Week 9: Embedded Programming
Assignment
☑ Read a Microcontroller Datasheet
☑ Group: compare the performance and development workflows for other architectures
☑ Program your board to do something
☑ EXTRA: Make a Fabkit/Shashakit/Fabio
BRAINSTORMING
Read a Microcontroller Datasheet
1.1.1 VCC
Supply voltage.
1.1.2 GND
Ground.
1.1.3 Port B (PB3:PB0)
Port B is a 4-bit bi-directional I/O port with internal pull-up resistors (selected for each bit). The Port B output buffers have symmetrical drive characteristics with both high sink and source capability except PB3 which has the RESET capability. To use pin PB3 as an I/O pin, instead of RESET pin, program (‘0’) RSTDISBL fuse. As inputs, Port B pins that are externally pulled low will source current if the pull-up resistors are activated. The Port B pins are tri-stated when a reset condition becomes active, even if the clock is not running.
I organized the pin out datasheet to be able to find things quicker.
so far, all I know is that the brown ones and the blue ones are important to me right now.
because I will need to look at them when I am coding with Arduino.
[What I learned this week from class]
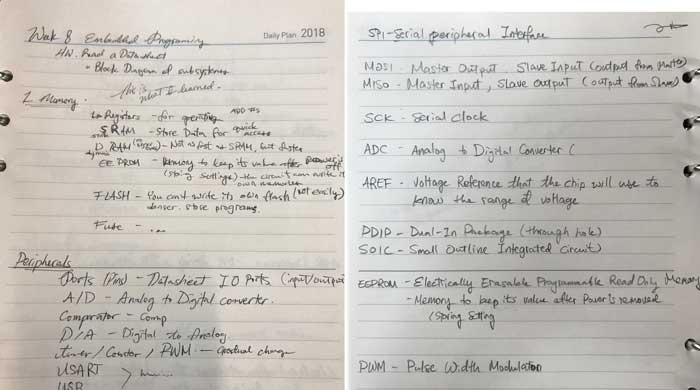
There are butt ton of information that I had to learn this week without actually making sense of them all.
(I am hoping that in the future I will be able to understand some of it)
Compare other architectures
I did not have a group to look through other architectures together, so I looked through other classmates to get some more information. I was able to get a lot of help from my friends Pamela, Flavie in Oshanghai, and Rico in Kamakura! Pamela's Documentation
Rico's Documentation
Flavie's Documentation
Program your board to do something
I had programmed my board to do stuff couple weeks ago.
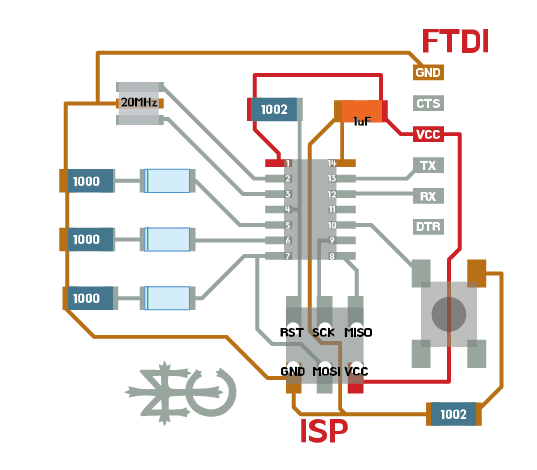
I tried using [#define] for naming your pins, and [if] & [else] code.
Extra: Make a Fabkit/Shashakit/Fabio
I decided to make a Fabkit because it looked most compact and square.
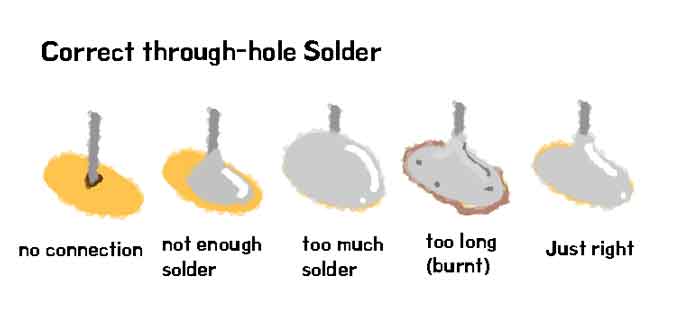
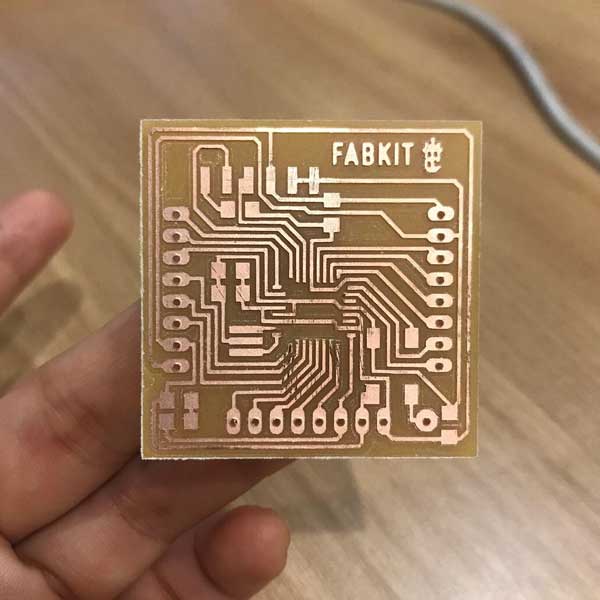
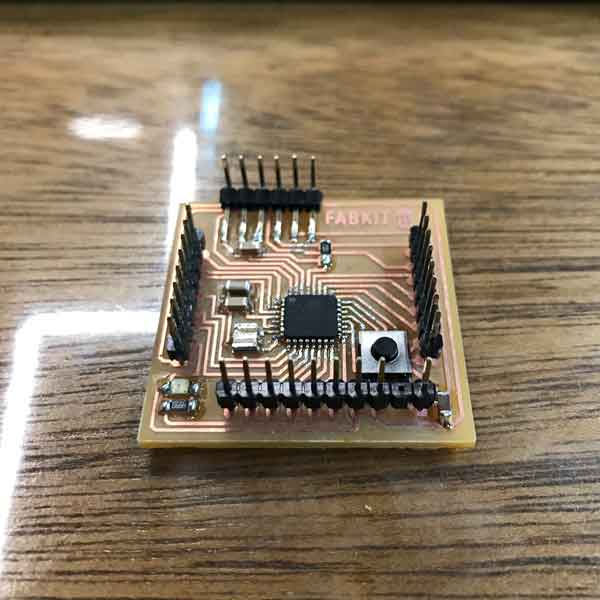
in some arears around the pins for ATMega so that I wouldn't have to do a lot of manual cutting. I had soldered on everything before understanding what each pins were...
so I was bound to have problems. (READ important datasheets and schematics!!!)
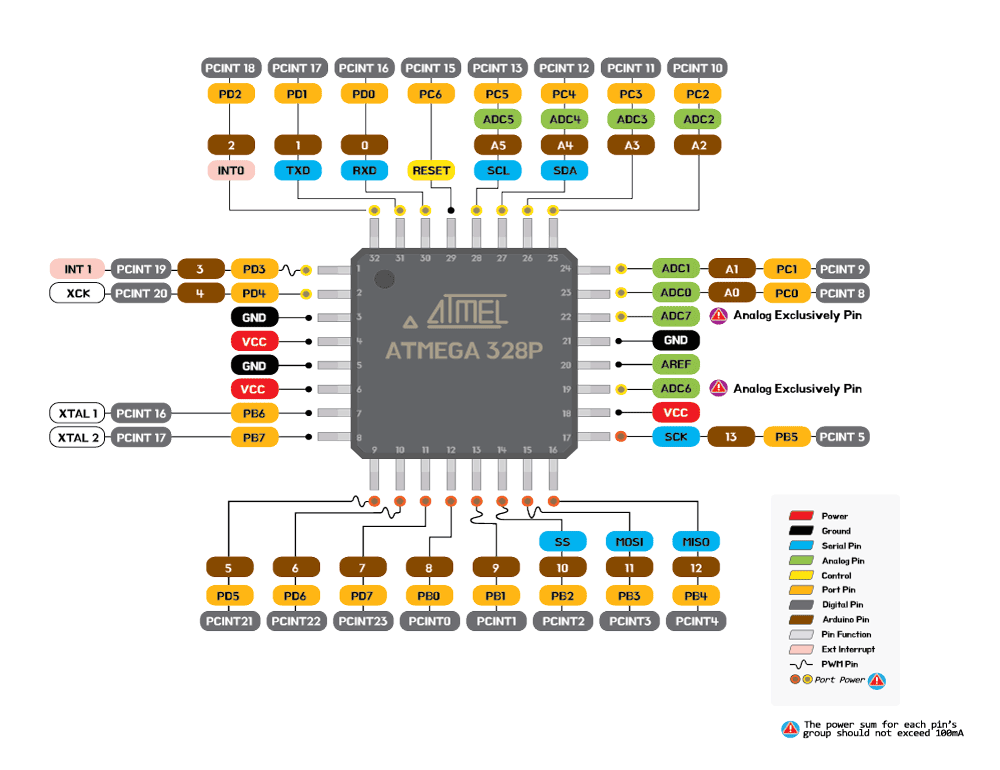
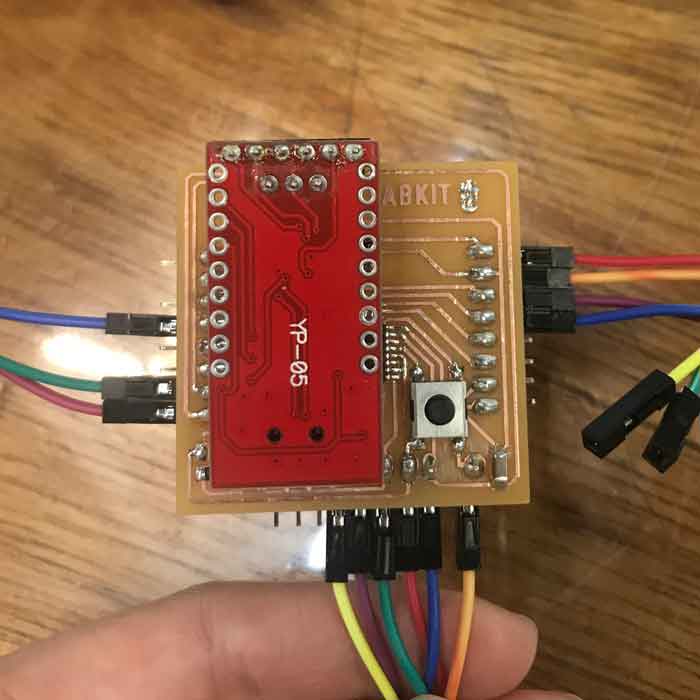
(NOW I know that all I needed was to change the connecting pins of the FTDI, but I was pretty close to burning it.)
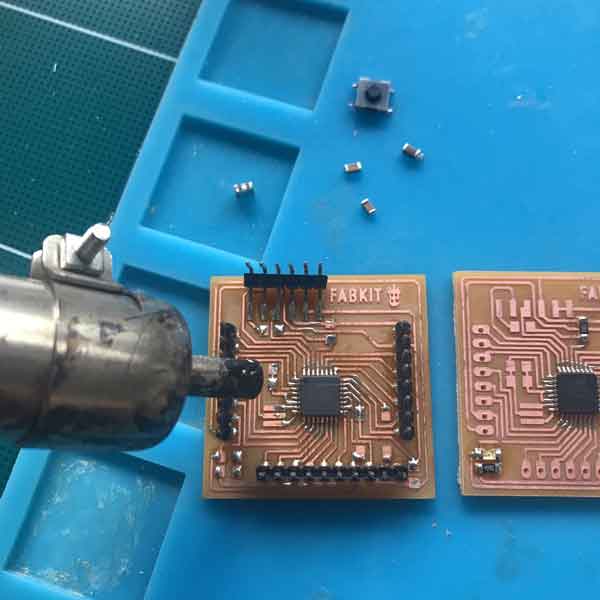
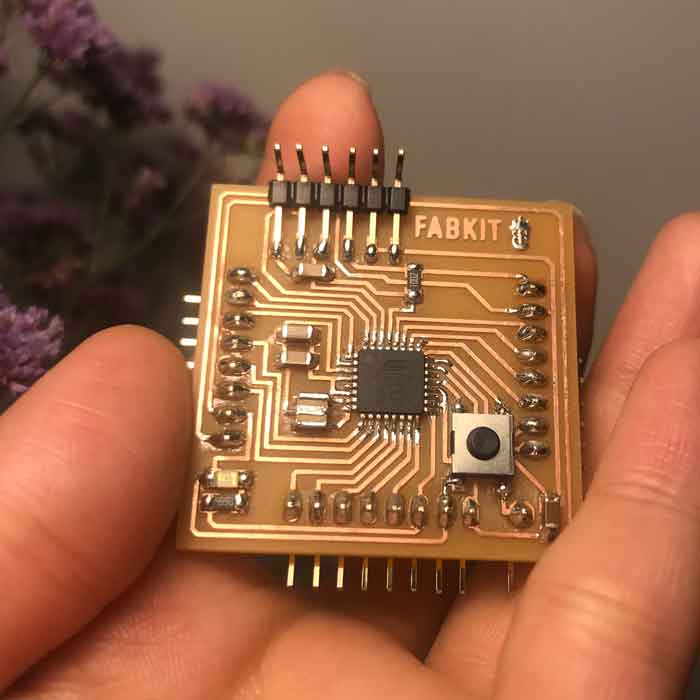
Programming
Connect your newly made Fabkit to your microcontroller and to your computer.
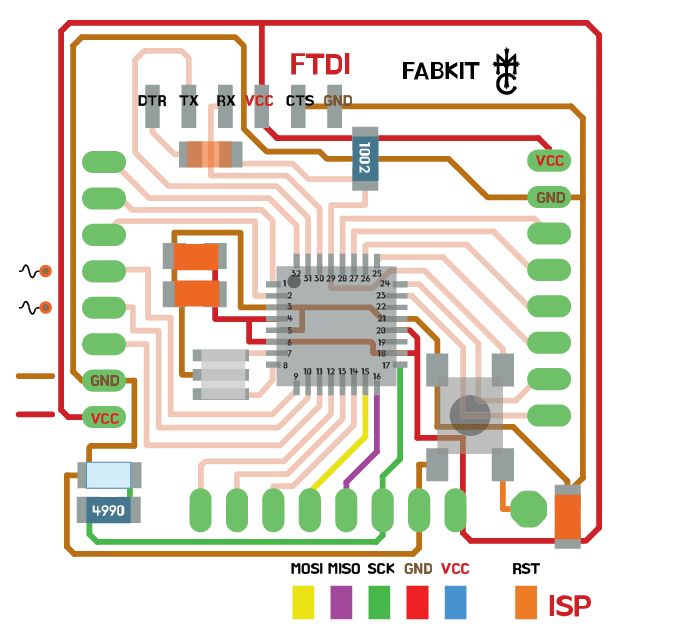
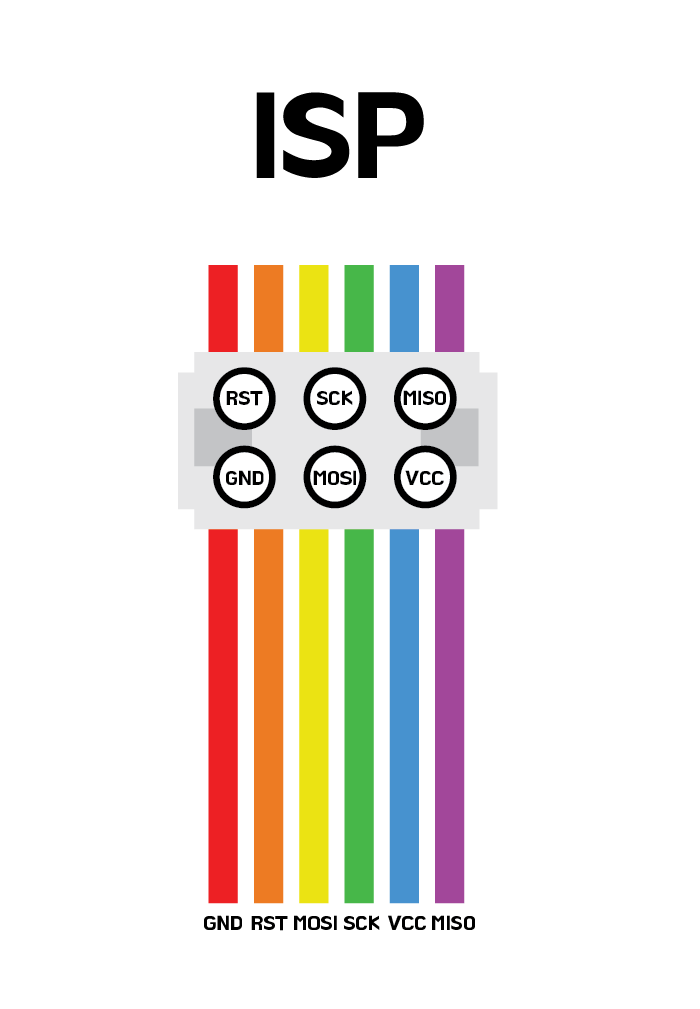
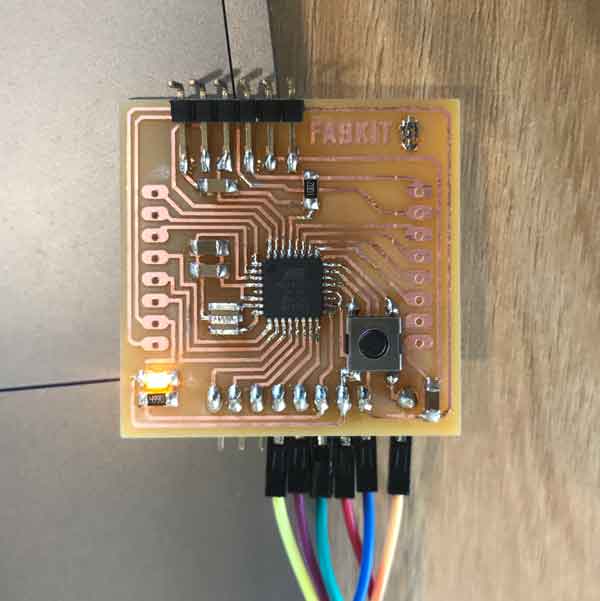
Open Arduino
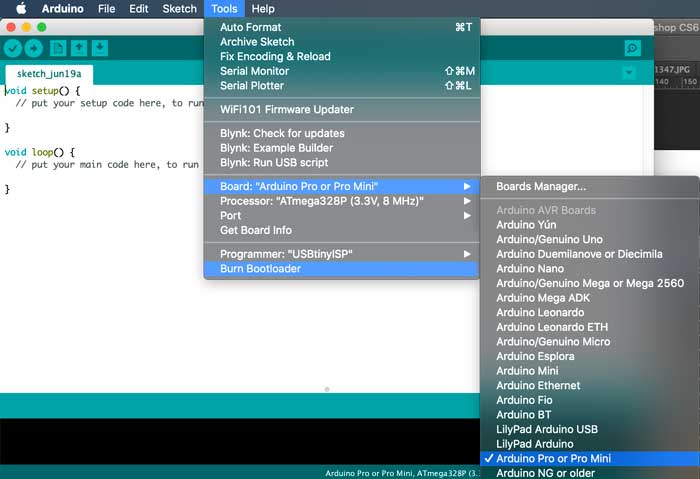
Trouble
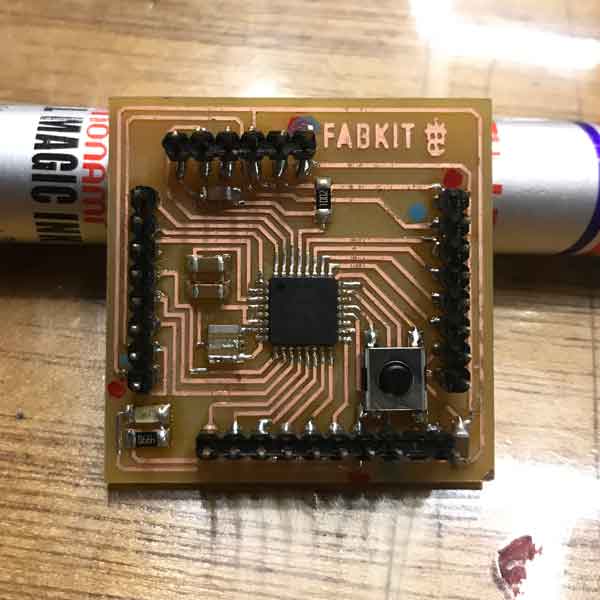