Week 15: Interface &
Application Programming
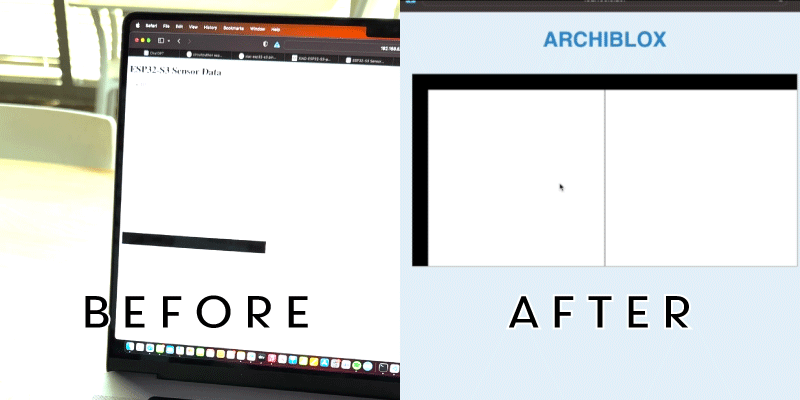
A More Aesthetic Interface
Currently, the web interace is a plain white background with a generic heading "ESP32-S3 Sensor Data" Along with a text area that populates with which sensors are being triggered along with the appropriate image of the floorplan displayed below.
While the vision for the web app in the long run will have lots of additional functionality, for this first prototype, I am focused on just a simple "blueprint" environment that populates the floorplan tiles on the screen.
Style Changes To The Web Interface:
The first thing I eliminated is the sensor data - while that was important in the development phase for me to see, I don't want the end user aware of how it magically knows which tile is which. So that information was no longer made visible on the web app- but we can still see it in the console on Thonny when the device is plugged in to the laptop.
The next thing was changing the header text to just read "ARCHIBLOX" and centering it on the page. Once again, the end user doesn't need to know what microprocessor is generating these images, I want them to associate the magic with the name of the product.
The last edit was to change the color of the background and of the text to look like a blueprint. A light blue that resembles the distinctive color of real blueprints that was created by the photosenstitive solution of the ammonium ferric citrate and potassium ferricyanide.
The result is a very simple and clean interface that puts all of the focus on the 2D floorplan. The coding was all done in Visual Studio Code with assistance from ChatGPT.
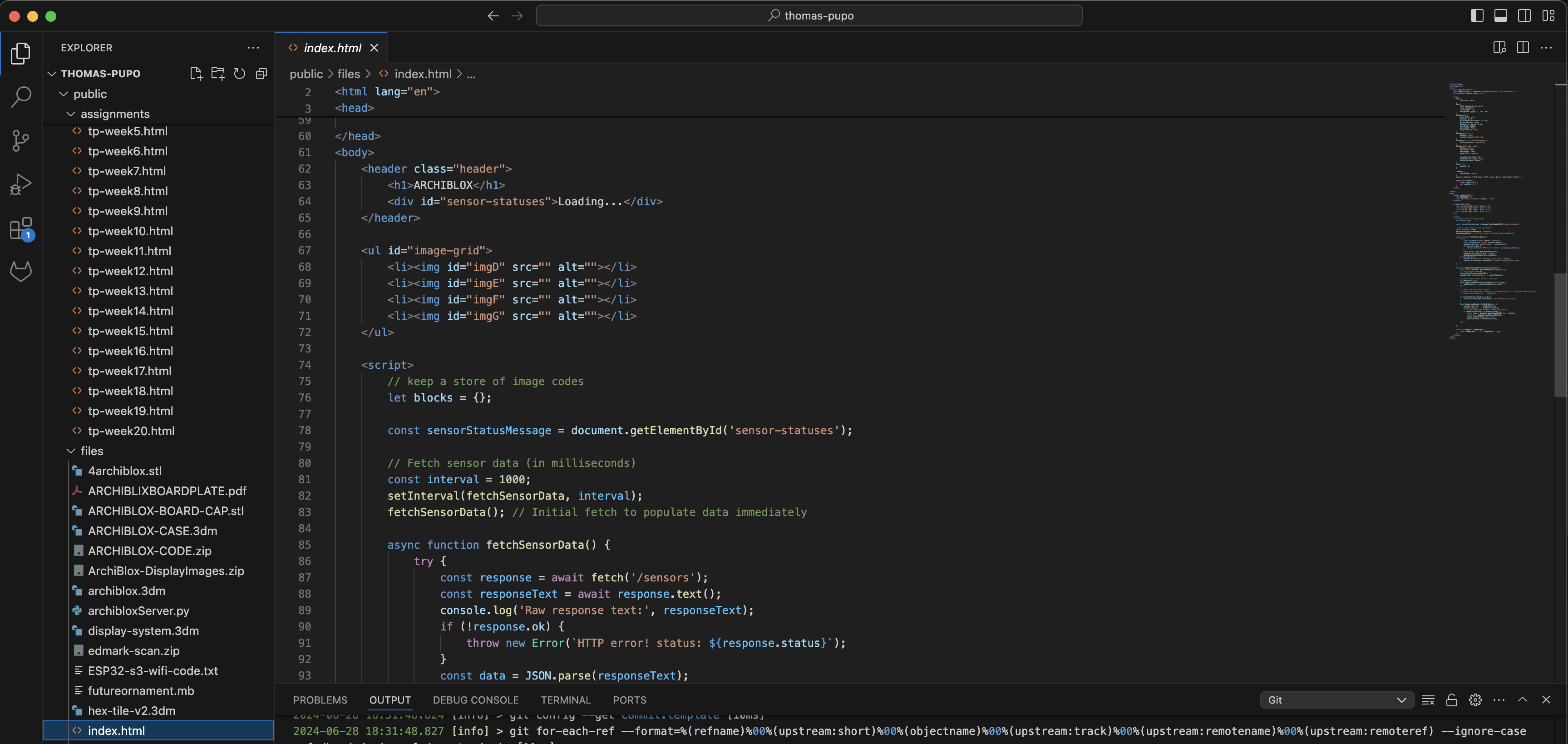
Setup and Initialization:
Python Backend (archiBloxServer.py):
This Python script runs on the ESP32-S3 microcontroller and sets up a web server to handle HTTP requests.
It reads data from hall sensors connected to the microcontroller and serves this data as JSON via an endpoint (e.g., /sensors).
HTML Frontend (index.html):
The HTML file sets up the structure and styling of the webpage, which is hosted on the microcontroller.
The 'style' defines the aesthetics, making the page look clean and easy to read, resembling a floor plan due to the use of colors and vector images.
Fetching Sensor Data
The JavaScript code in the HTML file uses the fetchSensorData function to periodically (every second) request sensor data from the /sensors endpoint.
When the data is fetched, it is parsed from JSON format and passed to the displaySensorStatuses function for updating the webpage.
Processing and Displaying Data:
Processing:
The fetchSensorData function sends an HTTP GET request to the /sensors endpoint.
If the request is successful, the response is converted from text to a JSON object.
This JSON object contains sensor statuses which are then processed to update the images on the webpage.
Displaying:
The displaySensorStatuses function maps sensor statuses to corresponding images.
Each sensor status is converted to an image filename (e.g., d1234.png), which is then set as the src attribute of an 'img' element.
If the sensor status changes, the corresponding image is updated to reflect this change.
Aesthetic Considerations:
The webpage uses a clean and simple design with a sans-serif font and a light blue background color (rgb(227, 242, 250)), which makes the content legible and visually appealing.
The #image-grid element displays images in a grid layout, with each image representing data from a different sensor. This layout helps in visualizing sensor data as a floor plan.
The use of animations (e.g., fadeIn) makes the updates smooth and visually appealing.
JSON Usage:
The sensor data is transmitted in JSON format, which is a lightweight data-interchange format.
The fetchSensorData function parses this JSON data to extract sensor statuses.
The imageSrc function constructs the image filename based on the sensor status and updates the src attribute of the corresponding 'img' element.
File Correspondence:
The filenames used in the imageSrc function correspond to sensor data. For instance, if a sensor status is 1234, the corresponding image file would be d1234.png.
This mapping allows the webpage to dynamically display the correct image based on the current sensor status.
Key Elements of my Communications and Interface Strategy:
Backend Server: Handles sensor data collection and serves it via an HTTP endpoint.
Frontend Interface: Fetches and displays sensor data using a clean and responsive design.
JSON Data: Used for transmitting sensor statuses from the server to the client.
Dynamic Image Updating: Images are updated dynamically based on sensor statuses, providing a real-time visual representation of the sensor data.
This combination of a Python backend, HTML/CSS for styling, and JavaScript for dynamic updates creates an efficient and user-friendly interface for monitoring sensor data in real time. The choice of colors, grid layout, and vector images enhances readability and makes the interface resemble a floor plan, which is particularly useful for applications in architecture and design.
Interface Demo
Files
Download Web Interface HTML Code
Download ESP32S3 Updated Python Code
Group Assignment
This week, we were asked to look at different tools and programs we could use as interfaces for our projects. Over time, we've used various tools like Processing for our Machine Design week. At the same time, I was working on an HTML web app for my 'Archiblox' project, so we thought it'd be cool to compare the two.
I did some research using ChatGPT to dig into the differences and similarities that weren't immediately obvious. Here's what I found:
Processing is awesome for visual arts and creative coding. It's perfect for projects that need interactive graphics, visualizations, or digital art. It runs in the Processing Development Environment (PDE) and uses a language based on Java, with an option to code in Python too. Processing is great for creating graphics and animations because it has tons of built-in functions just for that. It's also super beginner-friendly, especially for those new to visual arts and programming. Plus, you can run applications made in Processing as standalone programs.
On the other hand, we're pretty familiar with HTML since we've used it to create websites. HTML is a markup language that structures web content and is usually paired with CSS for styling and JavaScript for adding interactivity. Unlike Processing, HTML doesn't directly handle interactivity—it needs JavaScript for that. HTML projects run in web browsers, which makes them perfect for web apps. While HTML can handle graphics and animations with the canvas element and CSS, more complex stuff usually needs extra JavaScript libraries like p5.js.
Both Processing and HTML (with JavaScript) allow you to create interactive user interfaces where users can click, type, and interact with visual elements. They both support 2D and 3D graphics—Processing has these features built-in, while HTML uses things like WebGL and libraries like Three.js for 3D. They can handle events like mouse clicks and key presses; Processing has built-in functions for this, and HTML uses JavaScript. Both are open-source and have a lot of community support and plenty of libraries and tools available. They're also popular in education for teaching programming, graphics, and interactive design.
A Deeper Dive:
Processing
What it is and what it's good for:
Processing is a flexible software sketchbook and a language for learning how to code within the context of the visual arts. It's tailored for creative projects like interactive graphics, visualizations, and digital art.
Development Environment:
It runs in the Processing Development Environment (PDE), which is straightforward and beginner-friendly. PDE makes it easy to start coding right away without needing to set up a lot of complex configurations.
Language and Libraries:
Processing uses a language based on Java, but there's also an option to code in Python (with the Processing.py mode). The real power of Processing comes from its extensive libraries designed for graphics and animation. For example, you have built-in functions for drawing shapes, handling images, and creating animations. This means you don't have to start from scratch when you want to create visual elements.
Accessibility:
Processing is particularly beginner-friendly, making it accessible for people new to programming or the visual arts. It abstracts a lot of the complex details of programming, allowing users to focus on creating their art or visualization.
Standalone Applications:
Applications made in Processing can be exported as standalone programs. This means you can share your work with others who don't have Processing installed, making it easy to distribute your projects.
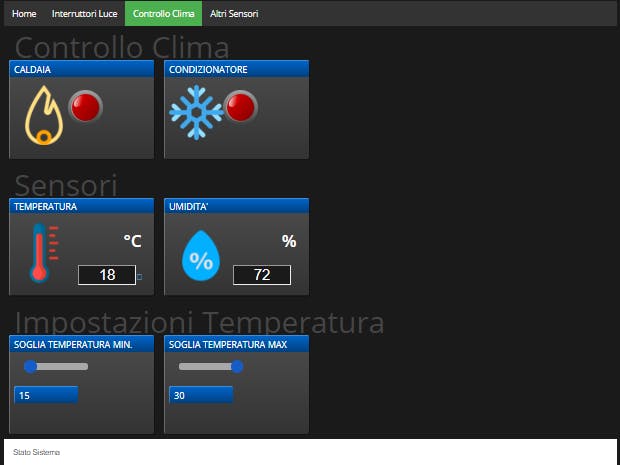
HTML
What it is and what it's good for:
HTML (HyperText Markup Language) is used to structure web content. It's the backbone of all web pages and works together with CSS (Cascading Style Sheets) for styling and JavaScript for interactivity.
Structuring Content:
HTML is excellent at defining the structure of web pages. You can use it to create headings, paragraphs, links, images, and more. However, on its own, HTML doesn't provide interactivity beyond basic links.
Adding Interactivity:
For interactivity, you need JavaScript. JavaScript can manipulate the HTML structure and style in real-time, handle user input, and create dynamic web experiences.
Graphics and Animations:
While HTML itself isn't designed for graphics, it can use the 'canvas' element for 2D graphics, which you can draw on with JavaScript. For more complex graphics and animations, you typically use libraries like p5.js (which is inspired by Processing) or Three.js for 3D graphics. These libraries provide more advanced functions and capabilities, similar to the built-in functions in Processing.
Running in Browsers:
HTML projects run in web browsers, making them ideal for creating web applications. This is great because your project can be accessed by anyone with a browser, without needing to install any additional software. This wide accessibility is a huge advantage for web-based projects.
Community and Support:
Both Processing and HTML have strong community support. Processing has a vibrant community of artists and creative coders who share their work and help each other out. HTML, along with CSS and JavaScript, has one of the largest developer communities, with countless resources, tutorials, and libraries available online.
Comparing Processing and HTML
Interactive Interfaces:
Both tools can create interactive user interfaces. Processing has built-in functions to handle user input like mouse clicks and key presses, which makes it very straightforward to create interactive applications. HTML relies on JavaScript to handle these interactions, which gives you a lot of flexibility but requires a bit more setup.
Graphics Capabilities:
Processing excels in 2D and 3D graphics with a straightforward API designed for these tasks. HTML can also handle graphics through the canvas element for 2D and libraries like WebGL and Three.js for 3D, but these require additional JavaScript code and libraries.
Usage and Accessibility:
Processing is great for standalone applications and creative projects, particularly in the arts and education. HTML, being the foundation of web content, is perfect for web applications that need to be easily accessible via web browsers.
Community and Resources:
Both have extensive resources available, but the nature of these resources differs. Processing's resources are often focused on creative coding and visual arts, while HTML's resources are broader, covering everything from basic web development to complex web applications.
Copyright 2024 Thomas Pupo - Creative Commons Attribution Non Commercial
Source code hosted at fabcloud/fabacademy/2024/thomas-pupo