Interface and application programming
Group assignment:
- Compare as many tool options as possible.
- Document your work on the group work page and reflect on your individual page what you learned.
Individual assignment:
- Write an application that interfaces a user with input and/or output device(s) on a board that you made.
Group Assignment
Processing
Processing is a flexible software sketchbook and a language for learning how to code within the context of the visual arts. It is an open-source language based on Java, created to teach artists, designers and researchers how to code and produce visualizations. Processing is designed to be a simple and easy-to-learn platform for coding, graphics and interactivity.
Below is a simple project done with Arduino board and Processing, which the board reads a infrered distance sensor and sends the value through Serial port to the computer. Computer then reads the values from Serial port and display and animation base on the reading it gets using the Processing program.
Arduino Code
#define pin A0
void setup() {
Serial.begin(9600);
pinMode(pin, INPUT);
}
void loop() {
uint16_t value = analogRead(pin);
uint16_t distance = get_gp2d12(value);
Serial.write(distance);
//Serial.println ();
delay(200);
}
//return distance (mm)
uint16_t get_gp2d12(uint16_t value) {
if (value < 30)
value = 30;
return ((67870.0 / (value - 3.0)) - 40.0);
}
Processing Code
import processing.serial.*;
Serial serial;
int distance;
//int unit = 20;
int count;
Module[] mods;
void setup() {
size(2000,1500);
serial = new Serial(this, "COM3", 9600);
}
void draw() {
background(0);
if ( serial.available() > 0) {
// 读取从串口产过来的Sensor数值。
distance = serial.read();}
if(distance==0){
distance = 1;
}
int unit = distance;
noStroke();
int wideCount = width / unit;
int highCount = height / unit;
count = wideCount * highCount;
mods = new Module[count];
int index = 0;
for (int y = 0; y < highCount; y++) {
for (int x = 0; x < wideCount; x++) {
mods[index++] = new Module(x*unit, y*unit, unit/2, unit/2, random(0.05, 0.8), unit);
}
}
for (Module mod : mods) {
mod.update();
mod.display();
}
}
class Module {
int xOffset;
int yOffset;
float x, y;
int unit;
int xDirection = 1;
int yDirection = 1;
float speed;
// Contructor
Module(int xOffsetTemp, int yOffsetTemp, int xTemp, int yTemp, float speedTemp, int tempUnit) {
xOffset = xOffsetTemp;
yOffset = yOffsetTemp;
x = xTemp;
y = yTemp;
speed = speedTemp;
unit = tempUnit;
}
// Custom method for updating the variables
void update() {
x = x + (speed * xDirection);
if (x >= unit || x <= 0) {
xDirection *= -1;
x = x + (1 * xDirection);
y = y + (1 * yDirection);
}
if (y >= unit || y <= 0) {
yDirection *= -1;
y = y + (1 * yDirection);
}
}
// Custom method for drawing the object
void display() {
fill(255);
ellipse(xOffset + x, yOffset + y, 6, 6);
}
}
Comparison with other tools
p5.js
p5.js is a JavaScript library based on Processing, highly focused on accessibility and interactivity within a browser environment. Like Processing, p5.js is designed to make coding accessible for artists, designers, educators and beginners.
- Advantages: As a JavaScript library, p5.js runs within a web browser, making it more accessible and convenient for sharing projects online. It also supports easy integration with other web technologies like HTML and CSS.
- Disadvantages: Due to being JavaScript-based, p5.js might encounter performance issues compared to Processing when handling complex computational tasks or large-scale projects.
Below is a program that sends camera data to the computer via WiFi by using a ESP32 development board. Because the code size is huge, so we don't just paste the code on here. With the close intergration of p5.js and the web platform, it's easier to achieve these kind of things with it.
This is an interface to control the walking robot which I designed for my cat. The left frame is the canvas for the camera, and right is 4 bottons to control move the robot.
openFrameworks
openFrameworks is an open-source C++ toolkit designed for creative coding, aiming to provide a platform for experiments in interaction design, computer vision, and graphics. openFrameworks is highly extensible, providing great flexibility for demanding projects.
- Advantages: openFrameworks offers native C++ performance, providing significantly faster execution than Processing and p5.js. It also supports a wide range of hardware and software integrations.
- Disadvantages: The learning curve for openFrameworks might be steeper than Processing or p5.js, especially for beginners.
Cinder
Cinder is a C++ library for creative coding and the development of professional-quality applications in fields such as gaming, interactive installations, and data visualization. Cinder provides powerful performance and extensive flexibility to create high-quality projects.
- Advantages: Cinder offers native C++ performance, robust API and support for diverse hardware and software integrations. It's tailored for professionals and demanding projects.
- Disadvantages: Cinder might not be beginner-friendly and has a steeper learning curve compared to Processing and p5.js.
Conclusion
Processing, p5.js, openFrameworks, and Cinder are all powerful tools for creating interactive applications and visualizations. While Processing and p5.js are more focused on accessibility and simplicity, openFrameworks and Cinder prioritize performance and flexibility. Choosing the right tool depends on your project's goals, required performance, and coding expertise.
Individual assignment
I did a little project which is a small animation on processign, a cube streching. And when you click it with left and right botton, it will shows Keep going! and Don't stop! I'm using P3d to creat a cube on processing ,and mousePressed funtion to interact.
Notice that if you have window you need to chekc on which port your arduino is connecting, it's usually COM1 COM2 ect.
Processing Code
import processing.serial.*;
float largeCubeSize = 150; // Size of the large cube
float smallCubeSize = 50; // Size of the small cube
float cubeSize; // Current size of the cube
float cubeX, cubeY, cubeZ; // Position of the cube
boolean changeSize = false; // Flag to indicate whether to change the cube size
Serial arduinoPort; // Serial port object
void setup() {
size(600, 600, P3D); // Use 3D mode
background(255, 255, 0); // Yellow background
cubeX = width / 2; // Initial X position of the cube
cubeY = height / 2; // Initial Y position of the cube
cubeZ = 0; // Initial Z position of the cube
cubeSize = largeCubeSize; // Initial size is set to the large cube
// Initialize serial communication
arduinoPort = new Serial(this, "/dev/cu.usbmodem144201", 9600);
}
void draw() {
background(255, 255, 0); // Refresh the yellow background
// Draw the black cube
stroke(0); // Black
noFill();
// Rotate the drawing matrix to create a perspective effect
float rotateAngle = map(mouseX, 0, width, -QUARTER_PI, QUARTER_PI);
translate(cubeX, cubeY, cubeZ);
rotateY(rotateAngle);
box(cubeSize);
// Change the size of the cube based on the flag
if (changeSize) {
if (mouseButton == LEFT) {
cubeSize = largeCubeSize; // Show the large cube on left click
arduinoPort.write('H'); // Send the character 'H' to Arduino
} else if (mouseButton == RIGHT) {
cubeSize = smallCubeSize; // Show the small cube on right click
arduinoPort.write('L'); // Send the character 'L' to Arduino
}
changeSize = false; // Reset the flag
}
}
void mousePressed() {
changeSize = true; // Set the flag to change the cube size in draw()
}
Arduino Code
#include
#include
#include
#define SCREEN_WIDTH 128
#define SCREEN_HEIGHT 64
Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, -1);
void setup() {
Serial.begin(9600);
if(!display.begin(SSD1306_I2C_ADDRESS, 4, 5)) {
Serial.println(F("SSD1306 allocation failed"));
for(;;);
}
display.display(); // Display initialization
delay(2000); // Pause for 2 seconds
display.clearDisplay(); // Clear the display
}
void loop() {
if (Serial.available() > 0) {
char receivedChar = Serial.read();
if (receivedChar == 'H') {
display.clearDisplay();
display.setTextSize(1);
display.setTextColor(SSD1306_WHITE);
display.setCursor(0,0);
display.println("Keep going!");
display.display();
}
else if (receivedChar == 'L') {
display.clearDisplay();
display.setTextSize(1);
display.setTextColor(SSD1306_WHITE);
display.setCursor(0,0);
display.println("Don't Stop!");
display.display();
}
}
}
- I use HC-SR04 ultrasonic sensor to test, it is a very popular sensor for rough distance measurement.
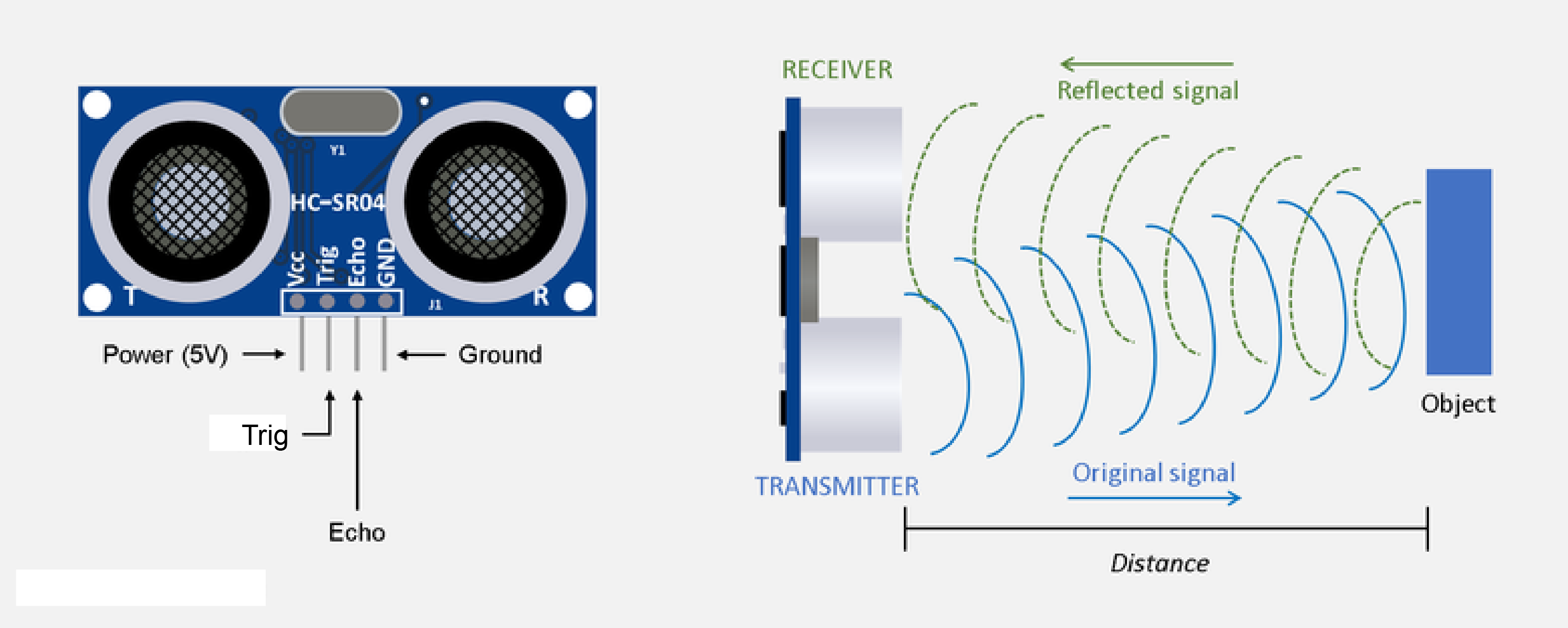
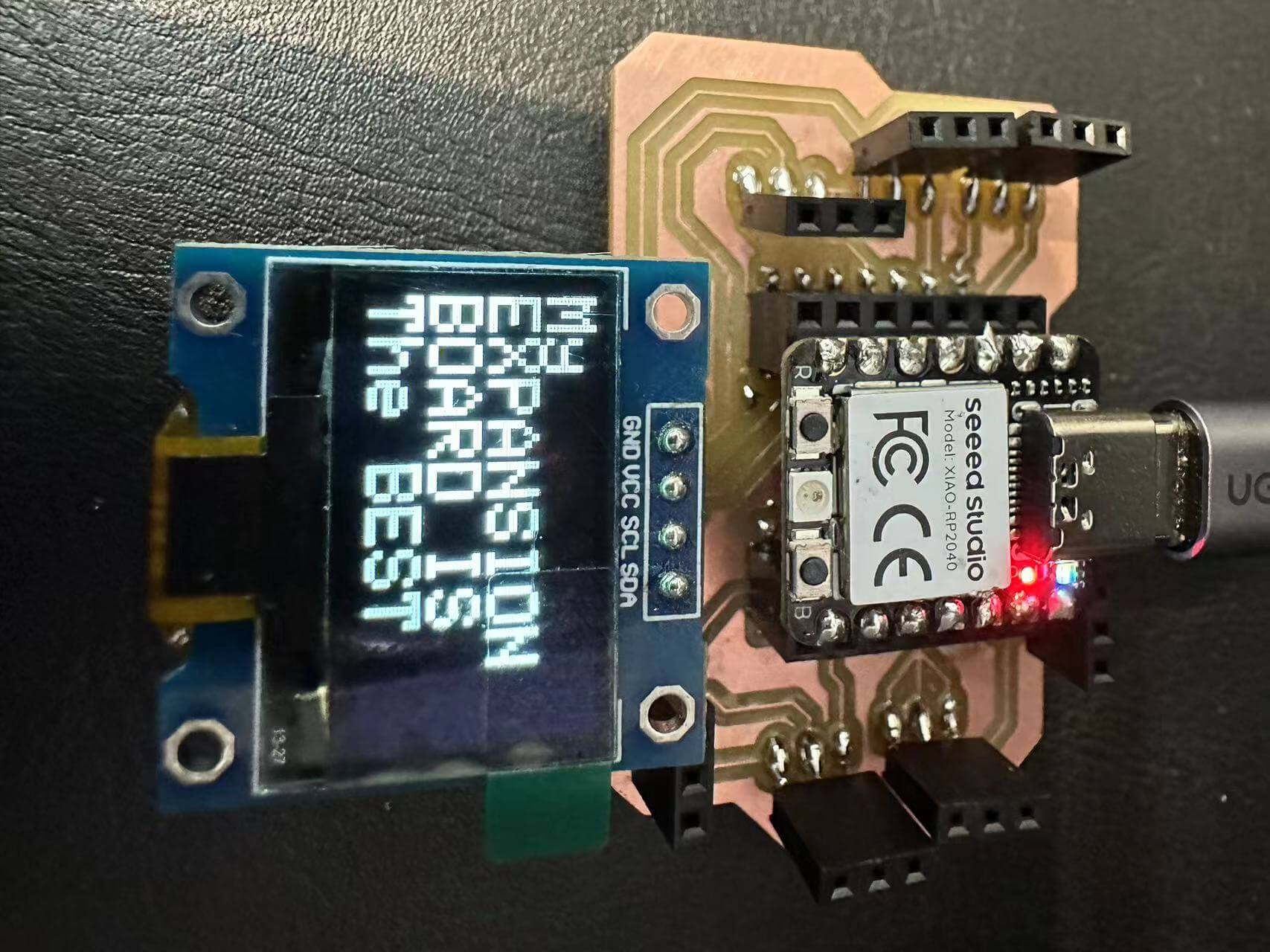
The test I did the week when I designed xiao expension board.
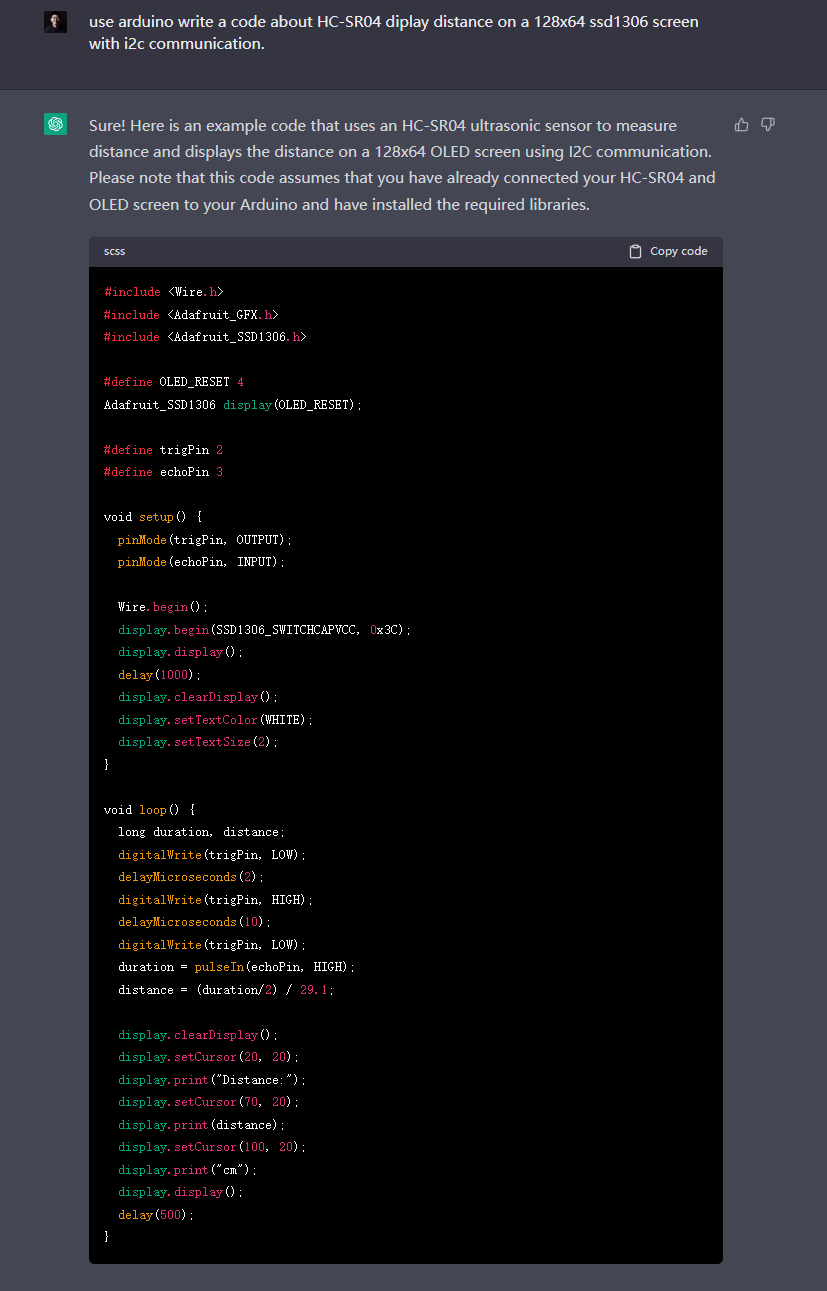