Interface and application programming (week12)
Group Assignment
Compare as many tool options as possible.
As I want to make music instrumental device in my final project, I tried to see the sound interface using python libraries that can make sounds from python codebase. Also, I checked OLED to display input values by bitmap-based graphical interface.
Sound Interface using python libraries
For making sound from MIDI input to audio output on Raspberry Pi, I run following command on Raspberry Pi :
$ qjackctl & $ qsynth &
In detail, Raspberry Pi Tips - Fluidsynth
Generate audio files
Generate sine, sawtooth and square wave by numpy and scipy libraries.
- Environment: Rpi 3B, python 3.5.3 / 3.7.0
pip install numpy scipy
- Github
- Ref:
python-rtmidi
Search "Synth" output port and play midi file
- Environment: Rpi 3B, python 3.5.3 / 3.7.0
pip install python-rtmidi
- Github
- Ref:
Mido
- Environment: Rpi 3B, python 3.5.3 / 3.7.0
pip install mido python-rtmidi
MIDI note-on and note-off by Mido
Load MIDI file and check the length of music
Ref:
Pygame
- Environment: Rpi 3B, python 3.5.3 / 3.7.0
pip install pygame
List output ports
- Github
- Ref:
- 自由研究の準備(その6)PythonでのMIDI操作 (リアルタイム編1), (リアルタイム編2)
GUI keyboard - display piano and send MIDI message
- Github
- Ref:
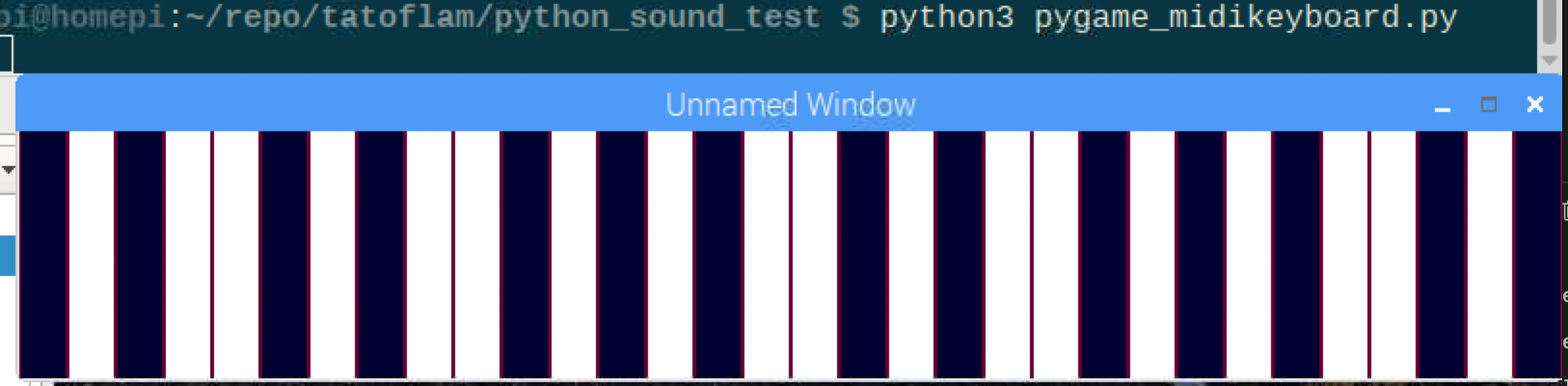
Pretty_midi
- Environment: Rpi 3B, python 3.5.3 (I could not install pretty-midi to virtual env of python3.7.0 )
cd pretty-midi
python3 setup.py install
Make MIDI file of noteon and noteoff of "G4"
Load MIDI file
Ref:
Graphical Interface using OLED
Display bitmap over I2C
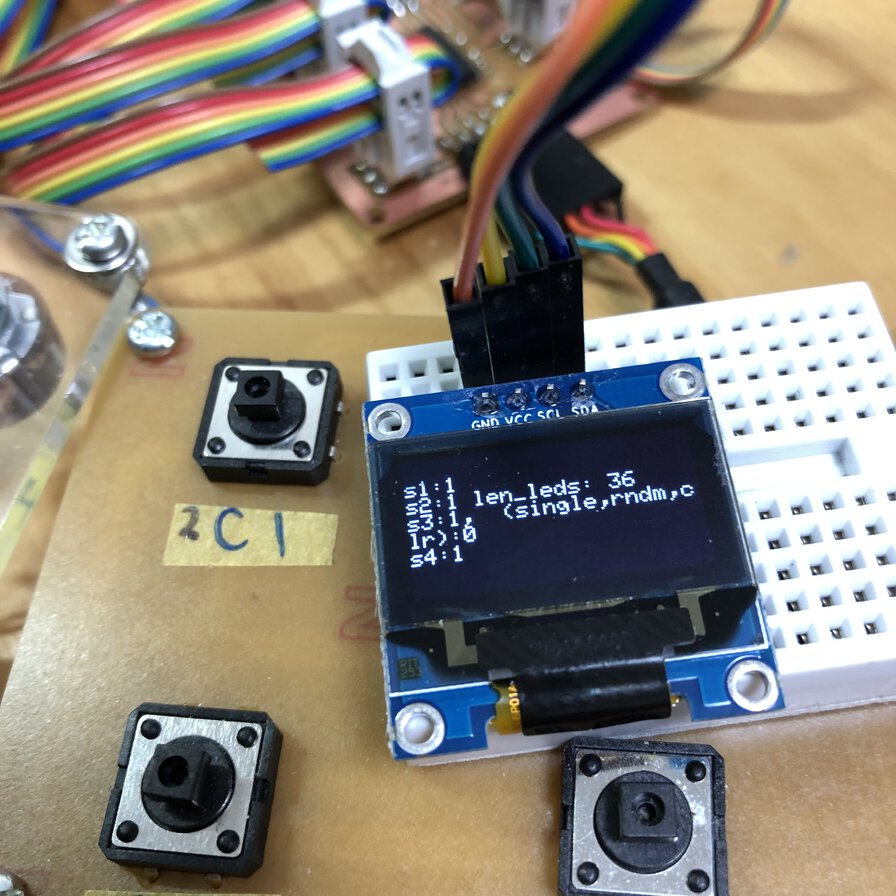
0.96 inches 128x64 dots OLED display
- Maker: SUNHOKEY Electronics Co.,Ltd.
- Vcc voltage: 3~5.5V
- Control protocol: I2C
- Microchip: SSD1306
- Resolution: 128x64 dot
- Color of charactor: white
- I2C address(default) 0x3C
I used software libraries to display the values from input devices
For using above libraries, I made a simple exampleas follows. I commented some important points in program to use above libraries on megaTinyCore(AVR 1-serires).
IC2.OLED.SSD1306.01.ino
#include#include #include #define SCREEN_WIDTH 128 // OLED display width, in pixels #define SCREEN_HEIGHT 64 // OLED display height, in pixels #define OLED_RESET -1 // Reset pin # (or -1 if sharing Arduino reset pin) Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, OLED_RESET); void setup() { // https://github.com/SpenceKonde/megaTinyCore // set the pins for SCL:PA2(15) and SDA:PA1(14) on ATtiny3216 // default is swap(0) that means SCL:PB0(9) and SDA:PB1(8) Wire.swap(1); // I2C address for OLED device is 0x78 (01111000) // after shift 1 bit, 00111100 is 0x3C display.begin(SSD1306_SWITCHCAPVCC, 0x3C); } void loop() { display.clearDisplay(); display.setTextSize(2); display.setTextColor(WHITE); display.setCursor(0, 10); display.println("Hello"); display.println("ATTiny3216"); display.display(); delay(1000); }
Test code to display value from input board to OLED over I2C
For my final project, I want to display the value that I selected by tactile switches.
Test code for displaying value from tactile switches controlled by ATtiny3216 input board is:
Graphics
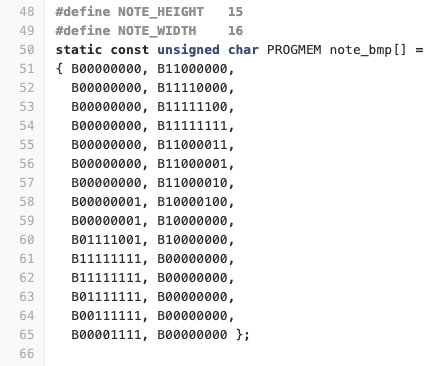
For displaying the note mark of "♪", I created a graphics by 15x16(height x width) pixels.
The hight and pixel match with the size of text and row of OLED.
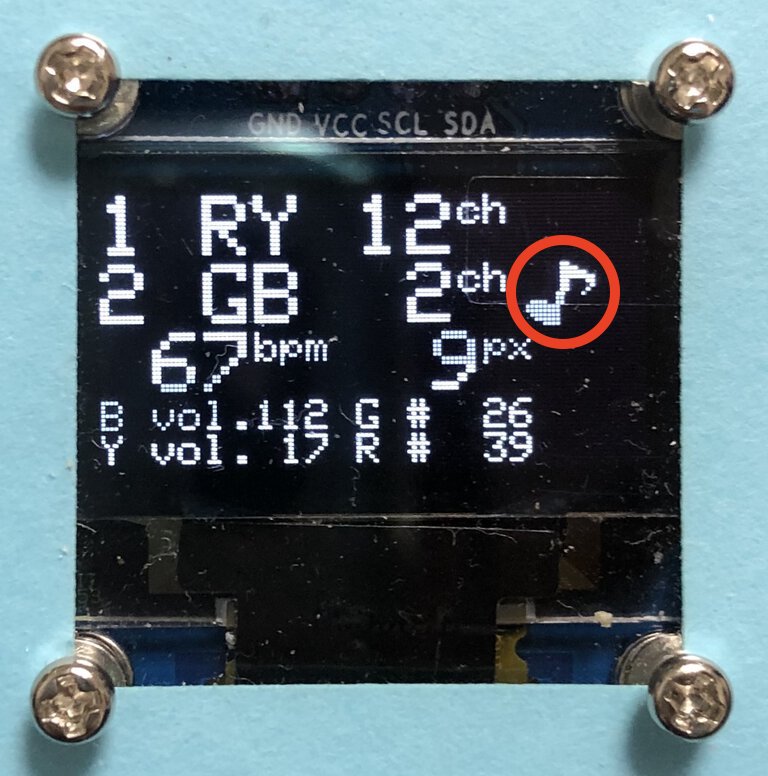
Reference
- Weekly Assignment
- Week14 (Networking and communications)
- Wire library reference (Arduino)
- megaTinyCore I2C support
- ArduinoでOLEDディスプレイを試す -- library is updated and some of description is not relevant for June 2016
- Wire.h
- Adafruit_GFX.h (ver. 1.8.4)
- Adafruit_SSD1306.h (ver. 2.3.0)