Interface and application programming
Group Assignment
compare as many tool options as possible
Individual assignment
write an application that interfaces a user with an input &/or output device that you made
Group Assignment
Link for our group Assignment
Individual Assignment
This week assignment is to write an application to interface with the input/output board i have designed.
Processing
I have linked a wikipedia page to understand better on processing
Here is a link to download processing
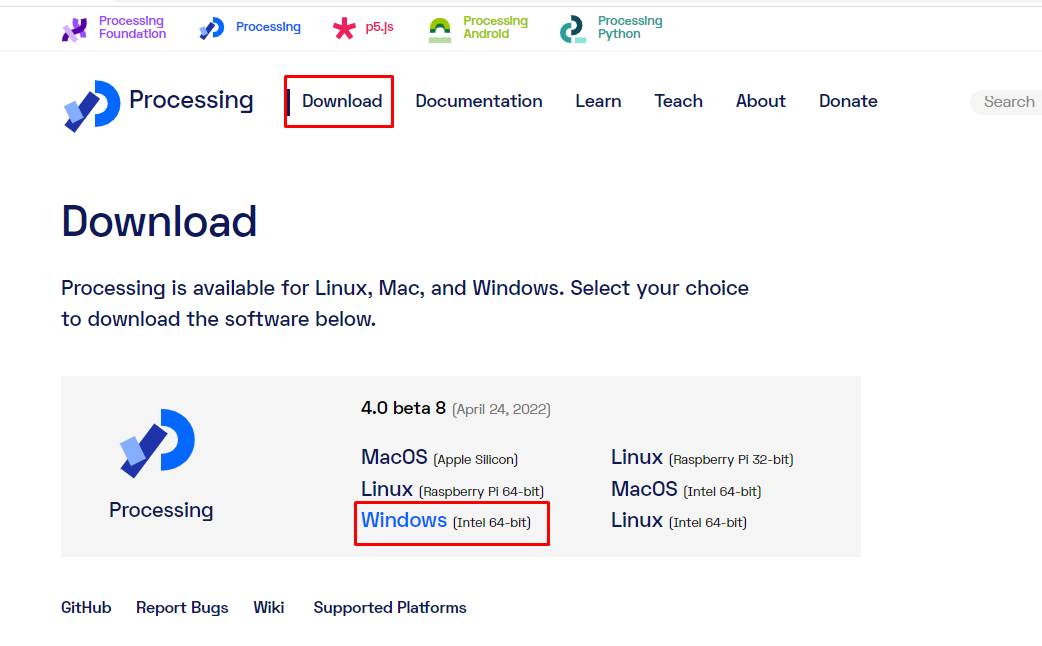
Image source from google
Download processing as per the system and install the app. Once we have installed than we can explore with coding and creating apps.
Processing layout
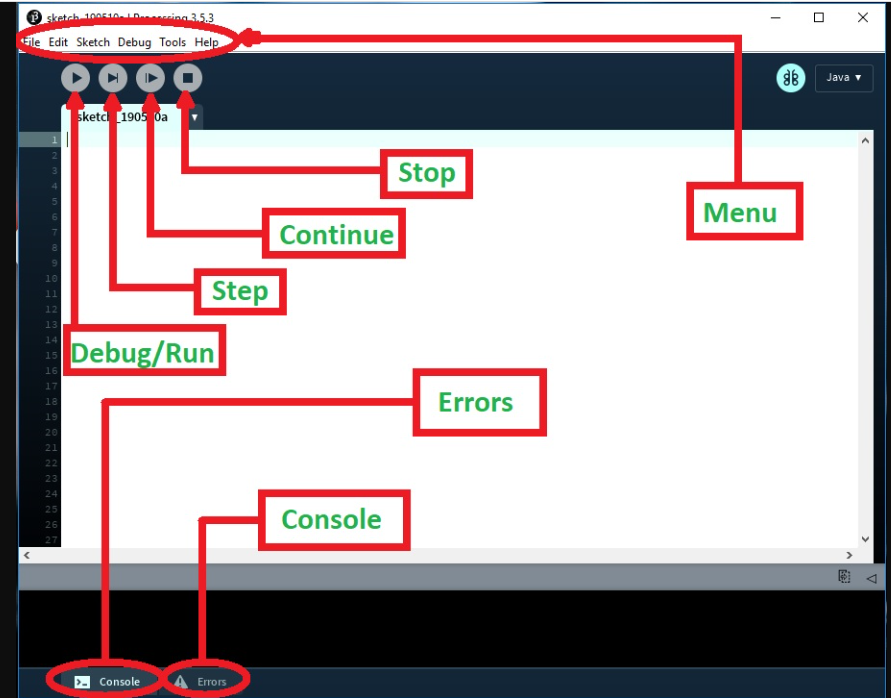
Getting Started with processing
Making a colourful Canvas and defining the size of the canvas
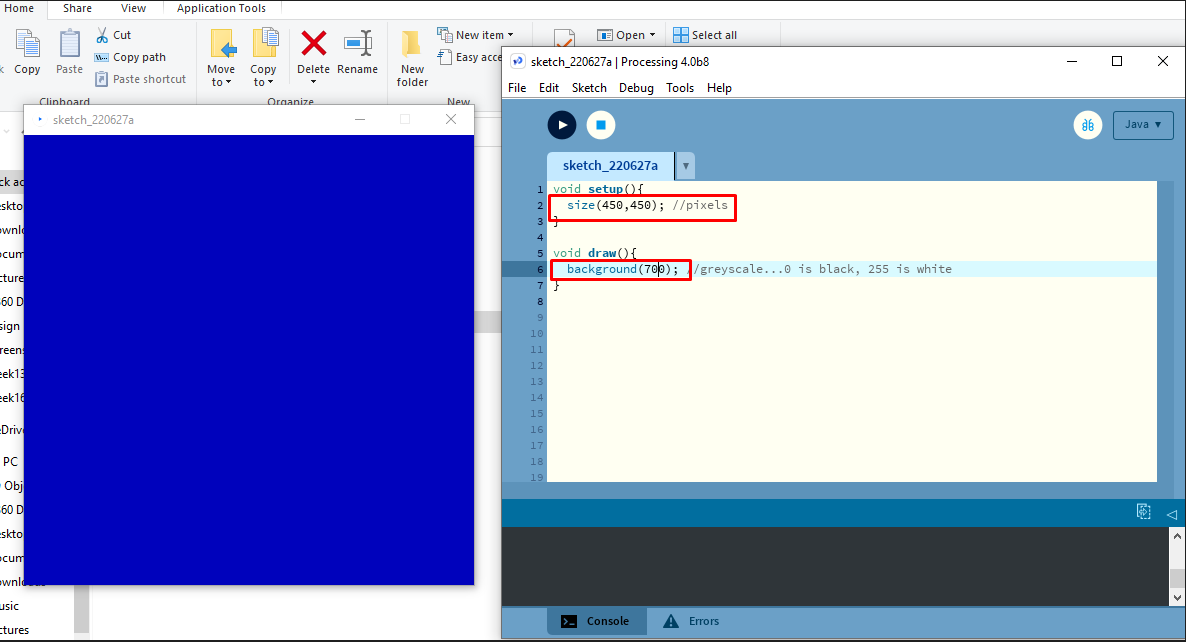
We can even change the color to RGB as shown in picutre. By changing the value of RGB we can change the colour of canvas
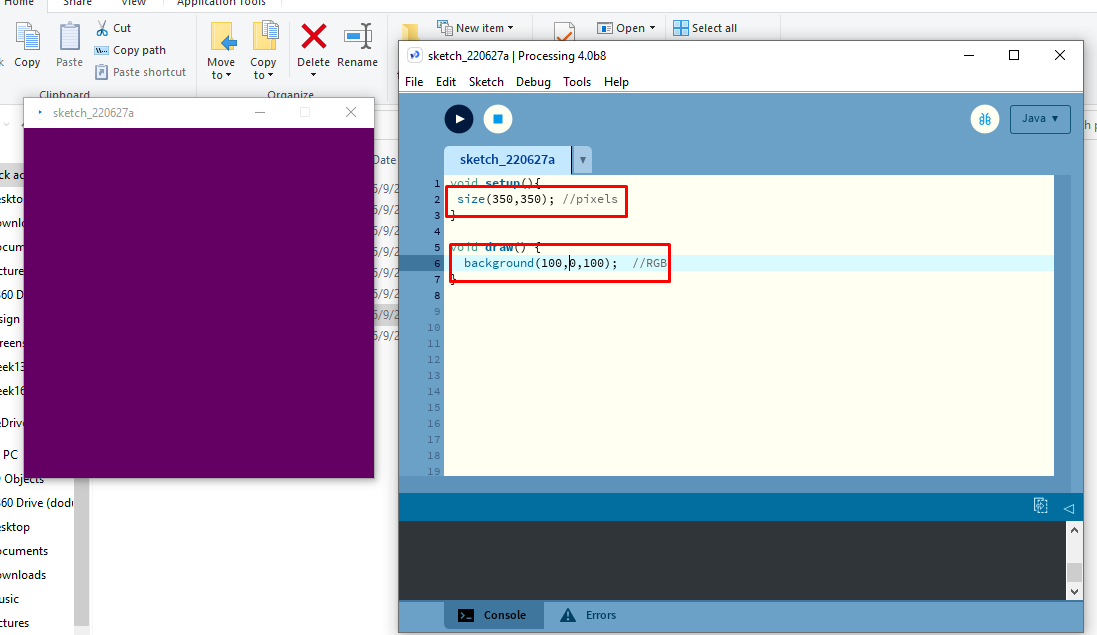
Than i created a rectangle button in the center of canvas. We can resize the rectangle also.
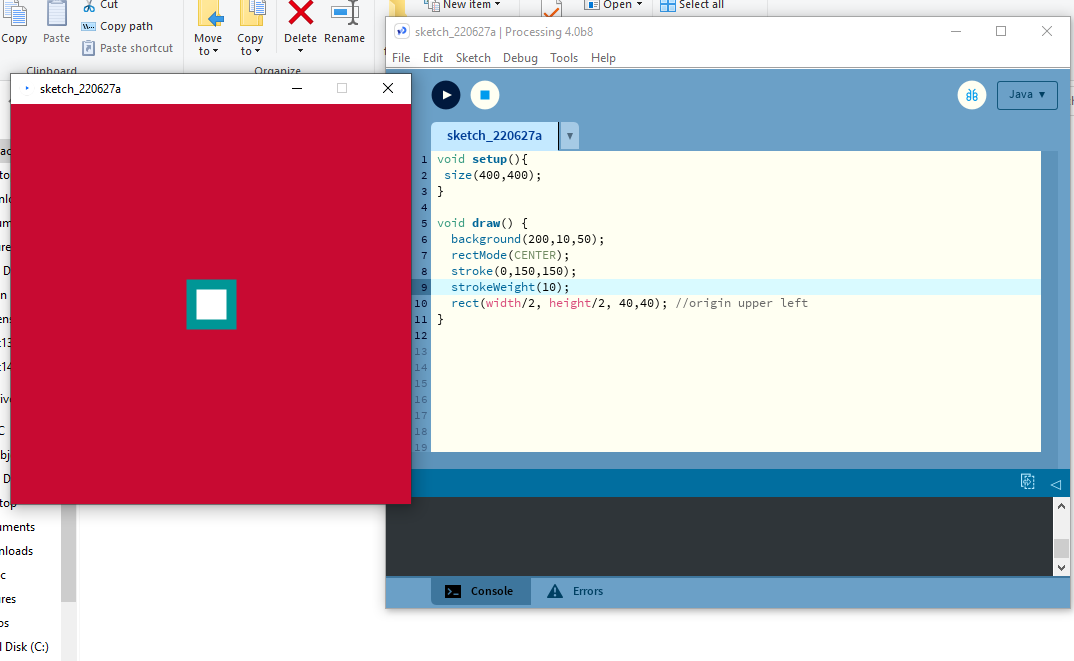
Creating a circle button in the center of canvas
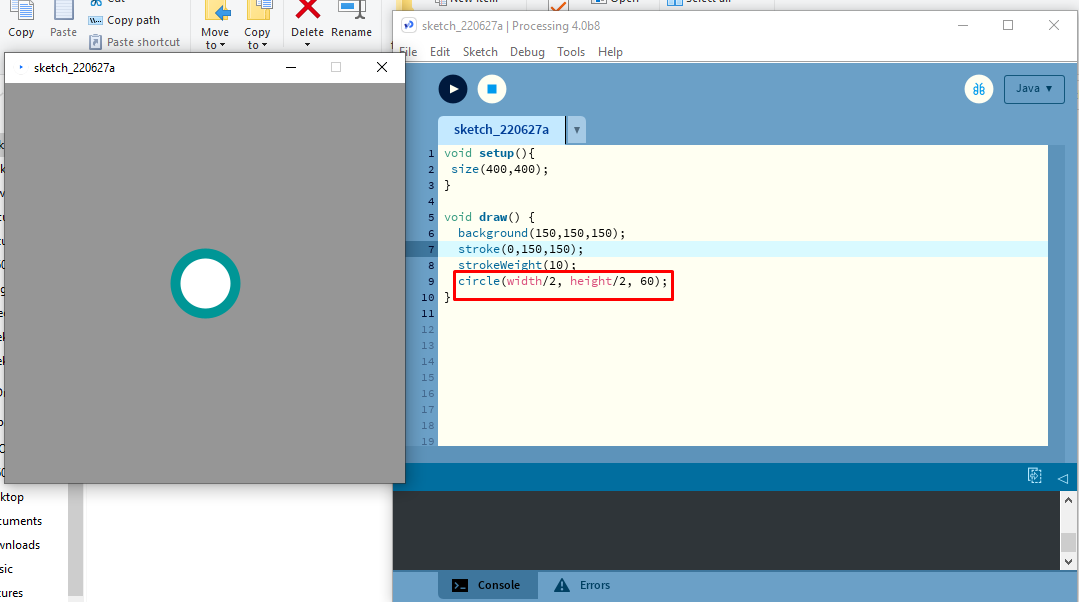
Code to write text on canvas. I wrote "ENTER" inside the circle
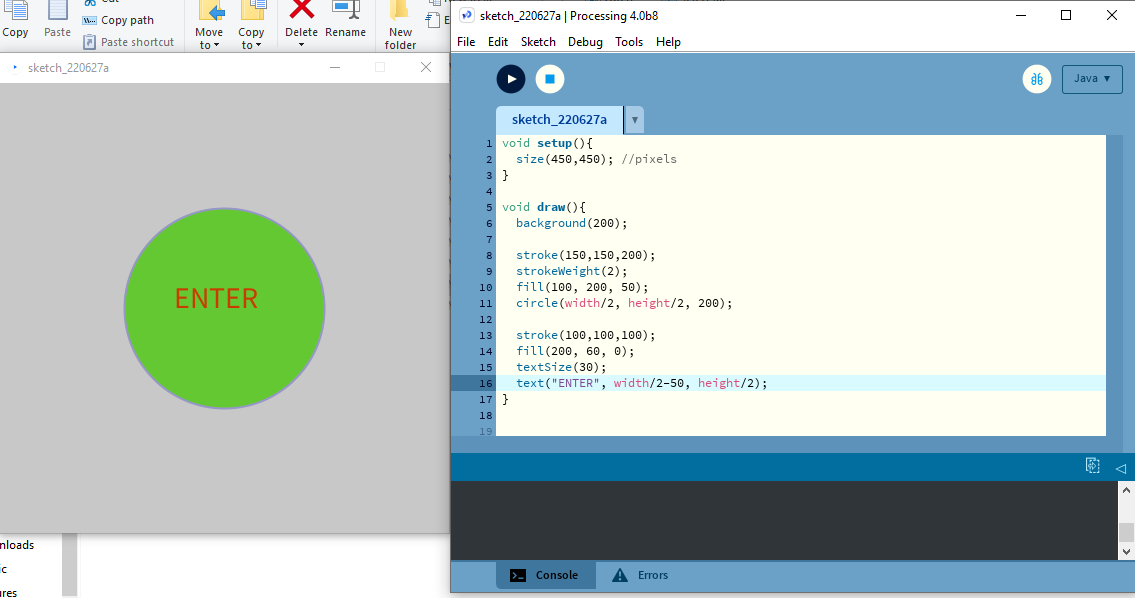
Communication between Arduino_IDE and processing
Sending data from arduino to processing over a serial port
To send a data from arduino to processing we need a microcontroller board. i used my Project board which has ESP32. So to program microcontroller from arduino i used FTDI cable
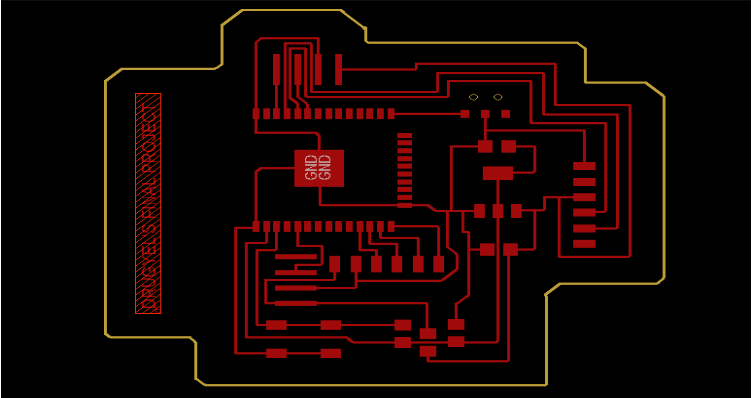
Open the arduino and i entered the coding as give in image below. The set up is where we can set up our program. so to start the serial communication from arduino our computer baud rate should be 115200. after set up prepare the loop which runs again n again. i tried to send "hello Drugyel"
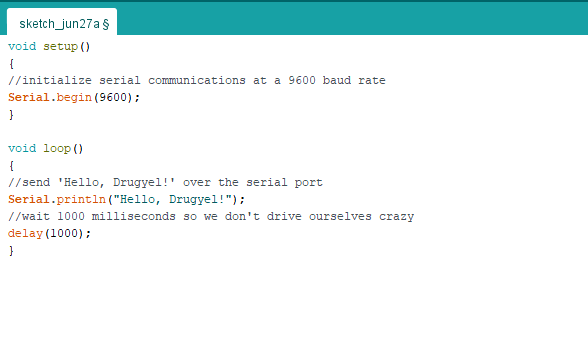
To setup processing open the software. Go to sketch than import library and click on serial
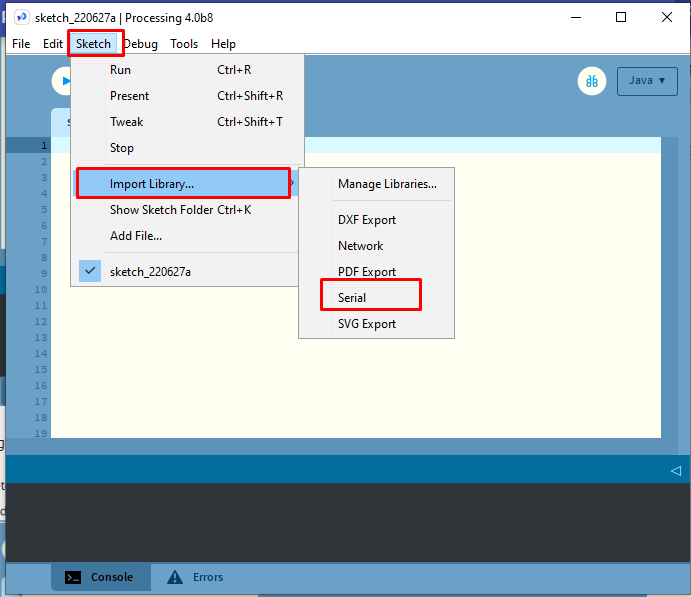
Now we can declare some global variables which can be used anywhere in sketch
Serial myPort; // create object from serial class
String val; // Data received from the serial port
We have to get serial object to listen to serial communication. It will allow us to listen to a serial port for any incoming data. I am sending a string "Hello Drugyel" from arduino_IDE and to receive the msg in processing we have to prepare processing haing similar coding style void setup() and void draw() instead of loo
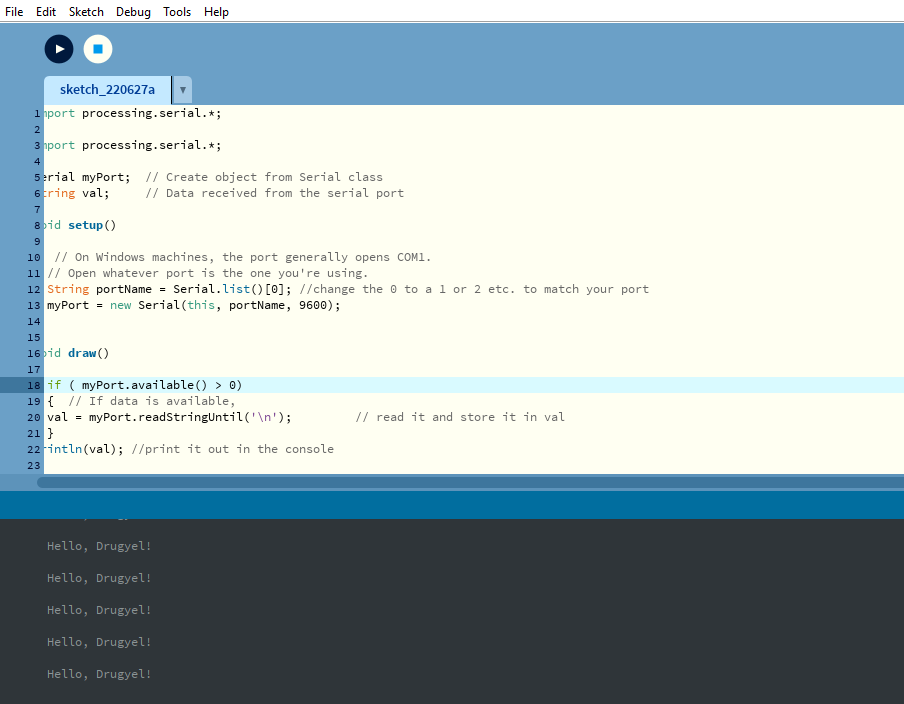
How to send data from Processing to Arduino?
In my above work I have sucessfully send the data from arduino to processing. Now let's send data from processing to arduino. every thing is similar to my last sketch.
To send a data from arduino to processing we need a microcontroller board. So i used my final project board which has a ESP32 WROOM.
To program ESP32, first we have to select the board. To do that, go to tools>> board>> ESP32 Arduino>> ESP32 Dev Module
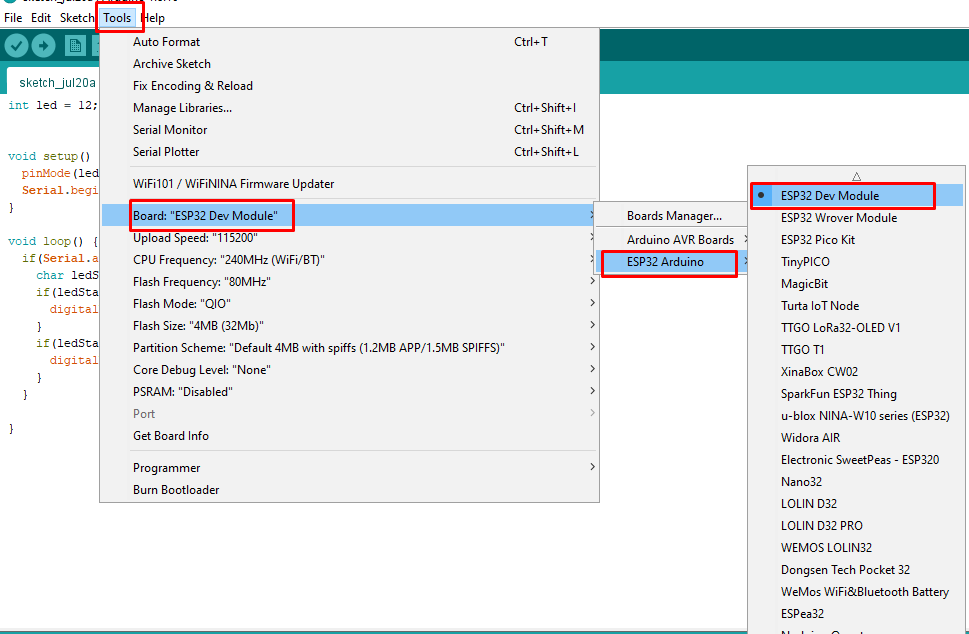
Once board is selected, next i selected the upload speed. To do so, go to tools >> Upload speed >> and i selected 115200
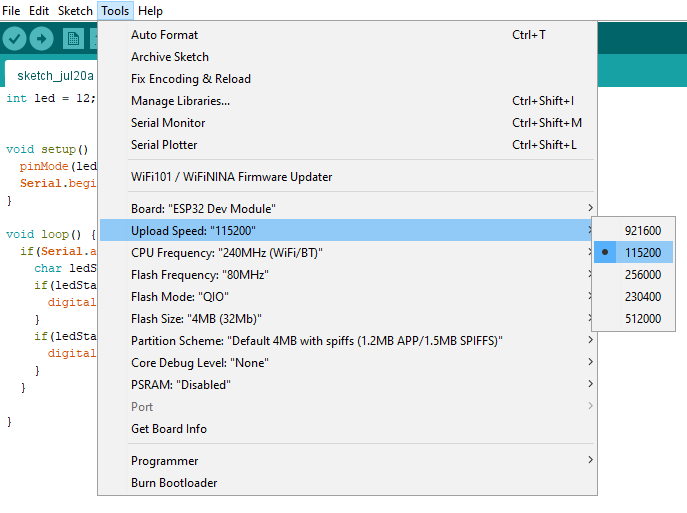
Next i selected the port for ESP32. For that select tools >> port>> COM7
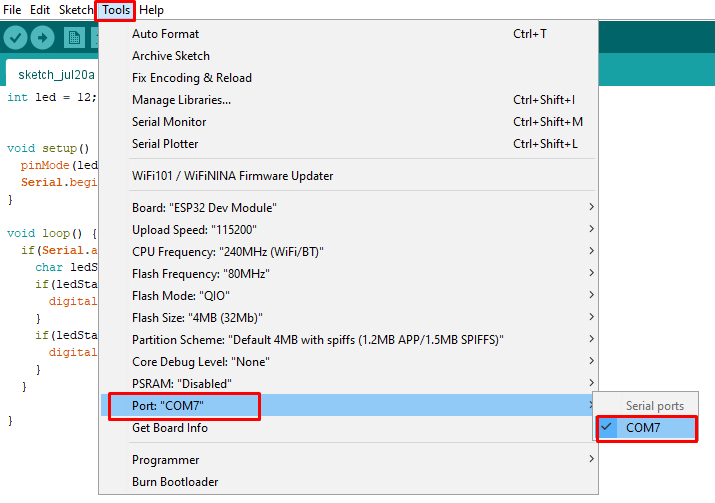
I have used FTDI module to program my board which has ESP32 microcontroller
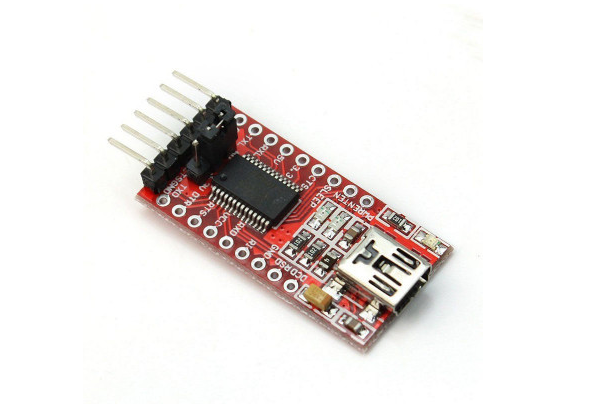
Than I compiled the arduino code to check for errors and to my delight no errors were found so i successfully uploaded it
Arduino Code
int led = 8; void setup() { pinMode(led, OUTPUT); Serial.begin(9600); } void loop() { if(Serial.available() > 0) { char ledState = Serial.read(); if(ledState == '1') { digitalWrite(led, ledState); } if(ledState == '0') { digitalWrite(led, LOW); } } }
Processing code to interface with arduino
It is a process of uploading a arduino code to ESP32 WROOM and make serial communication between processing and ESP32 WROOM. To make serial commucaton i have run the processing code in ESP32 WROOM.
Serial communication is communicatoin method that uses transmission lines to send data and receive data. At a time one bit of data will be send and receive continuously
Steps for uploading processing code to ESP32 WROOM
To be able to send serial data from processing to arduino IDE, we have to import the processing serial data library. to do that, select sketch >> import library>> Serial.
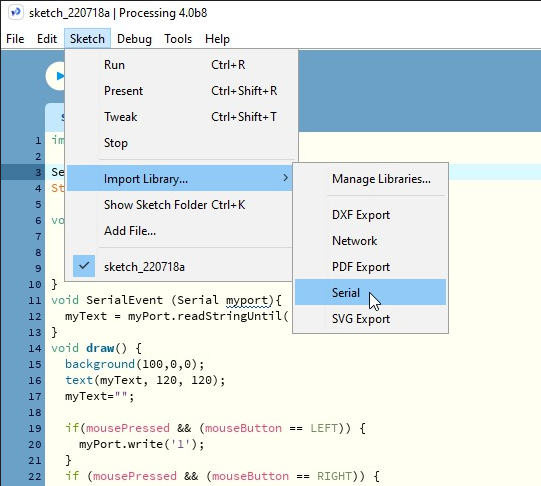
And i used the following processing code to interface with arduino where i declared the port as COM7.
For the background i used a RGB color combinationn of (100, 0, 0) which is red.
I used a mouse press function whereby when i click on the left button of mouse, the led will turn on and when i click the right button of mouse the led will turn off.
The processing code for mouse press function is given below;
if(mousePressed && (mouseButton == LEFT)) { myPort.write('1'); } if (mousePressed && (mouseButton == RIGHT)) { myPort.write('0'); }
The processing code to make serial communication with ESP32 WROOM.
import processing.serial.*; Serial myPort; String myText=""; void setup(){ size(300, 300); myPort = new Serial(this , "COM8", 9600); myPort.bufferUntil('\n'); } void SerialEvent (Serial myport){ myText = myPort.readStringUntil('\n'); } void draw() { background(100,0,0); text(myText, 120, 120); myText=""; if(mousePressed && (mouseButton == LEFT)) { myPort.write('1'); } if (mousePressed && (mouseButton == RIGHT)) { myPort.write('0'); } }
After making serial communication between ESP32 WROOM and processing when i click on left button of mouse on the canvas the LED will glow. When I click on right button of mouse on the canvas LED will turn off