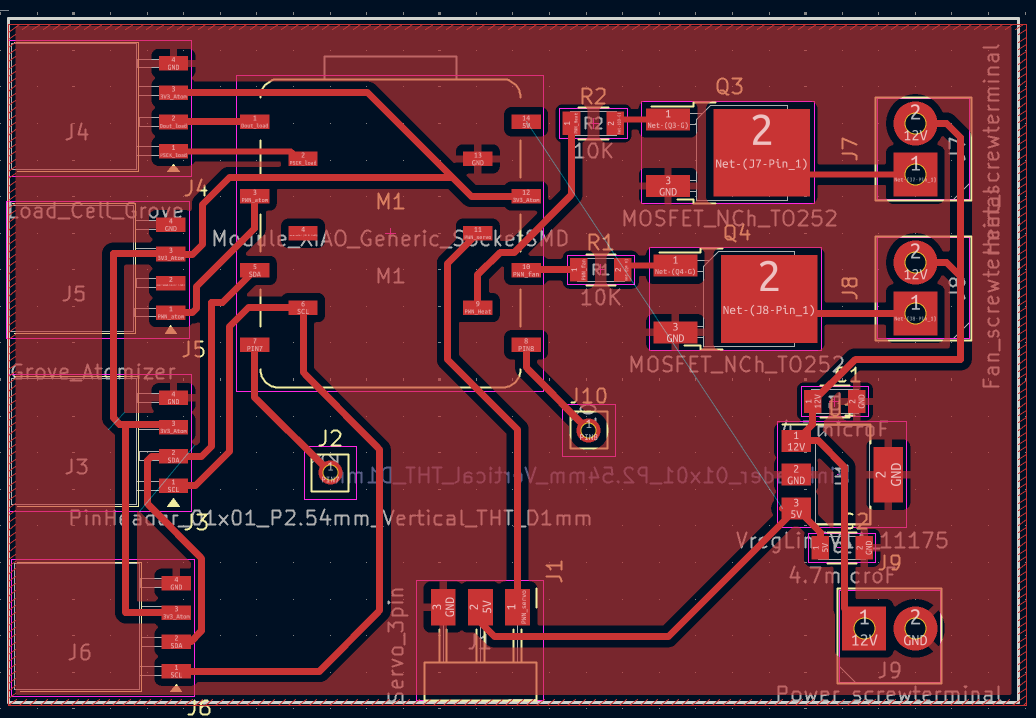
I ran into some practical errors, spacing for the grove connectors, GND islands that aren’t connected
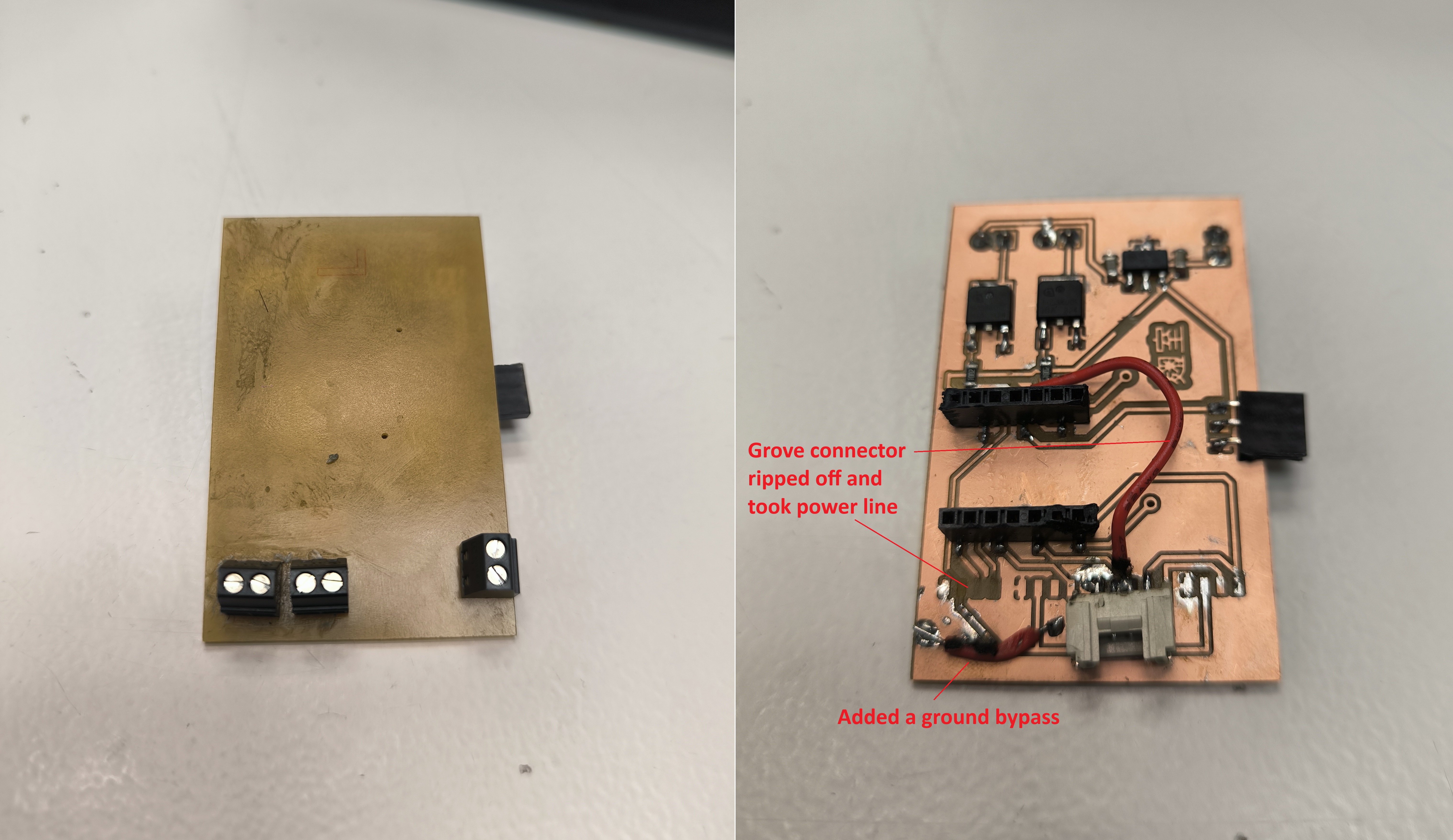
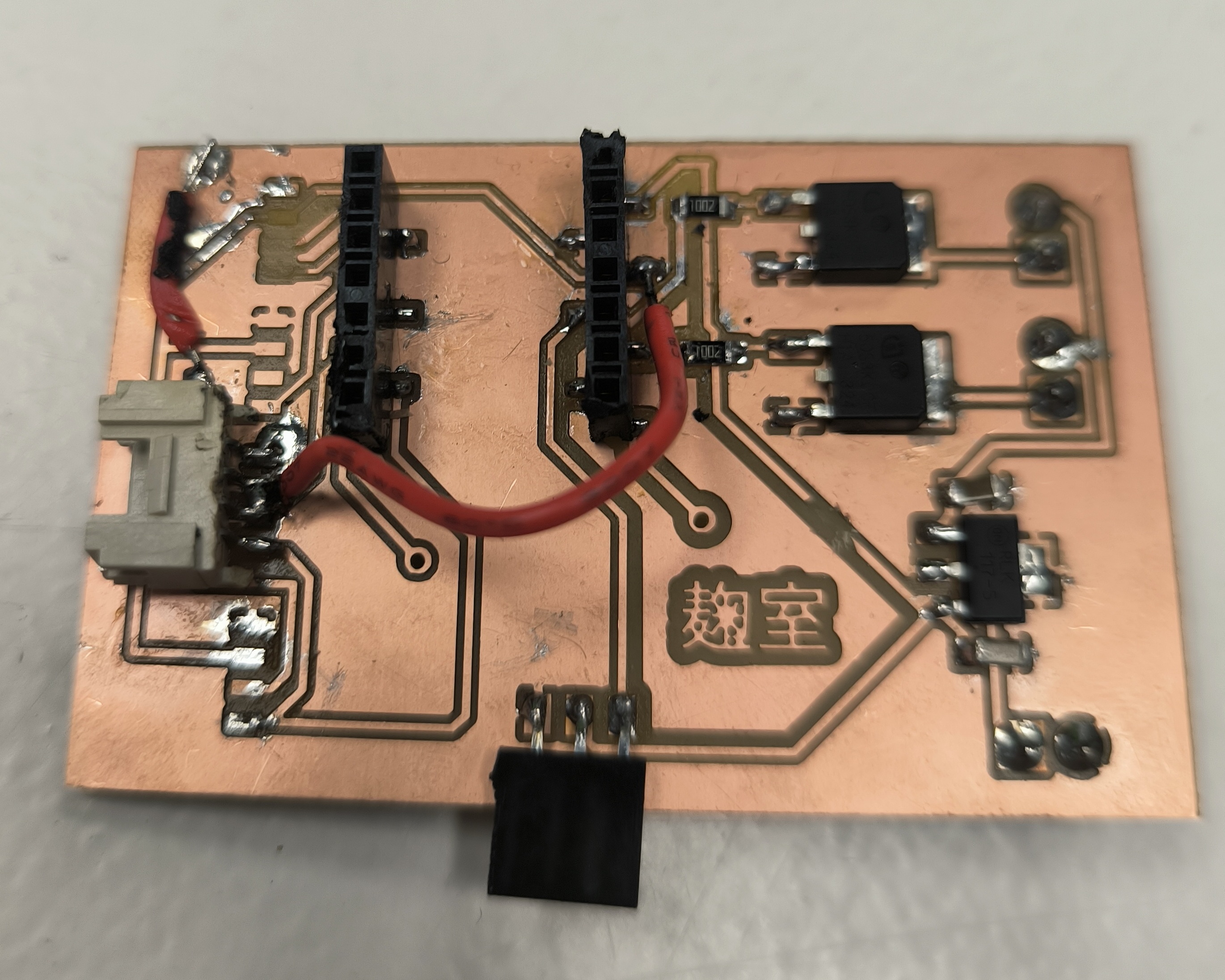
Assignment
The Grove connectors are isolated on my board with a direct connection to the Xiao. There are no pullup resistors used as the Xiao and the Seeed Studio sensors are already designed correctly. I’ll fix the board and code the Xiao and Temperature sensor so it works with the corrected board.
I connected a Xiao to both the temperature sensor and the Barduino 4.0.2 board on the I2C line
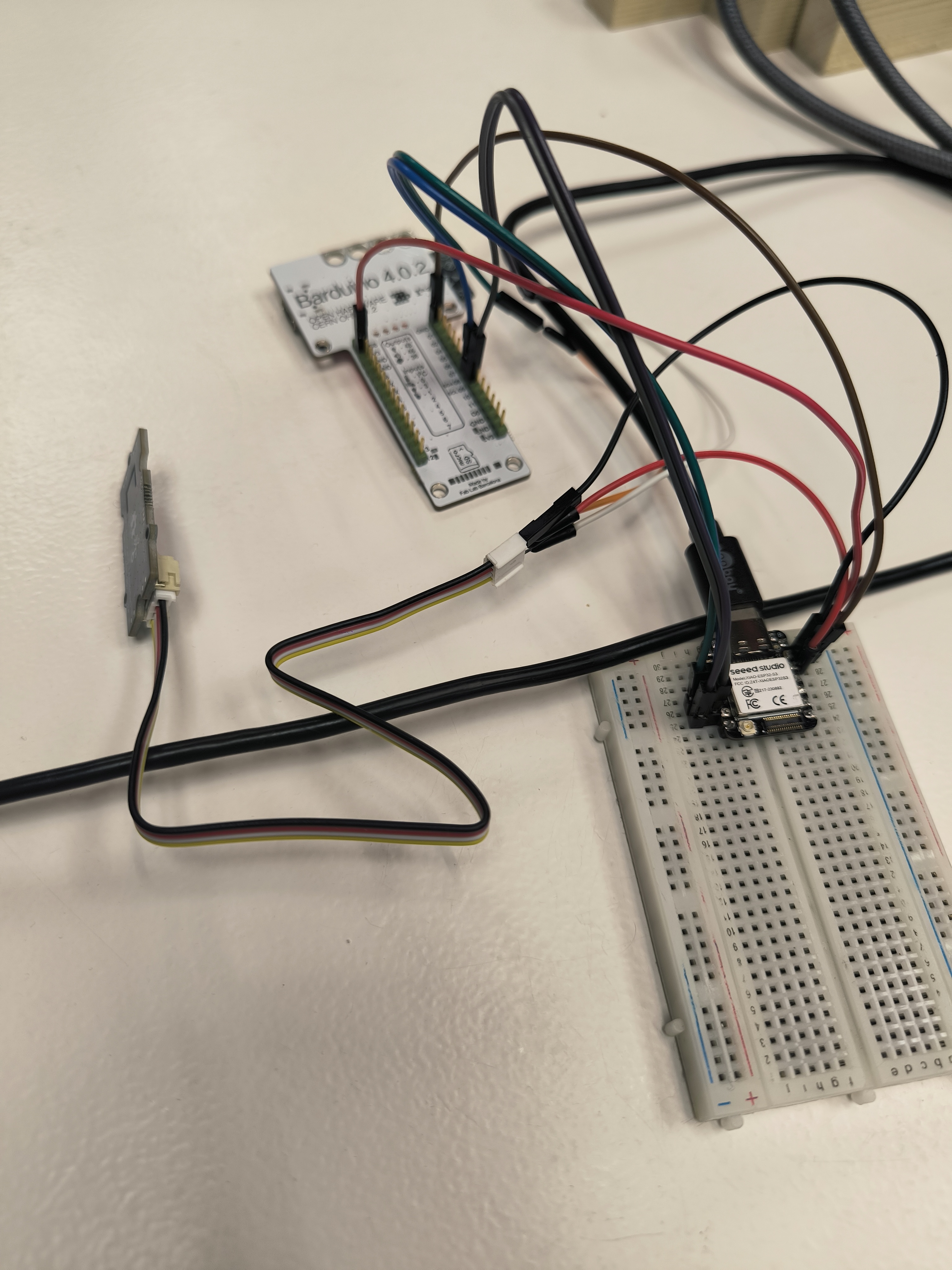
I coded the Xiao to be the main, his job is to read the temperature, send the value to the secondary Barduino board. If the temperature is above 25oC The Barduino then responds with a message saying it is very hot.
Main Xiao code:
#include <Wire.h>
#include "Seeed_SHT35.h"
// Define the I2C Slave Address of the other ESP32
const byte slaveAddress = 0x08; // Choose a unique 7-bit I2C address (1-127)
// Define SDA and SCL pins for XIAO ESP32S3
#define SDAPIN D4 // GPIO5
#define SCLPIN D5 // GPIO6
SHT35 sensor(SCLPIN); // .
void setup() {
Serial.begin(115200); //
delay(10);
Serial.println("XIAO ESP32S3 I2C Master/SHT35"); //
Wire.begin(SDAPIN, SCLPIN); // Initialize Wire with your defined pins
if (sensor.init()) {
Serial.println("sensor init failed!!"); //
} else {
Serial.println("SHT35 sensor initialized successfully."); //
}
delay(1000);
}
void loop() {
delay(2000);
float temp, hum;
if (NO_ERROR != sensor.read_meas_data_single_shot(HIGH_REP_WITH_STRCH, &temp, &hum)) {
Serial.println("Failed to read temperature from SHT35!");
} else {
Serial.print("SHT35 Temperature: ");
Serial.println(temp);
Serial.print("Sending temperature to slave (0x");
Serial.print(slaveAddress, HEX);
Serial.println(")...");
// Add a small delay before sending data
delay(100); // Adjust this delay if necessary
Wire.beginTransmission(slaveAddress);
Wire.write((byte*)&temp, sizeof(temp));
byte error = Wire.endTransmission();
if (error == 0) {
Serial.println("Temperature sent successfully.");
// Receive the reply from the slave
Wire.requestFrom(slaveAddress, 64); // Request up to 64 bytes for the reply
if (Wire.available()) {
String repy = "";
while (Wire.available()) {
char c = Wire.read();
reply += c;
}
Serial.print("Slave replied: ");
Serial.println(reply);
// Send the final reply back
String finalReply = "I'm fine thanks, disfruta!";
Wire.beginTransmission(slaveAddress);
Wire.write((const uint8_t*)finalReply.c_str(), finalReply.length()); // Cast to const uint8_t*
Wire.endTransmission();
Serial.println("Final reply sent.");
} else {
Serial.println("No reply received from slave.");
}
} else {
Serial.print("Error sending temperature, error code: ");
Serial.println(error);
}
}
}
Secondary Barduino Code
#include <Wire.h>
// I2C Slave Address - Must match the master's slaveAddress
const byte slaveAddress = 0x08;
// Define I2C pins for the Slave ESP32-S3
const int sdaPin = 9; // GPIO9
const int sclPin = 8; // GPIO8
// Buffer to store received temperature
float receivedTemperature;
byte receivedData[sizeof(float)];
// Buffer for the reply message
char replyMsg[64];
// Flag to indicate if the master is requesting a reply
bool masterIsRequesting = false;
void receiveEvent(int howMany) {
Serial.println("receiveEvent triggered");
if (howMany == sizeof(float)) {
// Read temperature data
for (int i = 0; i < howMany; i++) {
receivedData[i] = Wire.read();
}
memcpy(&receivedTemperature, receivedData, sizeof(receivedTemperature));
Serial.print("Temperature received: ");
Serial.println(receivedTemperature);
// Prepare the reply based on temperature
if (receivedTemperature > 25.0) {
strcpy(replyMsg, "My word it is hot! I could do with an ice plunge");
} else {
strcpy(replyMsg, "It's quite pleasant here, actually.");
}
masterIsRequesting = true; // Set the flag that we have a reply ready
} else if (howMany > 0) {
// Handle the final reply from the master
String receivedFinalReply = "";
while (Wire.available()) {
receivedFinalReply += (char)Wire.read();
}
Serial.print("Master's final reply: ");
Serial.println(receivedFinalReply);
} else {
Serial.println("Received unexpected number of bytes.");
}
}
void requestEvent() {
Serial.println("requestEvent triggered");
if (masterIsRequesting) {
Wire.write((uint8_t*)replyMsg, strlen(replyMsg)); // Send the prepared reply as bytes
masterIsRequesting = false; // Reset the flag
} else {
// Optionally send a default or empty response if no reply is ready
const char emptyResponse[] = "";
Wire.write((uint8_t*)emptyResponse, strlen(emptyResponse));
}
}
void setup() {
Serial.begin(115200);
Serial.println("I2C Slave/Replier");
Wire.begin(slaveAddress, sdaPin, sclPin); // Initialize Wire as slave with specified pins
Wire.onReceive(receiveEvent);
Wire.onRequest(requestEvent);
}
void loop() {
delay(100);
}
Unfortunately i’m stuck debugging, the Xiao reads the temperature but there is a problem with the transmission to the Barduino, here is the serial monitor error message.
SHT35 Temperature: 23.81
Sending temperature to slave (0x8)...
E (1156466) i2c.master: I2C transaction unexpected nack detected
E (1156466) i2c.master: s_i2c_synchronous_transaction(924): I2C transaction failed
E (1156468) i2c.master: i2c_master_multi_buffer_transmit(1186): I2C transaction failed
Error sending temperature, error code: 4
I used DSO Nano to check that there was a message being transmitted on the pins. The oscillations suggest that it is, the GND is correctly connected and the 5V from the Xiao is powering the Barduino.
The file isn’t downloading so a a temporary fix here is a link to the video:
Debugging will be finished this evening.