networking and communications (week14)
Group Assignment
- individual assignment:
- write an application that interfaces a user with an input &/or output device that you made
- group assignment:
- compare as many tool options as possible
MQTT
Network - host MQTT configuration
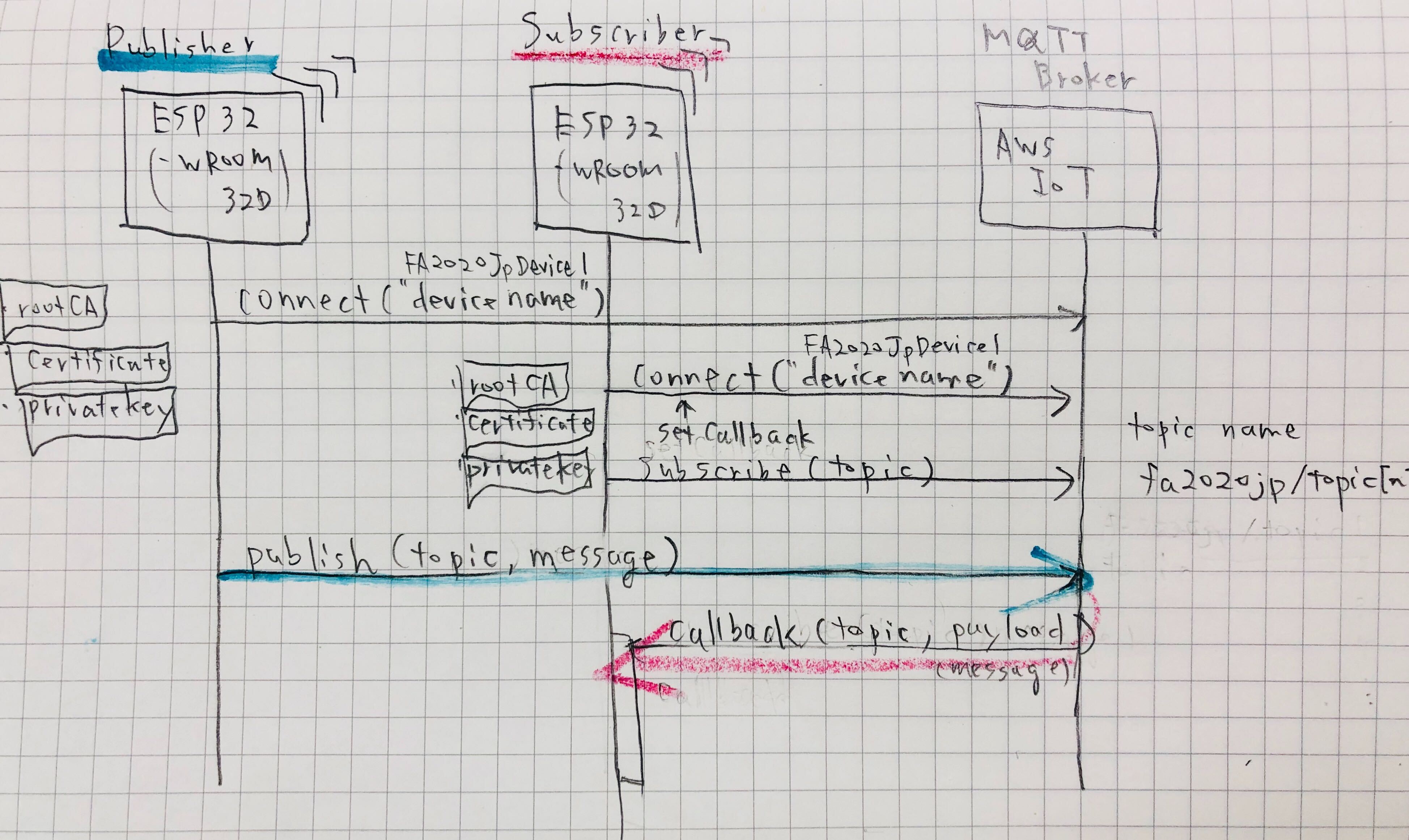
I hosted MQTT broker on AWS IoT and configured things, certification and security, policy.
The purpose of this is benchmarking MQTT for validating configuration, coding and performance. If it's useful, this is one of candidate networking infrastructures to make a "distributed musical device" idea, that Japan Fabacademy 2020 students work together for make musical device for each home location and play it together in remote place. This project is for group assignment of mechanical design and machine design week.
I described about general configuration in my individual page. Please look thorough:
MQTT client implementation on ESP32
Environment and libraries
- Environment (as an example)
- Board: ESP32 DevkitC
- IDE: Arduino IDE (1.8.10) on Mac OS Mojave (10.14.6)
- Board manager on Arduino IDE: ESP32 Wrover Module
- Wifi: 2.4GHz (802.11ac)
- Library for Arduino code
- ArduinoJson by Benoit Blanchon (ver 6.9.1)
- PubSubClient by Nick O'Leary (ver 2.7.0)
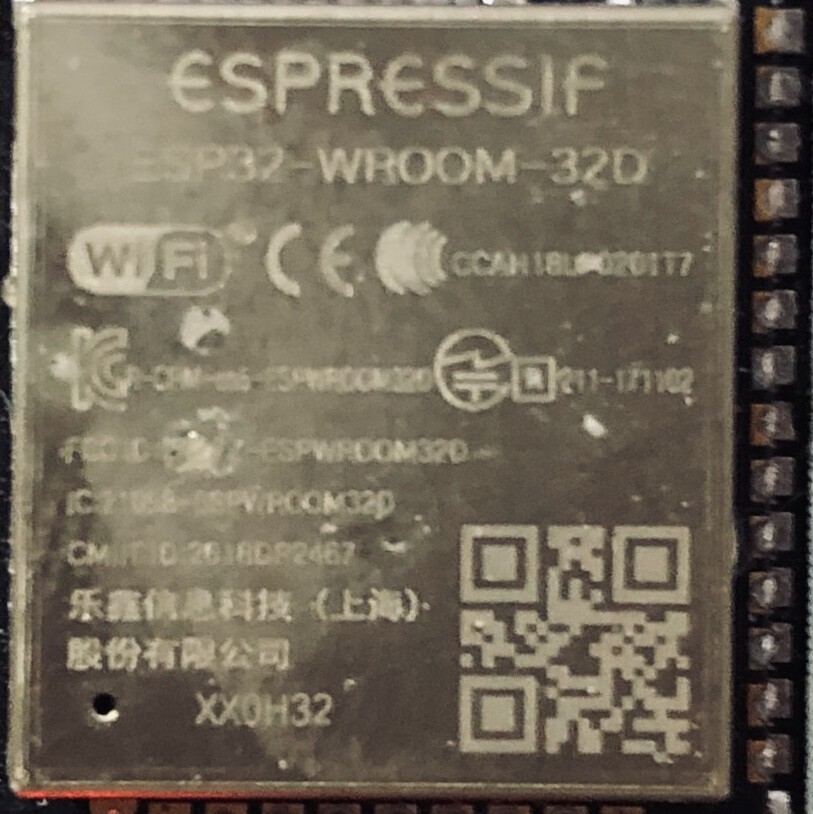
Client implementation procedure
- Environmental setup
-
- Download client source code template
- mqtt_aws_v3.ino(Google Drive, with SSL certification information). < Please download from here (You need to login to your google account. Pease contact to tato.flam@gmail.com for access permission with your name and google account).
- mqtt_aws_v3.ino(Gitlab, clean up security information)
- Setup board manager
- Install required libraries on Arduino IDE
- ArduinoJson by Benoit Blanchon (ver 6.9.1)
- PubSubClient by Nick O'Leary (ver 2.7.0)
- Configure max packet size configuration in PubSubClient.h
On Arduino IDE, Tool > Board > ESP Wrover Module
Check other parameters like "partition scheme" as "Default 4MB with spiffs"On Arduino IDE, Tool > Manage Library , Install following libraries. Make sure following version especially in major version more than ArduinoJson ver.6 and PubSubClient ver.2)
If you cannot find "Manage libraries" in "Tool" tab on Arduino IDE, check "Sketch" > "Include Library" "Manage Libraries".
At PubSubClient.h in library, Change
#define MQTT_MAX_PACKET_SIZE 128
to#define MQTT_MAX_PACKET_SIZE 512
PubSubClient.h will be the location like in ~/[Arduino home]/libraries/PubsubClient/src in your local computer.
- Coding
-
- Set your WiFi SSID and password
- Configure your topic (or for your use to publish/receive message)
- Specify your topic name to subscribe in connectAWSIoT()
- Write your logic on receiving message in mqttCallback()
- Write your logic for publishing message in mqttLoop()
// 1)----- set your ssid and password ----- char *ssid = "xxxxxxxxxx"; char *password = "xxxxxxxxxx";
// 2)----- configure your topic (or for your use to connect to other people) ----- // Topic name needs to be format in "fa2020jp/topic[*]" // Topic for publishing (if you do not need to publish, you do not need pubTopic. char *pubTopic1 = "fa2020jp/topic1"; char *pubTopic2 = "fa2020jp/topic2";
Following is topic name rule for FabAcademy 2020 MTM Japan group project. Following is just a rule for asigninig topic. In program, topics are generated dynamically under policy of "fa2020jp/topic[*]".
person topic name memo Common fa2020jp/topic0 common topic for everyone. You may publish here for dispatch message used for everyone Homma fa2020jp/topic1 Kimura fa2020jp/topic2 Tsuchiyama fa2020jp/topic3 Oka fa2020jp/topic4 HARU(Oguri) fa2020jp/topic5 Yanome fa2020jp/topic6 Yume fa2020jp/topic7 Tamiya fa2020jp/topic8 // 3) ----- Specify your topic name to subscribe ----- mqttClient.subscribe([topic name], qos);
// 4) ----- Write your logic on received message ----- void mqttCallback (char* topic, byte* payload, unsigned int length) { // }
void mqttLoop() { if (!mqttClient.connected()) { connectAWSIoT(); } mqttClient.loop(); // 5) ----- Write your logic for publishing message from here ----- // (If you are not publishing anything, you can delete following code)
Rules for implementing MQTT client (to "FA2020JpDevice1" in MQTT broker)
- For publishing, please consider your test code not running in unnecessarily frequency. AWS IoT is cheap but costs slightly (like 0.096US/1M connections, 1.20USD/1B messages)
- Please disconnect your client after you finish to use it by the same reason with above
- Check your topic name and use it
- Do NOT reveal certification information and source code embedded that information in any sharable place
Experiment result in multiple site
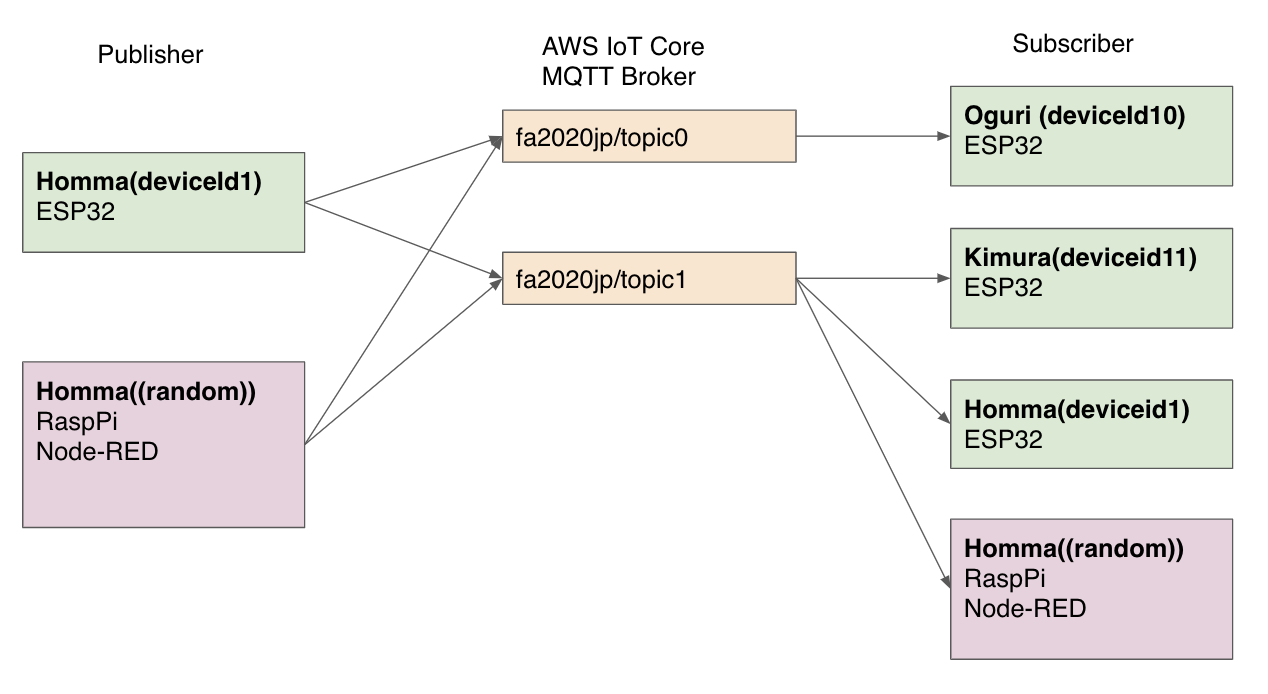
We did experiment for validate how multiple clients of MQTT work in remote location with hiroaki.kimura & hideo.oguri)
The result is in individual assignment page