Assignment
INDIVIDUAL ASSIGNMENT:
Write an application that interfaces a user with an input &/or output device that you made
GROUP ASSIGNMENT:
Compare as many tool options as possible
Interface and Application Programming
This week our assignment is to write an application that can interface with an input or output device.What I did
- Made android app using MIT app inventer
- Connected bluetooth module and RTC
MIT APP INVENTOR
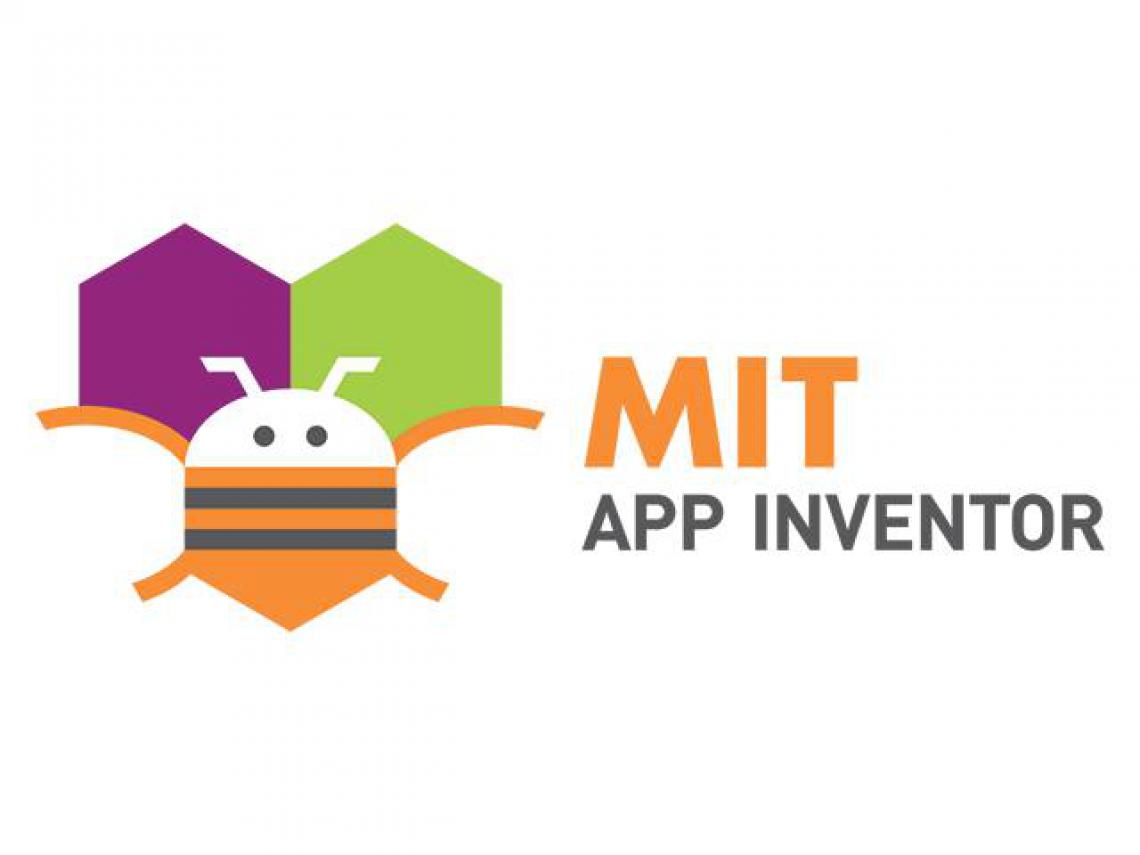
App Inventor for Android is an open-source web application originally provided by Google, and now maintained by the Massachusetts Institute of Technology (MIT).App Inventor lets you develop applications for Android phones using a web browser and either a connected phone or emulator.
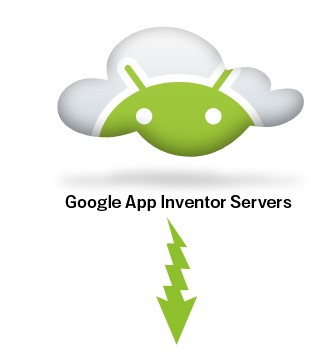
The App Inventor Components are located on the left hand side of the Designer Window under the title Palette. Components are the basic elements you use to make apps on the Android phone.
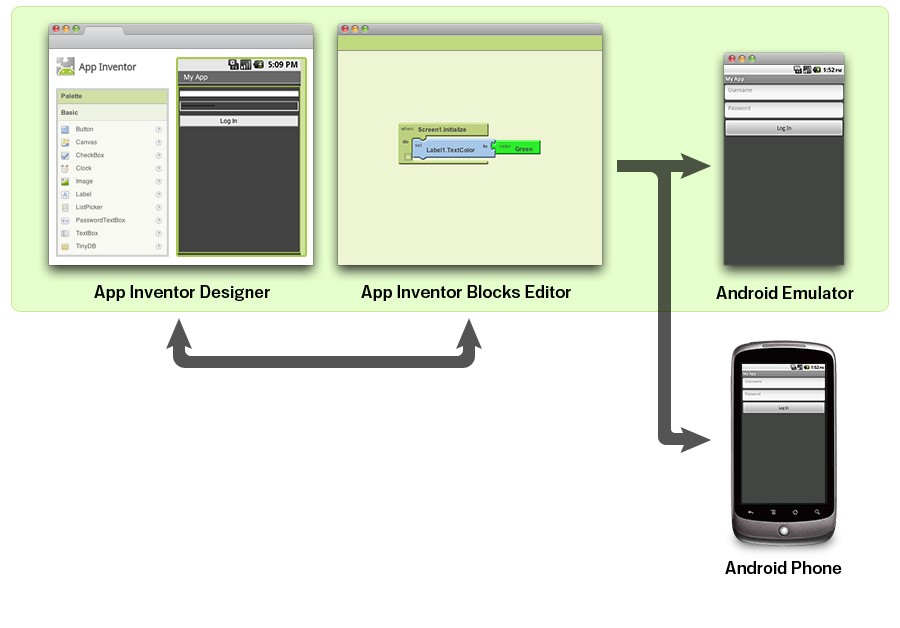
Your app appears on the phone step-by-step as you add pieces to it, so you can test your work as you build. When you're done, you can package your app and produce a stand-alone application to install. Here we are going to control LED light by using our phone , for this we need an Application so we are using MIT App inventor. The App Inventor development environment is supported for Mac OS X, GNU/Linux, and Windows operating systems, and several popular Android phone models. Applications created with App Inventor can be installed on any Android phone. Before we use App Inventor, we need to set up our computer and install the App Inventor Setup package on our computer.
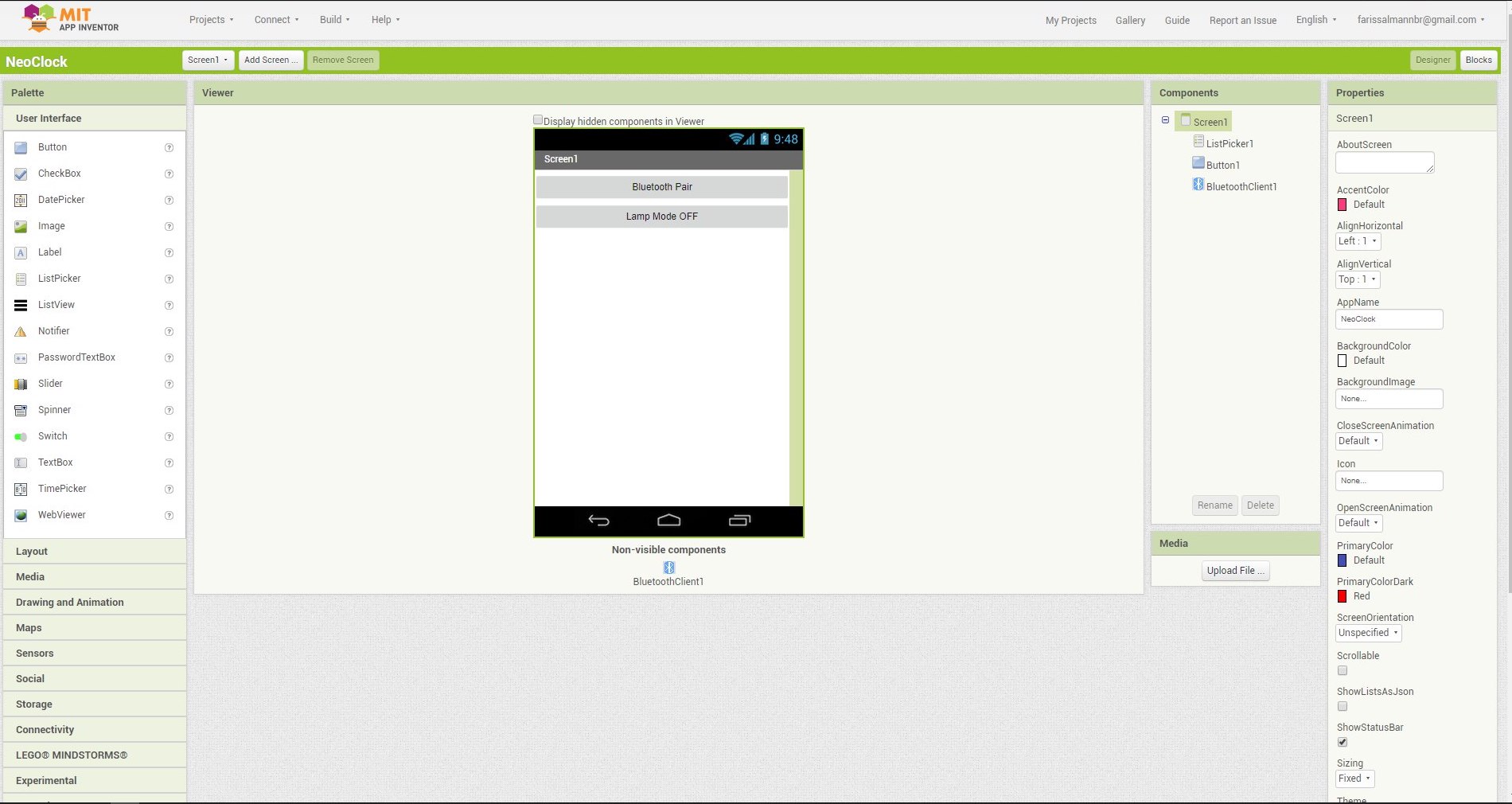
we are using ListPicker for the bluetooth selection.
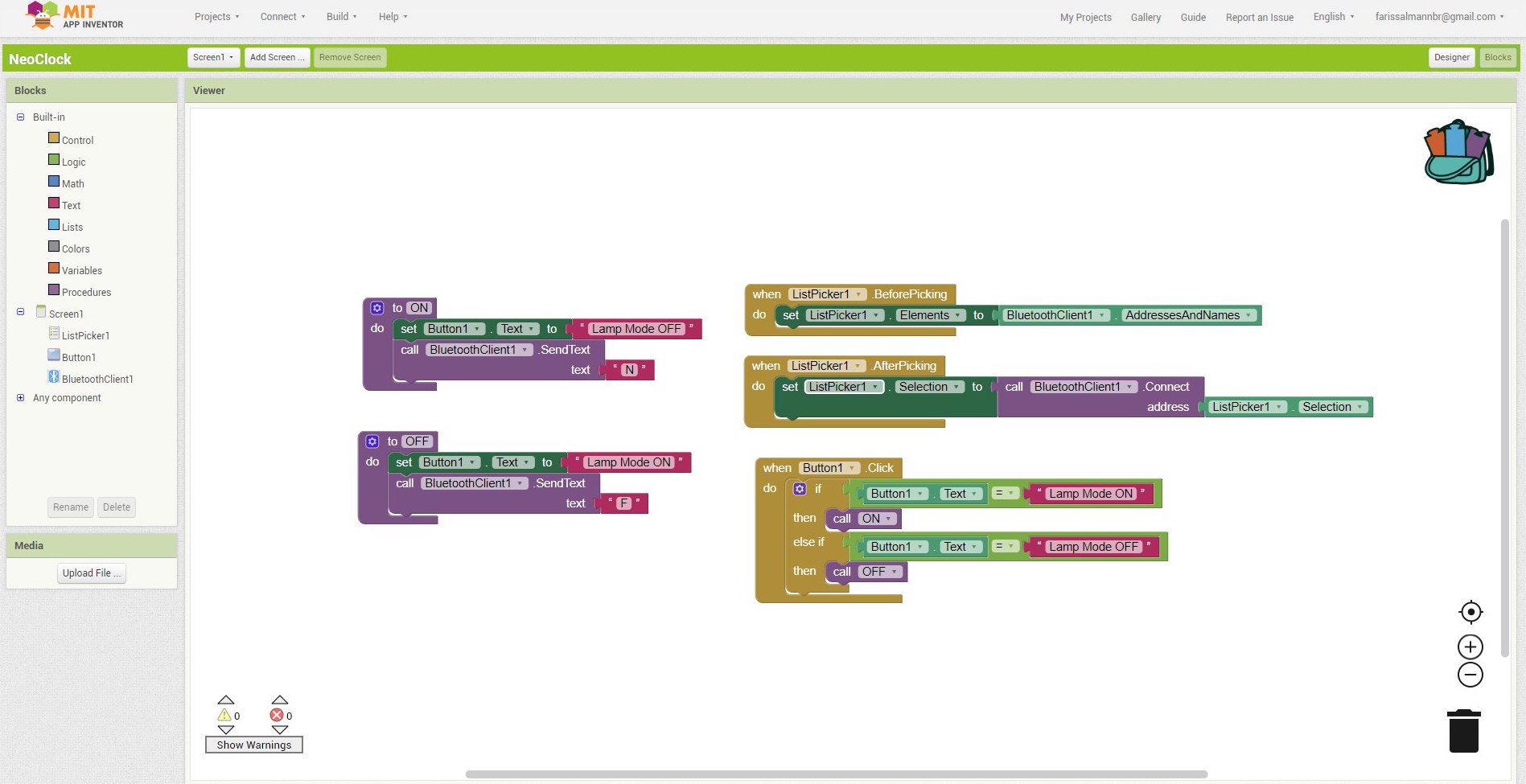
BLUETOOTH SELECTION
I have used ListPicker for the bluetooth selection
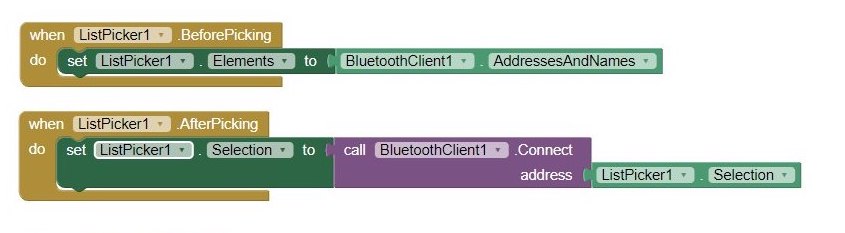
ListPicker have two state , one is BeforePicking state,in here we are loading bluetooth names in the list, second is AfterPicking here we are connecting the Bluetooth device that loaded on the ListPicker BeforePicking state , and also we are changing the text of the ListPicker in to Connected when we establish a bluetooth connection.
BUTTON ACTIVITYwe are using two buttons for contorling LED state.
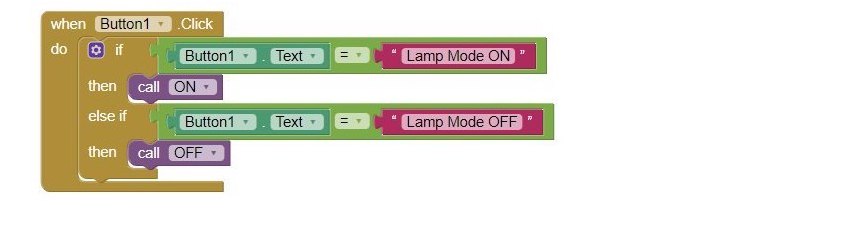
when we click a button bluetooth will transmit a text to device that connectedw with phone(we alredy connected a bluetooth device using ListPicker Bluetooth Selection). here if we press the Button2 it will transmit letter 'A' and if we press the Button3 it will transmit letter 'B' ,that's all.
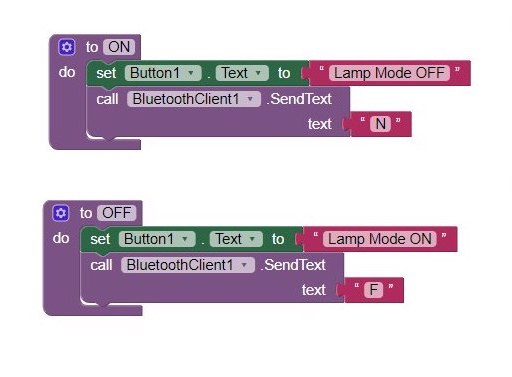
#include <Adafruit_NeoPixel.h> #include <TimeLib.h> #include <Wire.h> #include <DS1307RTC.h> #define PIN 9 #define DIGITS 6 #define S1_START 0 #define S2_START 7 #define P1_START 14 #define S3_START 16 #define S4_START 23 #define P2_START 30 #define S5_START 32 #define S6_START 39 Adafruit_NeoPixel strip = Adafruit_NeoPixel( 7 * DIGITS + 4, PIN, NEO_GRB + NEO_KHZ800); byte segments[10] = { 0b1110111, //0 0b0010001, //1 0b1101011, //2 0b0111011, //3 0b0011101, //4 0b0111110, //5 0b1111110, //6 0b0010011, //7 0b1111111, //8 0b0111111, //9 }; void setup() { Serial.begin(9600); strip.begin(); setSyncProvider(RTC.get); // colorWipe(strip.Color(255, 0, 0), 50); // colorWipe(strip.Color(0, 255, 0), 50); // colorWipe(strip.Color(0, 0, 255), 50); // // rainbowCycle(2); } void loop() { char data = Serial.read(); bool dataF = false; if (data == 'N') { dataF = true; } if (data == 'F') { dataF = false; } if (dataF) { clearDisplay(); white(); Serial.println("ON"); delay(5000); } if (!dataF) { Serial.println("OFF"); clearDisplay(); printSeconds(); printMinute(); printHour(); strip.show(); dotOn(); delay(500); dotOff(); delay(500); } } void writeDigit(int segStart, int value) { byte seg = segments[value]; for (int i = 6; i >= 0; i--) { int offset = segStart + i; uint32_t color = seg & 0x01 != 0 ? strip.Color(50, 0, 0) : strip.Color(0, 0, 0); strip.setPixelColor(offset , color); seg = seg >> 1; } } void clearDisplay() { for (int i = 0; i < strip.numPixels(); i++) { strip.setPixelColor(i, strip.Color(0, 0, 0)); } strip.show(); } void dotOn() { strip.setPixelColor(14, strip.Color(0, 255, 0)); strip.setPixelColor(15, strip.Color(0, 255, 0)); strip.setPixelColor(30, strip.Color(0, 255, 0)); strip.setPixelColor(31, strip.Color(0, 255, 0)); strip.show(); } void dotOff() { strip.setPixelColor(14, strip.Color(0, 0, 0)); strip.setPixelColor(15, strip.Color(0, 0, 0)); strip.setPixelColor(30, strip.Color(0, 0, 0)); strip.setPixelColor(31, strip.Color(0, 0, 0)); strip.show(); } void white() { for (int i = 0; i <= 50; i++) { strip.setPixelColor(i, strip.Color(100, 100, 100)); delay(10); } strip.show(); } void printSeconds() { int secondT = second(); int secondTF = secondT / 10 ; writeDigit(S5_START, secondTF); int secondTS = secondT % 10; writeDigit(S6_START, secondTS); } void printMinute() { int minuteT = minute(); int minuteTF = minuteT / 10 ; writeDigit(S3_START, minuteTF); int minuteTS = minuteT % 10; writeDigit(S4_START, minuteTS); } void printHour() { int hourT = hourFormat12(); int hourTF = hourT / 10 ; writeDigit(S1_START, hourTF); int hourTS = hourT % 10; writeDigit(S2_START, hourTS); } void rainbowCycle(uint8_t wait) { uint16_t i, j; for (j = 0; j < 256 * 5; j++) { // 5 cycles of all colors on wheel for (i = 0; i < strip.numPixels(); i++) { strip.setPixelColor(i, Wheel(((i * 256 / strip.numPixels()) + j) & 255)); } strip.show(); delay(wait); } } uint32_t Wheel(byte WheelPos) { WheelPos = 255 - WheelPos; if (WheelPos < 85) { return strip.Color(255 - WheelPos * 3, 0, WheelPos * 3); } if (WheelPos < 170) { WheelPos -= 85; return strip.Color(0, WheelPos * 3, 255 - WheelPos * 3); } WheelPos -= 170; return strip.Color(WheelPos * 3, 255 - WheelPos * 3, 0); } void colorWipe(uint32_t c, uint8_t wait) { for (uint16_t i = 0; i < strip.numPixels(); i++) { strip.setPixelColor(i, c); strip.show(); delay(wait); } }Download arduino sketch here
Turning clock into table lamp
Download APK file here