Week 9 Assignment
OUTPUT DEVICES
This weeks assigmenat was to test output devices using different development boards so i stared with the neo pixel led that attached with my board,and i wanted to build something related to my project i tried to build a mosfet driver that i will used to test a spark generator
Group Assignment Week 9
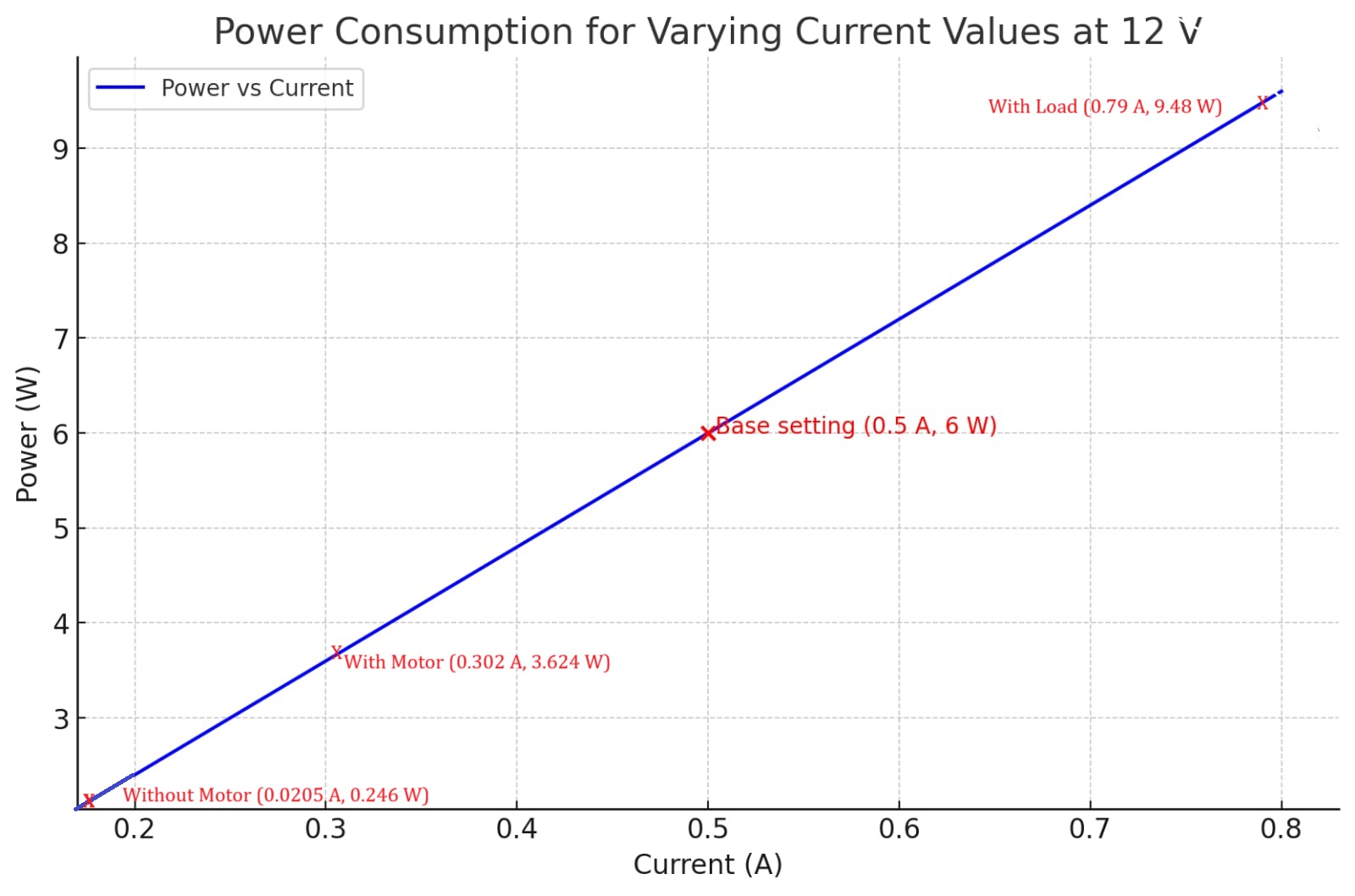
WS2812
WS2812 is a type of addressable RGB LED (Light Emitting Diode) commonly used in various lighting and display applications. These LEDs are often referred to as NeoPixels, a trademarked name by Adafruit, one of the leading manufacturers of WS2812-based products. The WS2812 LED integrates the LED die, control circuitry, and a communication interface into a single package, making it easy to control individual LEDs or groups of LEDs with a microcontroller or other digital control devices. Each WS2812 LED has a built-in shift register, which allows it to receive data serially and pass on the data to the next LED in the chain. One of the key features of WS2812 LEDs is their ability to be individually addressed, meaning that each LED in a chain can be assigned a unique color and brightness. This makes them ideal for applications such as LED matrix displays, decorative lighting, and wearable electronics. WS2812 LEDs communicate using a simple serial protocol, typically using a single data line to transmit color and brightness information to each LED in the chain. The communication protocol requires precise timing to ensure accurate data transmission, and there are various libraries and software tools available to simplify the process of controlling WS2812 LEDs with microcontrollers
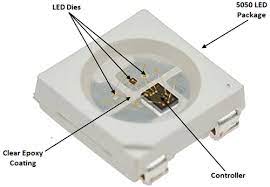

WS2812 LIGHTUP
So i used a basic programme to light up and run a animation on the single led
this is the sketch that i used for the WS2812B individual addressable led
#include Adafruit_NeoPixel.h // Pin connected to the NeoPixels (WS2812B) #define LED_PIN 15 // Number of NeoPixels #define LED_COUNT 1 // Create NeoPixel object Adafruit_NeoPixel strip(LED_COUNT, LED_PIN, NEO_GRB + NEO_KHZ800); void setup() { // Initialize NeoPixel strip strip.begin(); strip.show(); // Initialize all pixels to 'off' } void loop() { // Cycle through different colors rainbow(20); // Change colors every 20ms } // Function to cycle through rainbow colors void rainbow(uint8_t wait) { for (uint16_t i = 0; i < 256; i++) { uint32_t color = Wheel((i + strip.numPixels()) & 255); for (int j = 0; j < strip.numPixels(); j++) { strip.setPixelColor(j, color); } strip.show(); delay(wait); } } // Input a value 0 to 255 to get a color value. // The colors are a transition r - g - b - back to r. uint32_t Wheel(byte WheelPos) { WheelPos = 255 - WheelPos; if (WheelPos < 85) { return strip.Color(255 - WheelPos * 3, 0, WheelPos * 3); } if (WheelPos < 170) { WheelPos -= 85; return strip.Color(0, WheelPos * 3, 255 - WheelPos * 3); } WheelPos -= 170; return strip.Color(WheelPos * 3, 255 - WheelPos * 3, 0); }
HOW DOES THE CODE WORK
#include Adafruit_NeoPixel.h:
This line includes the Adafruit NeoPixel library, which provides functions to control WS2812B LEDs.#define LED_PIN 15:
This line defines the pin to which the data line of the WS2812B LED strip is connected. In this case, it's pin 15.#define LED_COUNT 1:
This line defines the number of LEDs in the LED strip. In this case, there is only one LED.Adafruit_NeoPixel strip(LED_COUNT, LED_PIN, NEO_GRB + NEO_KHZ800):
This line creates a NeoPixel object named strip. It initializes the object with the number of LEDs (LED_COUNT), the pin connected to the LEDs (LED_PIN), and the color order and communication speed (NEO_GRB + NEO_KHZ800). Here, NEO_GRB specifies the color order (red, green, blue), and NEO_KHZ800 specifies the communication frequency (800 KHz).void setup():
This function is called once when the Arduino board starts up. It initializes the NeoPixel strip.strip.begin():
This line initializes the NeoPixel strip.strip.show():
This line sends the initial color data to all pixels in the strip, turning them off initially.void loop():
This function is called repeatedly in a loop after the setup() function. It contains the main code logic.rainbow(20):
This line calls the rainbow() function with a delay of 20 milliseconds. This function changes the color of the LED strip in a rainbow pattern.void rainbow(uint8_t wait):
This function defines the rainbow effect. It cycles through different colors of the rainbow with a delay specified by the wait parameter.for (uint16_t i = 0; i < 256; i++) { ... }:
This loop iterates through 256 color values to create the rainbow effect.uint32_t color = Wheel((i + strip.numPixels()) & 255):
This line calculates the color for the current position in the rainbow using the Wheel() function.strip.setPixelColor(j, color):
This line sets the color of each pixel in the strip to the calculated color.strip.show():
This line updates the LED strip with the new color data.delay(wait):
This line pauses the execution for the specified delay time.uint32_t Wheel(byte WheelPos):
This function calculates the color for a given position in the rainbow. It takes a value between 0 and 255 and returns a corresponding color value. The colors transition from red to green to blue and back to red.