Interface and Application Programming
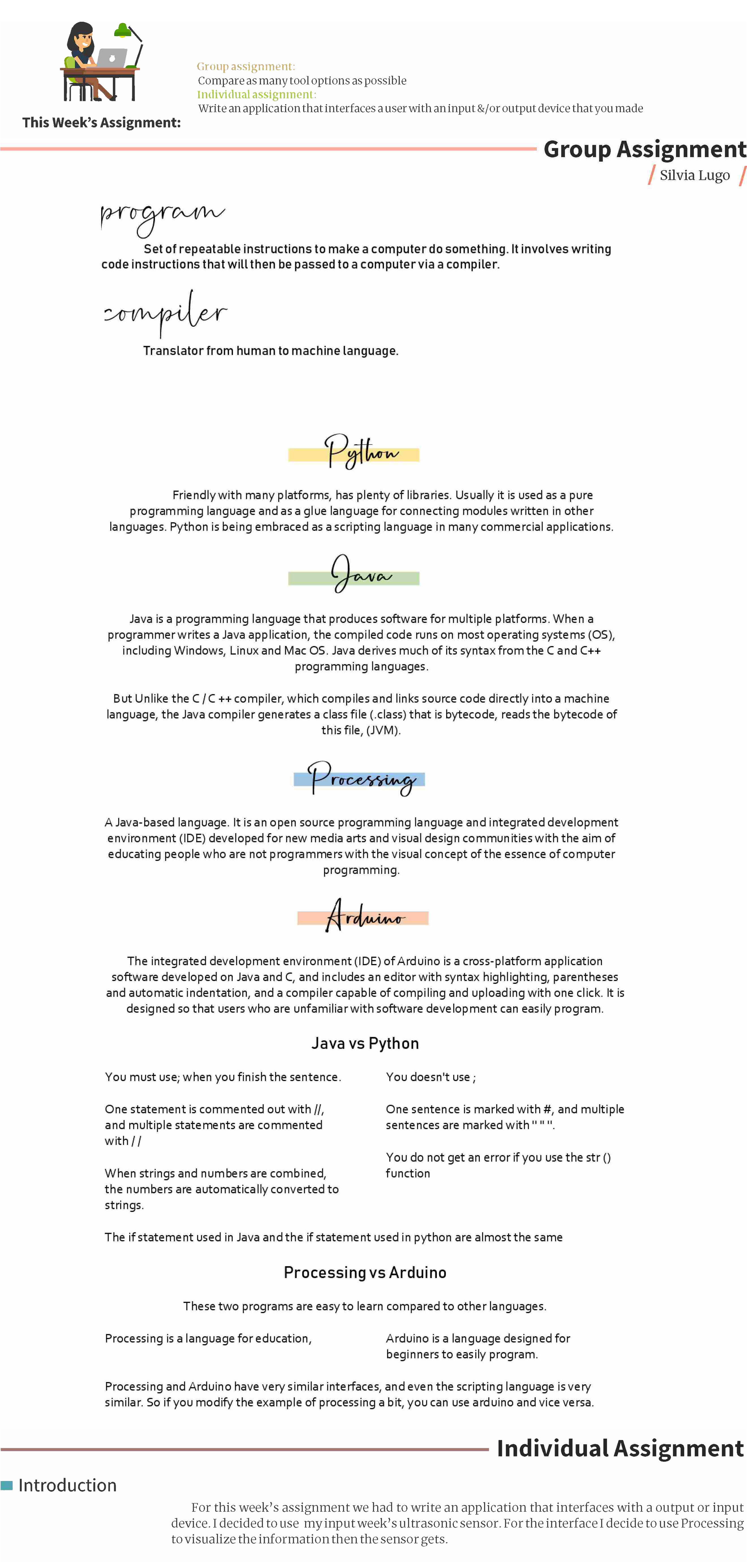
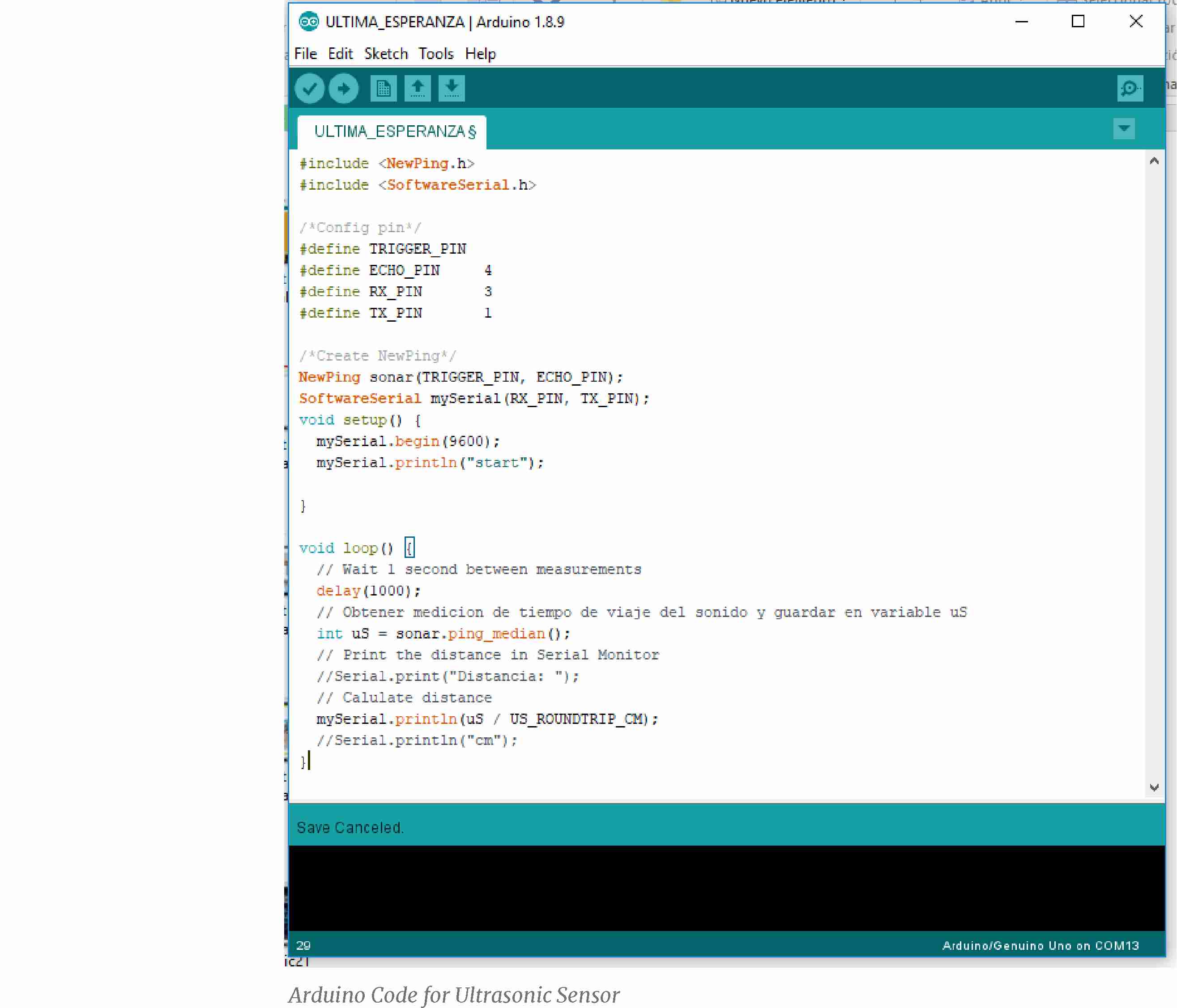
#include < NewPing.h>
#include < SoftwareSerial.h>
/*Config of pins*/
#define TRIGGER_PIN 9
#define ECHO_PIN 8
#define RX_PIN 0
#define TX_PIN 1
/*Create NewPing*/
NewPing sonar(TRIGGER_PIN, ECHO_PIN);
SoftwareSerial mySerial(RX_PIN, TX_PIN);
void setup() {
mySerial.begin(9600);
mySerial.println("start");
}
void loop() {
// Wait 1 second between measurements
delay(1000);
// Obtener medicion de tiempo de viaje del sonido y guardar en variable uS
int uS = sonar.ping_median();
// Print the distance in Serial Monitor
//Serial.print("Distancia: ");
// Calulate distance
mySerial.println(uS / US_ROUNDTRIP_CM);
//Serial.println("cm");
}
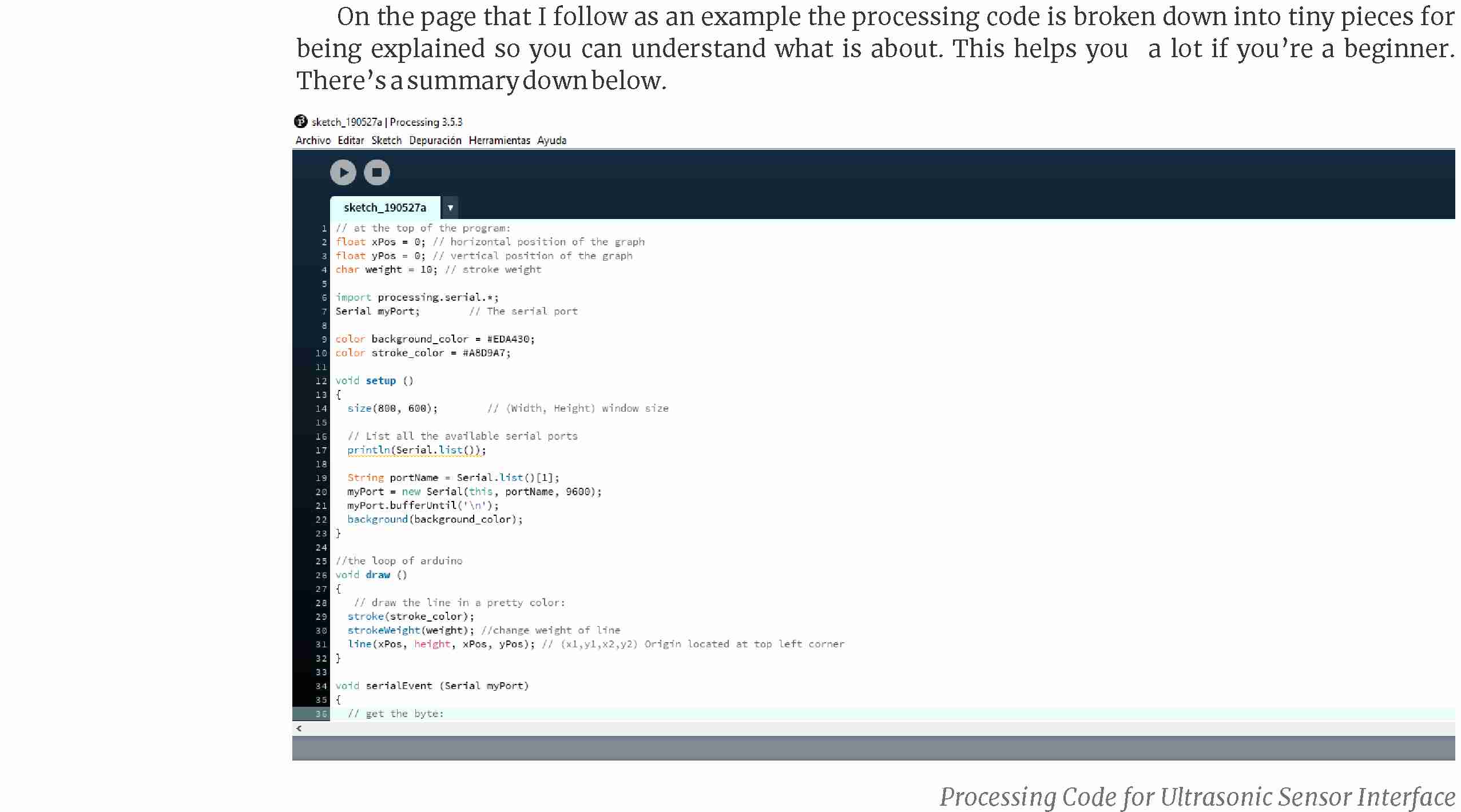
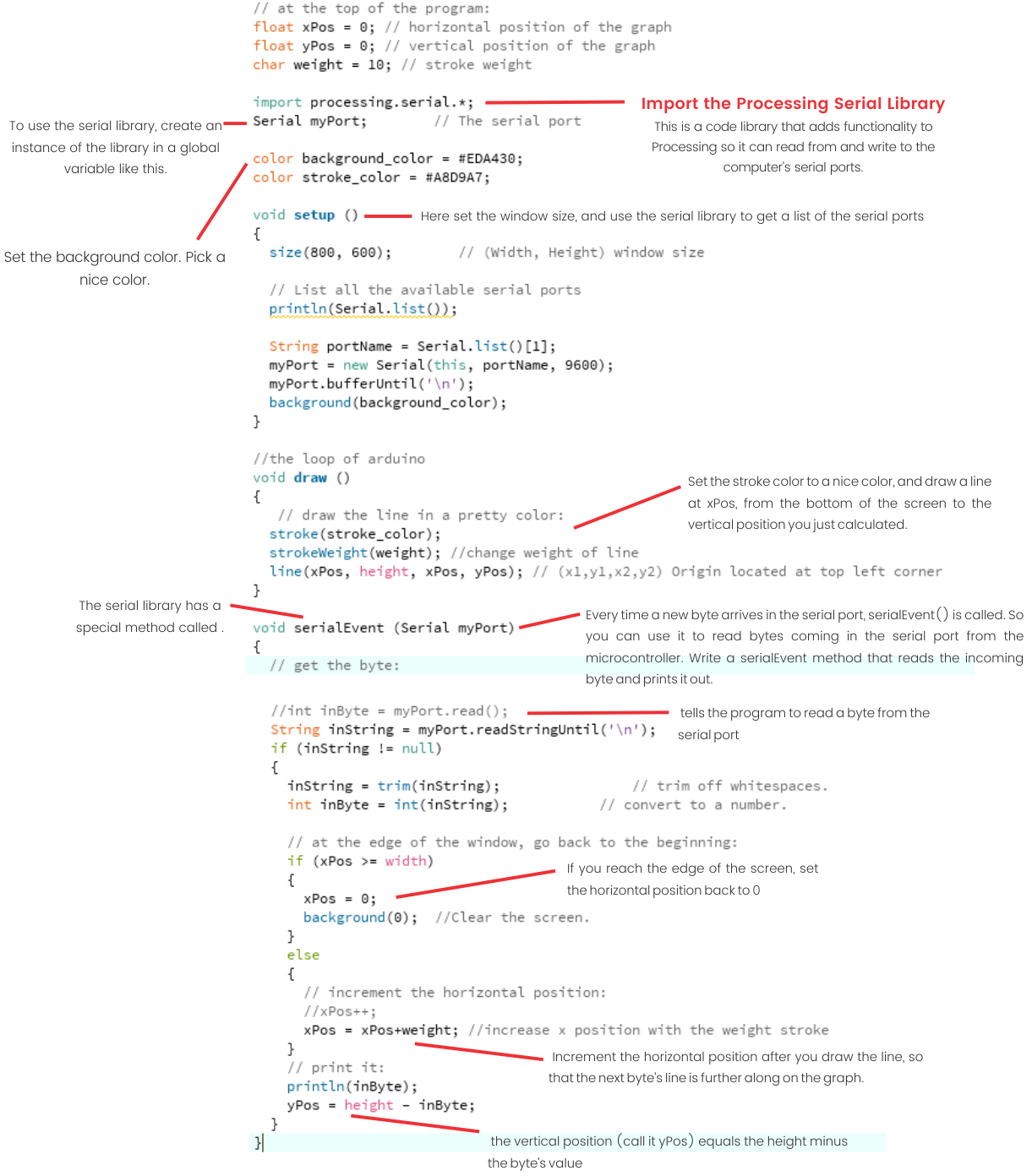
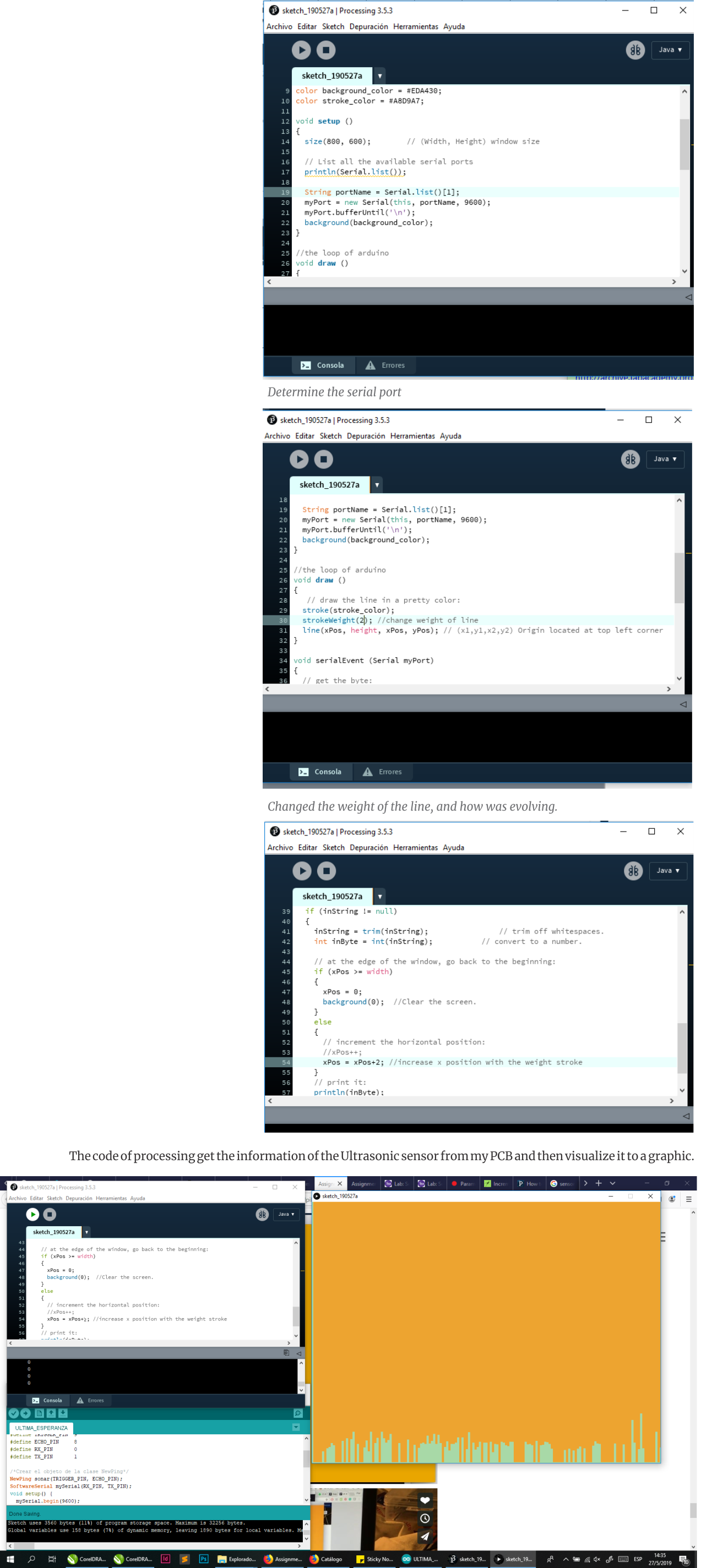
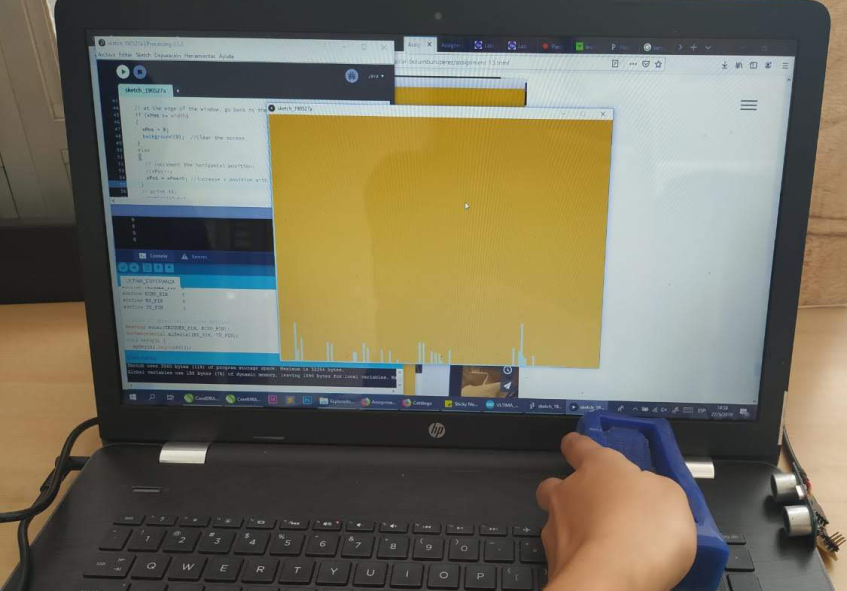
Final Code
// at the top of the program:
float xPos = 0; // horizontal position of the graph
float yPos = 0; // vertical position of the graph
char weight = 5; // stroke weight
import processing.serial.*;
Serial myPort; // The serial port
color background_color = #EDA430;
color stroke_color = #A8D9A7;
void setup ()
{
size(800, 600); // (Width, Height) window size
// List all the available serial ports
println(Serial.list());
String portName = Serial.list()[1];
myPort = new Serial(this, portName, 9600);
myPort.bufferUntil('\n');
background(background_color);
}
//the loop of arduino
void draw ()
{
// draw the line in a pretty color:
stroke(stroke_color);
strokeWeight(2); //change weight of line
line(xPos, height, xPos, yPos); // (x1,y1,x2,y2) Origin located at top left corner
}
void serialEvent (Serial myPort)
{
// get the byte:
//int inByte = myPort.read();
String inString = myPort.readStringUntil('\n');
if (inString != null)
{
inString = trim(inString); // trim off whitespaces.
int inByte = int(inString); // convert to a number.
// at the edge of the window, go back to the beginning:
if (xPos >= width)
{
xPos = 0;
background(0); //Clear the screen.
}
else
{
// increment the horizontal position:
//xPos++;
xPos = xPos+2; //increase x position with the weight stroke
}
// print it:
println(inByte);
yPos = height - inByte;
}
}
Testing interface using ultrasonic sensor from Silvia Lugo on Vimeo.