Week 2: Project management
01/29/2019 - Stéphane Muller
This week's assignment was to build a personal website, upload it to the class archive on Gitlab and work through a git tutorial to learn about version control.
Developping the website
I already have a good grasp of HTML, CSS and JavaScript, so I was able to dive right into it. My first step was to find a nice template I could use and then customize it. I chose the Strata template designed by HTML5UP. It’s simple, beautiful, the code is clean and it’s fully responsive.
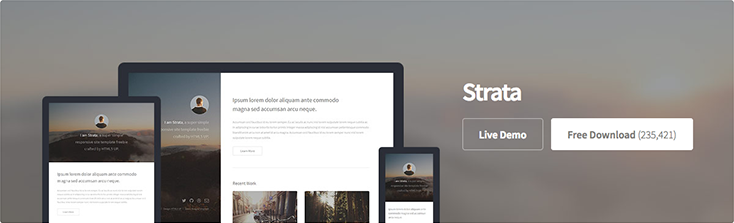
I started by analyzing the code and customizing a few simple elements like the header, the footer and the first paragraph “About me…”.
To have a clean code and be able to update the header and footer easily without having to update each page manually, I added a JavaScript function to emulate an include method and just have to type the following:
<div w3-include-html="./header.html"></div>
The “About me…” paragraph seemed a bit long so I added a jQuery library “Show more/less” function I found and customized its look in the CSS.
.showmore-button {
cursor: pointer;
text-transform: uppercase;
font-size: 0.7em;
padding: 7px 5px 5px 5px;
margin-top: 5px;
}
.showmore-button a {
color: #787878;
}
To use this plugin I had to add the script to the html and call the function, like so:
<script src="assets/js/jquery.show-more.js"></script>
$('#aboutme').showMore({
minheight: 150,
buttontxtmore: 'show more',
buttontxtless: 'show less',
animationspeed: 500
});
I also added 2 new classes to the CSS to create warning and tip blocks.
.tip {
border-left: solid 6px #25BA4A;
background-color: #DFF5E4;
font-style: italic;
margin: 0 0 2em 0;
padding: 0.5em 0 0.5em 1.5em;
}
.warning {
border-left: solid 6px #EB8C21;
background-color: #F5E9DC;
font-style: italic;
margin: 0 0 2em 0;
padding: 0.5em 0 0.5em 1.5em;
}
To make the meaning of those blocks clearer I wanted to use little icons like these but I didn't want to use pictures. So I found a way to embed them inline with a simple HTML tag:
<i class="fa fa-exclamation-triangle" aria-hidden="true"></i>
One way I think I'm going to improve the site in future versions is by using Jekyll to make a real template and be able to focus more on the content.
Version control with Git
Although I knew how to code, I have to confess I had never used git. So after reading the Gitlab documentation, a quick tutorial (in French) and with a lot of help from my teammate Madjid, I was able to synchronize a directory on my computer with my Fab Academy Gitlab account.
Because I had already used xcode (Apple's IDE) on my computer, git was already installed. But I made sure by I had the latest version by using this command in the terminal:
$ git --version
git version 2.17.2 (Apple Git-113)
You can use a GUI with git but since I'm already familiar with the command line I thought I'd start learning the commands straight away. First thing you need to do is set up your environment, so I started by doing some basic configuration:
$ git config --global user.name "Stéphane Muller"
$ git config --global user.email "muller.stephane@gmail.com"
Then I had to define the working directory on my computer, so I navigated to it within the terminal and cloned my Gitlab repository like so:
$ git clone https://gitlab.fabcloud.org/academany/fabacademy/2019/labs/sorbonne/students/stephane-muller
Cloning a repo downloads the files on your computer and connects it with the remote directory.
Next I needed to understand how the most common commands worked. Git uses a transition state to list all modified files, so first you need to add the files you modified to this list. Then you compile the list of changes with a commit. And finally you push the changes on the repository, which basically uploads the files. So here is the basic workflow.
Start by pulling (downloading) the latest version of the project on your repo before doing some changes:
$ git status
Check the status of your local directory compared to the last saved copy on the repo:
$ git pull
Once I forgot I had made some changes directly in Gitlab and the push I did from my computer wouldn't work. So I did a pull but the command wouldn't work either. It took me some time to figure out I had to do a commit on my computer first.
If you're confident about all the changes you've made (much easier when you work alone of course), you can add all your changes at once:
$ git add .
Commit those changes:
$ git commit -m "Commit description here."
And finally push them on the repo:
$ git push
I just registered a domain name (http://fabnhack.net) for this website and wanted to publish it simultaneously via FTP, so I added the code below to the .gitlab-ci.yml file. That way, as soon as I push new pages or code to my Gitlab repo master branch, it automatically updates my website as well.
ftp:
stage: deploy
script:
- apk update
- apk add lftp
- lftp -c "set ftp:ssl-allow no; open -u $USERNAME,$PASSWORD $HOST; mirror -Rev ./ ./www --ignore-time --parallel=10 --exclude-glob .git* --exclude .git/"
only:
- master
Git is a powerful and complex program and there are many things I still need to explore, like branches and conflicts for example. Yet I feel like for now, to start working on my own project, this workflow and my understanding of it is enough. I have a good idea of git's possibilities and will learn new commands as the various projects require and the problems arise.