class: center, middle # Intro to Programming A Fab Academy Recitation by [Rodrigo Shiordia](https://www.instagram.com/rshiordia). --- # Overview * What is programming? * Code vs. Program vs. Algorithm * What does a program do? * The building blocks of programming. - Variables - Control Structures - Functions and Methods - Debugging - Libraries --- # What Is programming? * Programming is a way for us to tell computers what to do. * A computer is very fast, but also very dumb. * Computer programming is like giving instructions to someone, but you have to be very literal and describe everything in precise detail. The computer is incapable of understanding what you want, so you have to be very precise. --- # Coding vs. Programming * Coding is normally used as a synonym to programming. You code in python, C or C++ which are programming languages. * Programming is the logic behind the code. It refers to coming up with the ideas that you need to order in a code so that the computer understands and executes your intent. --- # How does a program work? * In the context of Fab Academy, we will use programs to tell MCU's to deal with inputs, outputs, and talk to other devices. * A program is written in source code. * Source code is compiled by a compiler into machine code. * Machine code is executed by the computer and we get a specific computing result. --- # The building blocks of programming. * Variables * Control Structures * Functions and Methods * Debugging * Libraries --- # Variables * A variable is a pointer to some memory location. Whe give it a name and store data to it. - variable data type - variable name - variable value 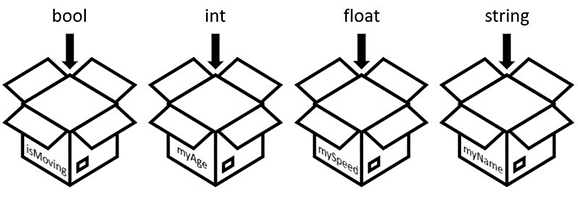 --- # Data Types * Data types are the "categories" that variables belong to. 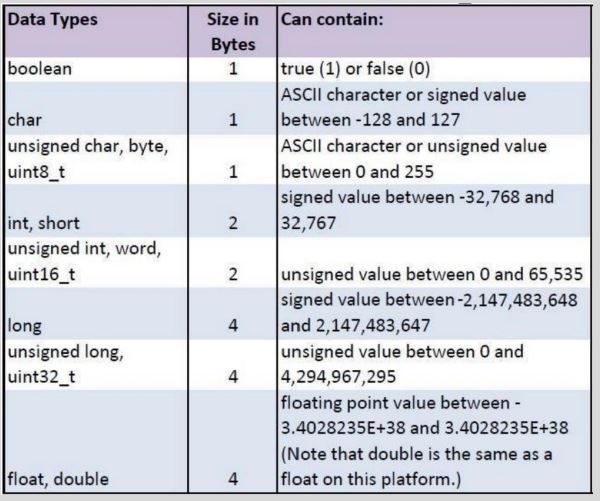 --- # Using Variables ```Arduino //Explaining Variables //Rodrigo Shiordia 2023 int myAge = 37; float mySpeed = 1.5; bool isAlive = true; String myName = "Rodrigo"; void setup() { // put your setup code here, to run once: Serial.begin(9600); } void loop() { // put your main code here, to run repeatedly: Serial.println(myName + " is " + myAge + " years old."); delay(2000); } ``` --- # Flow Control * Control loops help us manage the Flow a program. * Flow control is about making decisions of when to do things or how many times to do different things. * Using Flow control structures we can create more complex programs that can repeat code several times, check if a condition is met, do somehting in case something else happens, or read iteratively from a list, etc. * Common control structures are: * Conditionals * For loops * While Loops --- # Conditionals - If/Else ```Arduino //Explaining Conditionals //Rodrigo Shiordia 2023 int myAge = 37; float mySpeed = 1.5; bool isAlive = true; String myName = "Rodrigo"; void setup() { Serial.begin(9600); } void loop() { if (myAge < 37) { Serial.println(myName + " is young."); delay(2000); } else if (myAge == 37) { Serial.println(myName + " is old."); delay(2000); } else { Serial.println(myName + " is veeery old."); delay(2000); } } ``` --- # Iteration - For loops ```Arduino //Explaining For Loops //Rodrigo Shiordia 2023 int assignmentsDone = 0; void setup() { Serial.begin(9600); } void loop() { for (int weeks = 0; weeks < 15; weeks++) { assignmentsDone++; Serial.println("assignments completed: " + String(assignmentsDone)); delay(2000); } } ``` --- # Iteration - While loops ```Arduino //Explaining While Loops //Rodrigo Shiordia 2023 boolean working = true; int tiredLevel = 0; void setup() { Serial.begin(9600); } void loop() { while (working == 1) { Serial.println("I am working on my Fab Academy project!"); tiredLevel++; delay(1000); if (tiredLevel>15){ working=false; Serial.println("I am going to sleep!"); } } } ``` --- # Iteration - Do...While ```Arduino //Explaining Do...While Loops //Rodrigo Shiordia 2023 int tiredLevel = 0; void setup() { Serial.begin(9600); } void loop() { do{ Serial.println("getting some work done..."); tiredLevel++; delay(2000); } while(tiredLevel < 10); Serial.println("done working"); } ``` --- # Functions and methods * Functions and methods are ways of reusing code. * Something that you are going to do several times, you can put inside a function and use it again and again. * A function normally has a return value: somehting that it returns back to you after you call or invoke it. (Some functions have no return value or a void return value.) * It's important to distinguish between Built-in functions and custom functions. --- # Functions: return value ```Arduino //Explaining Functions and methods //Rodrigo Shiordia 2023 void setup() { Serial.begin(9600); Serial.println(" Temperature Calculator "); } void loop() { Serial.print("Please enter temperature in Fahrenheit: "); delay(1000); while (Serial.available() == 0) { } float F = Serial.parseFloat(); float C = fahrenheitToCelsius(F); Serial.println("Temperature in Celsius: "); Serial.println(C); delay(1000); } float fahrenheitToCelsius(float theValue) { return (theValue -32)*0.5556; } ``` --- # Functions: void return ```Arduino //Explaining Functions and methods //Rodrigo Shiordia 2023 int ledPin = 1; void setup() { Serial.begin(9600); Serial.println(" Function based LED blinker! "); } void loop() { Serial.print("How Many times you want the LED to blink: "); while (Serial.available() == 0) { } int T = Serial.parseInt(); blinkLED(T); } void blinkLED(int times) { for (int i = 0; i < times; i++) { digitalWrite(ledPin, HIGH); delay(1000); digitalWrite(ledPin, LOW); delay(1000); } } ``` --- # Debugging * Debugging is about getting your code to do what you want. * Syntax errors are when your code is written incorrectly according to the programming language. In C/Arduino, the most common is missing a semicolon or a bracket. * Use checks and print statements to know exaclty what your code is doing at any time. --- # Libraries * Arduino has libraries that extend its capabilities. * Libraries are collections of code that you can use. They normally provide you with functions and methods for you to use. --- # Tips for writing programs * Always start with some idea of what you want to do. * Write pseudocode. * Write small blocks of code and run and upload so you know it's working before writing more code. * Read as much code as you can. Writing code requires you to understand code. * Use online resources: * [stackOverflow](https://stackoverflow.com/) * [Arduino Forums](https://forum.arduino.cc/) --- # Difference between languages * C is compiled to opcodes. * Python is interpreted which means that there's an intermediate step. * Javascript is just in time compiling. --- # Thank you! ---