
Interface and Application Programming --- Հավելվածների ծրագրավորում
Introduction --- Ներածություն
For this week we had to:
write an application that interfaces a user with an
input &/or output device that you made. (individual assignment)
I will try to make an application using Processing
that will communicate with my input devices week
boards via FabFTDI.
Installing Processing 3.5.3 on Linux --- Պրոցեսինգ 3.5.3-ի ներբեռնումը Լինուքսում
Go to Processing Download page select the
correct linux version you need (32bit 64bit).
At this point a download should start and you will get a .tgz archive. Extract it in
your "home" directory. After done open the
Processing 3.5.3 folder in your home directory, right click and select "Open in Terminal".
Type ./processing
and hit enter. At this point you should get Processing
working. If not visit Supported-Platforms
page for solutions. If you are going to access Serial ports type
sudo ./processing
otherwise you
will get this error:
Error opening serial port /dev/ttyACM1: Permission denied.
Using Processing 3.5.3 --- Պրոցեսինգ 3.5.3-ի օգտագործումը
The default coding language in processing is Java, but you can change it easily by pressing
the "Java" buttun at the right upper corner.
There are lot of tutorials and examples you can use and learn with for
processing.
I started with setting up serial communication and with my FabFTDI and reading data from
button board I made during Input Devices week. My button board
was programmed to send "b" when button pressed and "u" when released.
I used the default example for serial read.
// Example by Tom Igoe
import processing.serial.*;
// imports serial library
Serial myPort;
// The serial port
void setup() {
// Open the port you are using at the rate you want:
myPort = new Serial(this, Serial.list()[0], 4800);}
// default baudrate is
9600 but FabFTDI supports up to 4800.
void draw() {
// draw() loops forever, until stopped.
while (myPort.available() > 0) {
// myPort.available() Returns the
number of bytes available.
int inByte = myPort.read();
// myPort.read() Returns a number
between 0 and 255 for the next byte that's waiting in the buffer.
println(inByte);}}
// prints the value of inByte.
To run the program you should save it and hit the play buttun at the upper left corner.
When I pressed the button on the board I got 98 and when released I got 117.
This are the ASCII codes for "b" and "u".
I changed the second-to-last line of the program to this:
char inByte = myPort.readChar();
// Returns the next byte in the buffer as
a char.
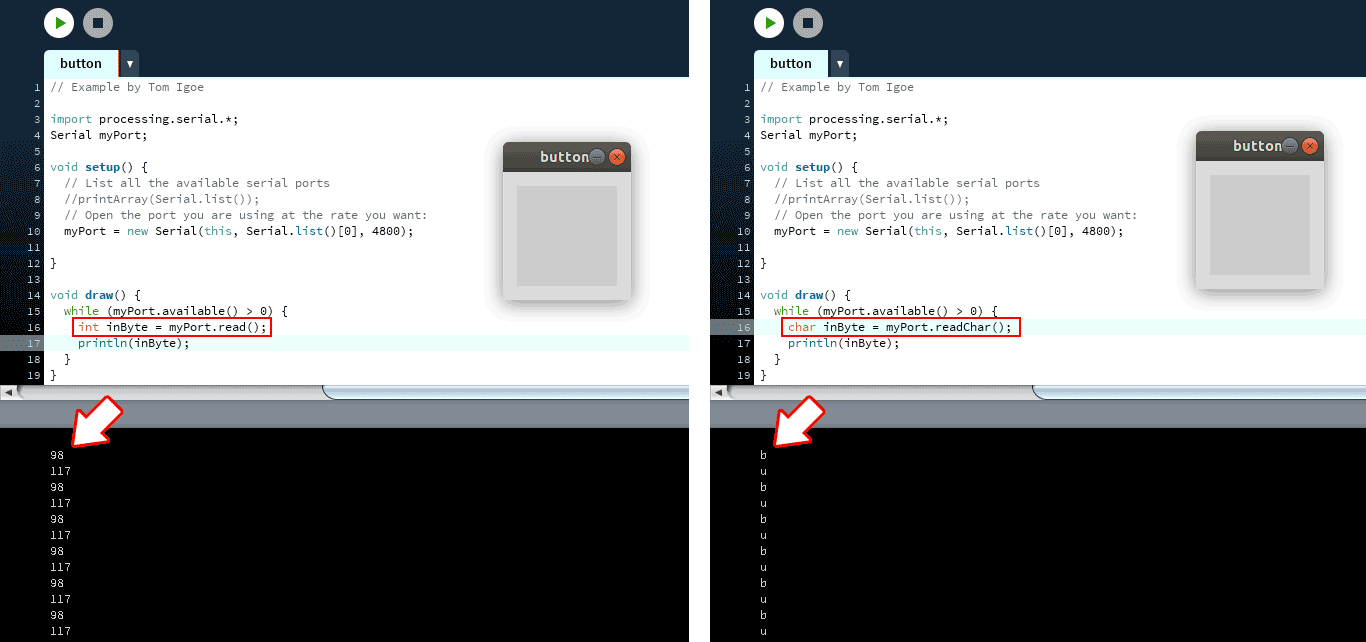
I added this line at the bottom of the code:
background(inByte-50)
// This changes the background color of the
application based on the integer number it has in it 0-white 255 is black.
If you want colors put 3 numbers like this background(255,255,255) this is RGB color code.
Here's what I got:
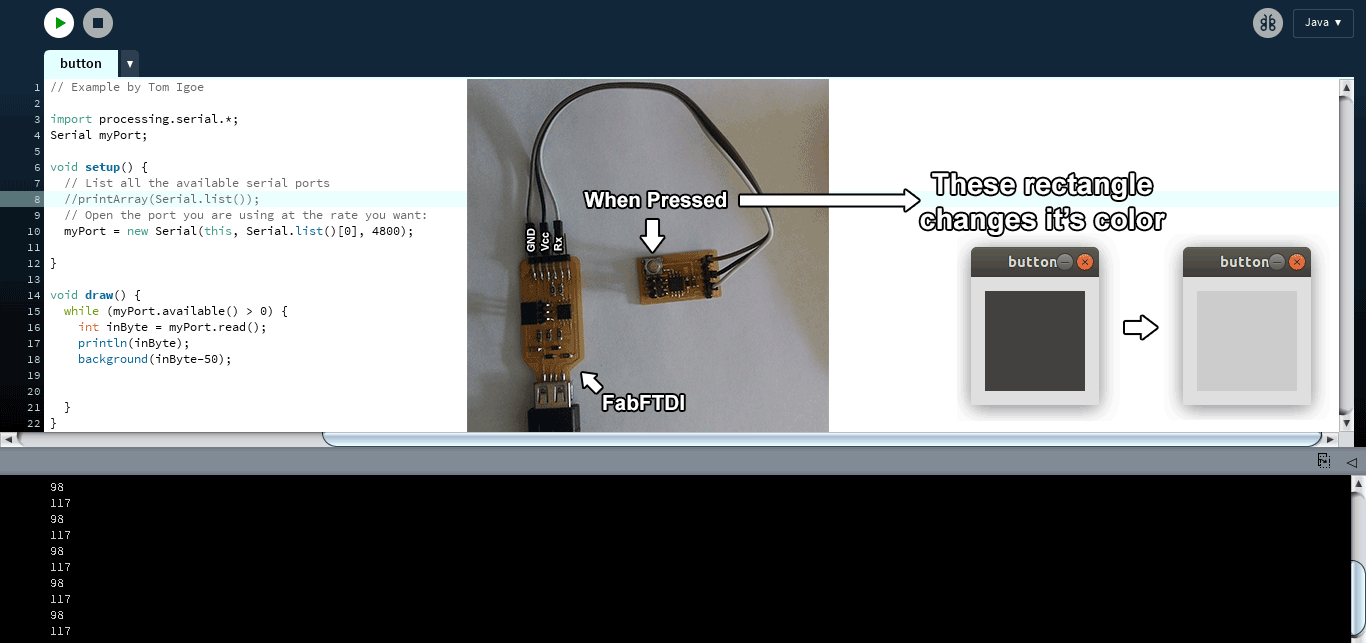
My application --- Իմ հավելվածը
After watching and reading some tutorials I came across the Bouncing Ball
tutorial and decides to make a simple game where a ball tries to go down and by pressing the button it goes up.
The goal is to keep the ball away from the ground. At first I made it to work with my input device button.
Here's the code:
import processing.serial.*;
Serial myPort;
float x=0, y=300, xSpeed=2, ySpeed=2 ;
// 4 variables declared
void setup() {
size(800,400);
// defines the size of the screen in pixels
myPort = new Serial(this, Serial.list()[0], 4800);}
void draw() {
fill(random(100),random(255),random(255));
// Sets the color used to fill shapes randomly
x += xSpeed;
// same as x=x+xSpeed
y += ySpeed;
// same as y=y+ySpeed
if (x > width || x < 0 ){
// if the ball reaches the corners
xSpeed *= -1;}
// changes the direction of xSpeed=xSpeed*-1
ellipse(x, y, 50, 50);
// defines the ball with the size 50px by 50px
while (myPort.available() > 0) {
char inByte = myPort.readChar();
println(inByte);
if (inByte == 'b' || keyPressed){
// if the button is pressed
ySpeed = -2;}
// changes the direction of ySpeed (the ball goes up)
else{
ySpeed = 2;}}}
// the ball goes down
Here's the result:
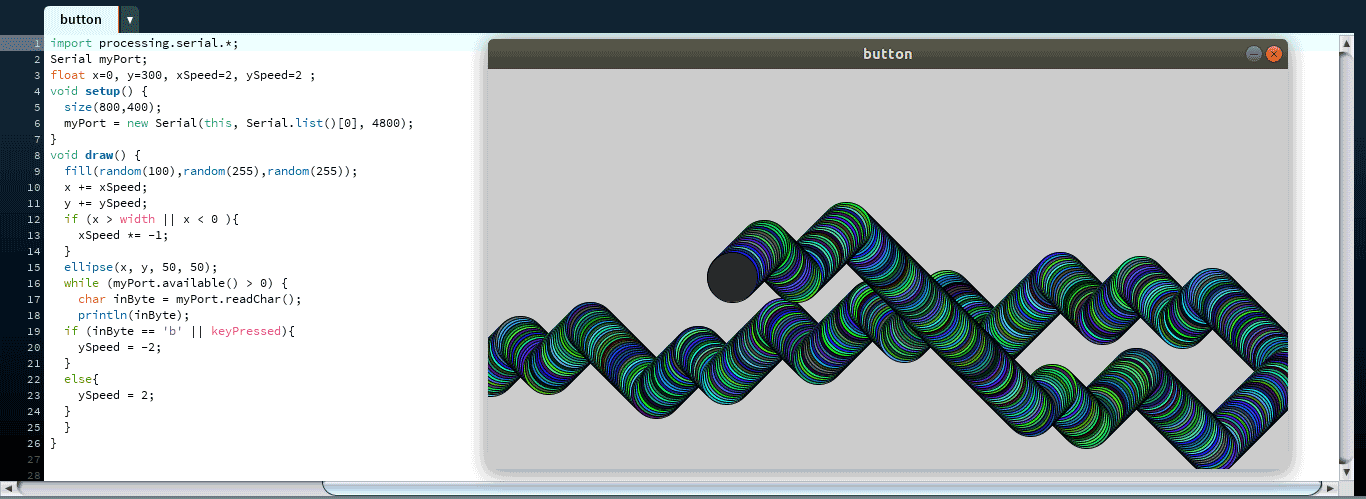
Then I thought it might be a better idea to make it with the keyboard and embedd this game in my website.
I removed the serial lines and added a helping text.
int state=0;
float x=0, y=200, xSpeed=2, ySpeed=2;
void setup() {
size(800,400);}
void mousePressed() {
if (mousePressed) {
// if the mouse is pressed
state = 1; }
// start
else {
state = 0; }}
// wait
void draw() {
if (state==0) {
textSize(30);
textAlign(CENTER);
text("Click to Start and press a letter", 400, 30);
fill(0, 102, 153);}
else if (state==1) {
// if mouse is pressed start the game
fill(random(100),random(255),random(255));
y += ySpeed;
if (x > width || x < 0 ){
xSpeed *= -1;}
ellipse(x, y, 50, 50);
if (keyPressed){
// if any key on the keyboard is pressed
ySpeed = -2;}
// the ball goes up
else{
// if no key is pressed
ySpeed = 2;}}}
// the ball goes down
Here ia my final program:
How to embedd Processing app in the website
Put your processing APPNAME.pde file in the same folder where your webpage is. Go to processingjs.org and download the latest processing.js files
and place it in the same folder as your webpage. Now add this to your HTML:
< canvas data-src="APPNAME.pde"></canvas>
Also add this at the end of the HTML document
< script type="text/javascript" src="processing.js"></script>
And you will get your processing app embedded.
Here are the files:
button.pde this works with serial button.
button_2.pde this works with the keyboard