In this weeks assignment I wanted to programm an flex sensor so it uses an analog signal to control an brushless motor. I will use it in my final project as a pedal to accelerate the gokart.
Schematic
I will use my board with the atmega328p chipset.
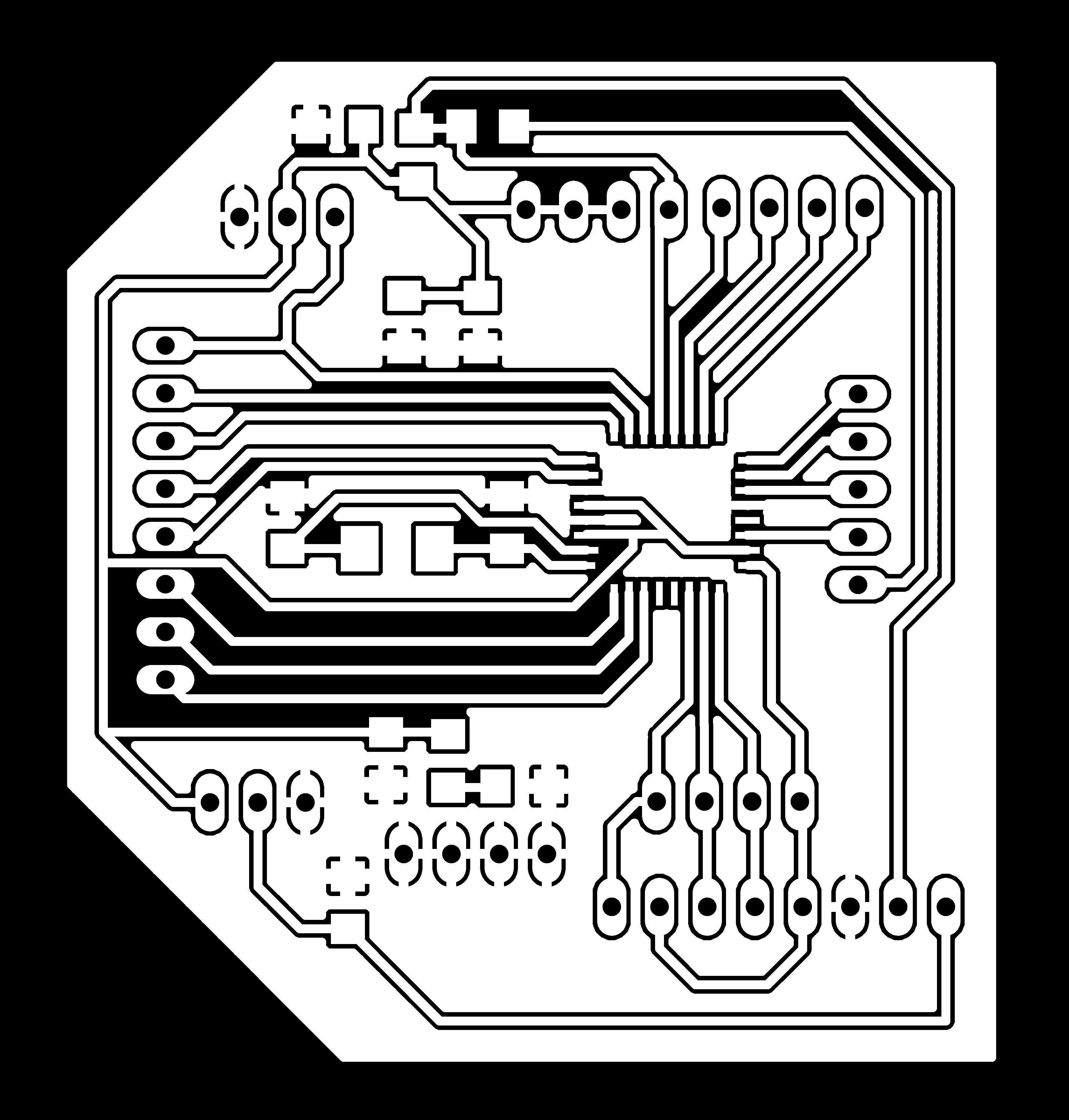
Since the flex sensor will give us an analog output, I will have to attach it to one of the analog pins. Through the datasheet I learned that the pin 23 is a ADC (analog to digital converter) pin which works at 10-bit accuracy and the microcontroller in general has 8 ADC channels. I followed this schematic.
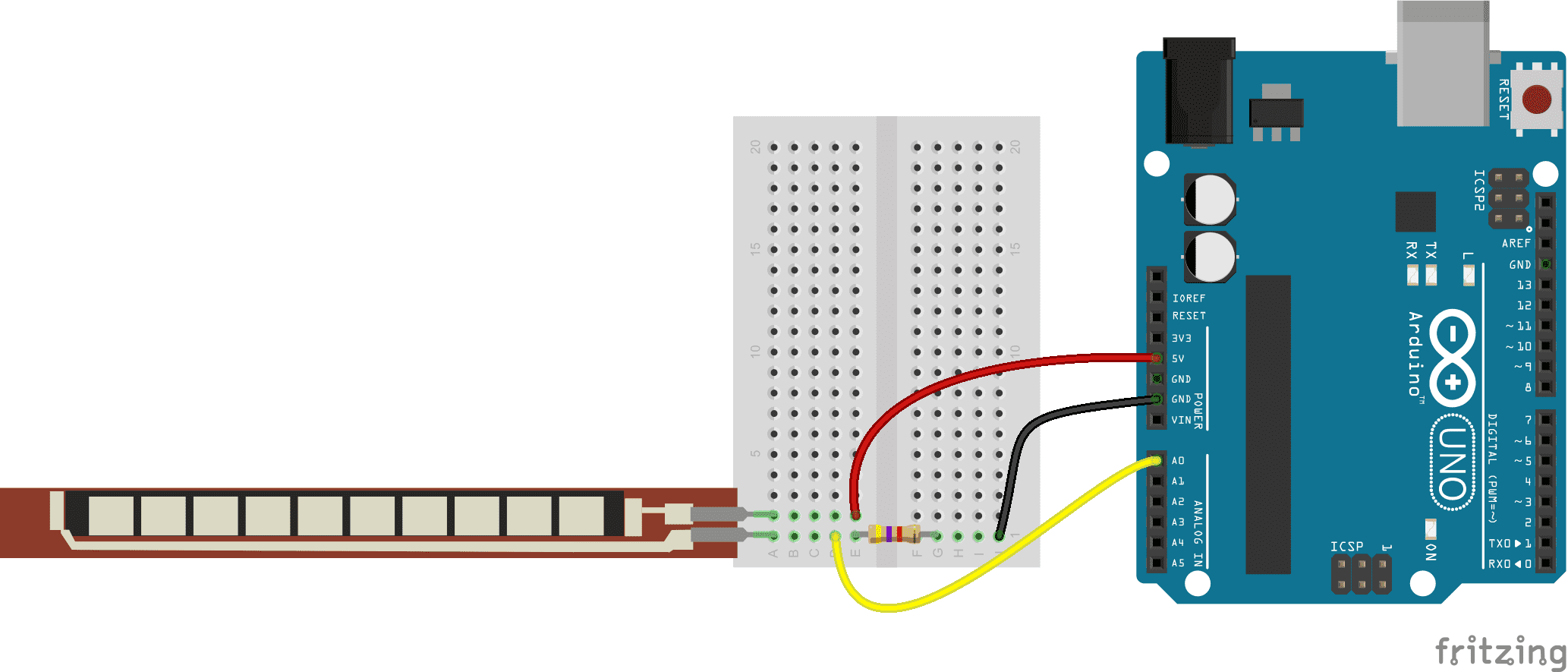
Arduino Code
Connected right, I have used this code
#include
int value=70; //Creating a value, could be 0 also
const int flexPin = A0; //initialize the analog pin 0 (A0) as int "flexPin"(Here we attach the sensor)
const int motor=11; //initialize the pin 11 as int "motor"(here I will attach the motor)
void setup() {
Serial.begin(9600); //begin serial on baud 9600
pinMode(motor,OUTPUT); //setting pinmode of pin 11/motor to output, because the motor requires a output signal
}
void loop() {
value = analogRead(flexPin); //initilize value with the outcome of the sensor using analogRead() function
value = map(value, 150, 254, 300, 150); //mapping the value to 150-300, because thats the range where the motor will start moving when writing on it
analogWrite(motor, value); //analogWrite on the motor to speed up using the value of our sensor
}
h3>PYTHON ON ARDUINO
I will write the same code in python to compare it to the arduino ide. But to do that I had to upload firmata to the arduino first. Luckily it is already present in the examples, so I just navigate to File -> Examples -> Firmata -> StandardFirmata.
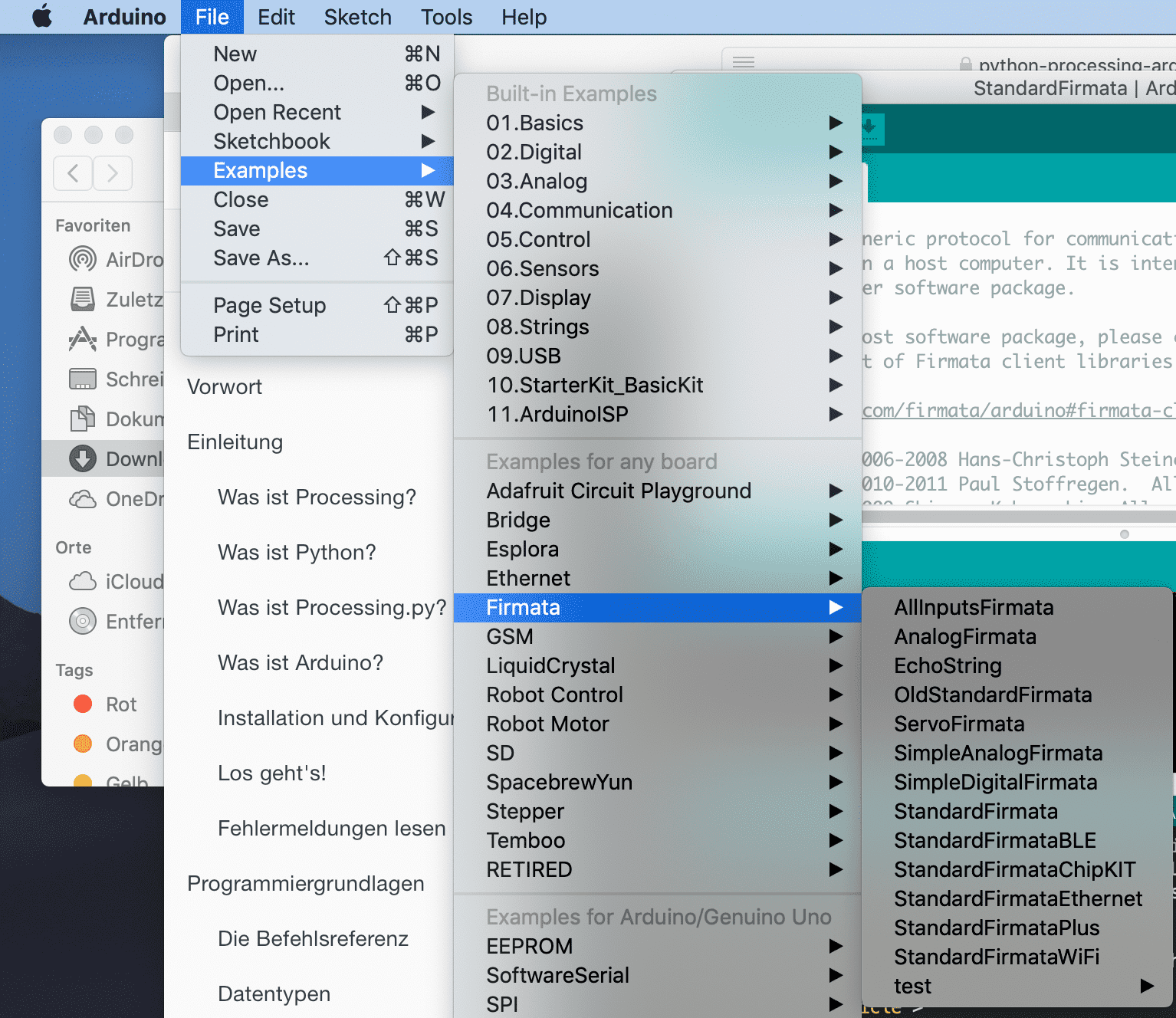
Then I will go on using processing to code in python, because the arduino editor doesn't support pyhton code. Processing is a ide with a own development envoirement, which supports a lot of programming languages, you can download processing following this link.
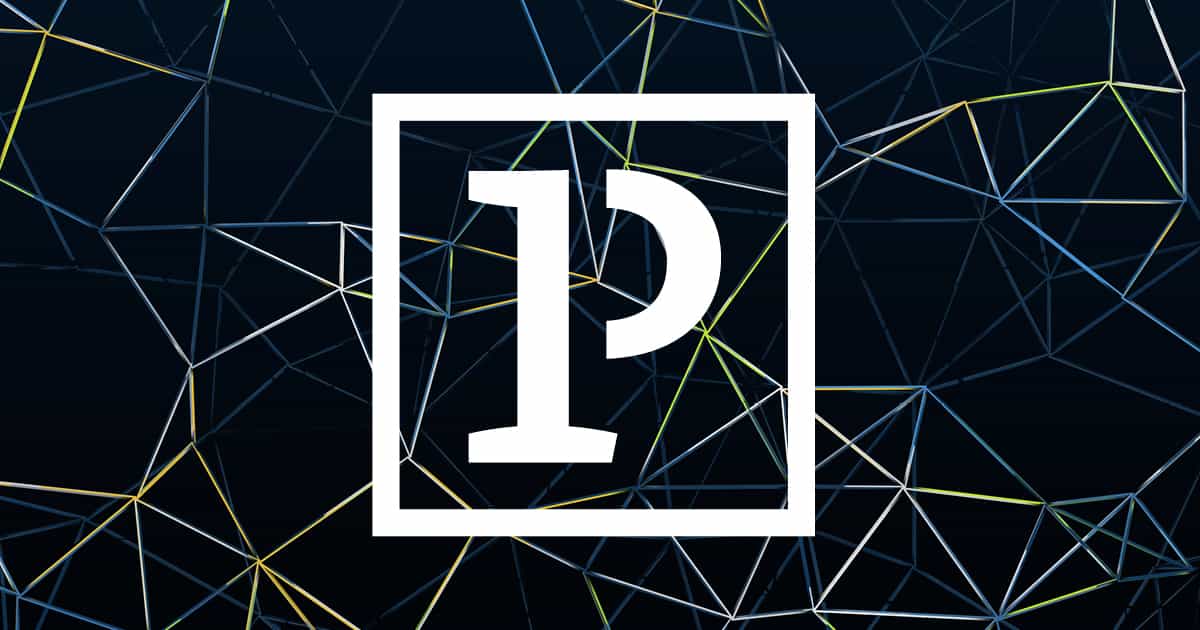
To get started with processing, I needed to install the pyhton package first by simply navigating to the "Java" button on the top right side.
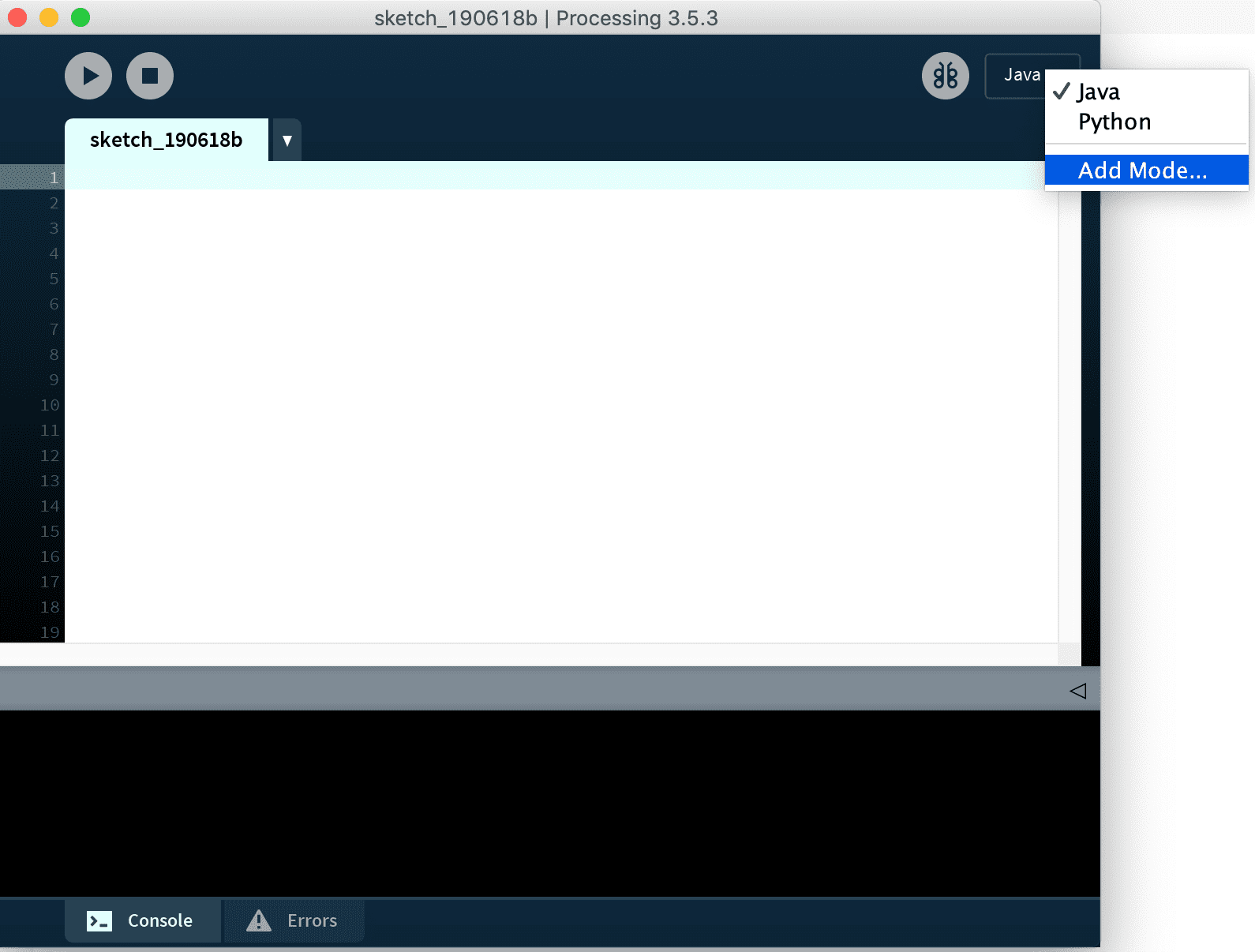
A window will pop up, in this library manager I needed to install the pyhton package for processing
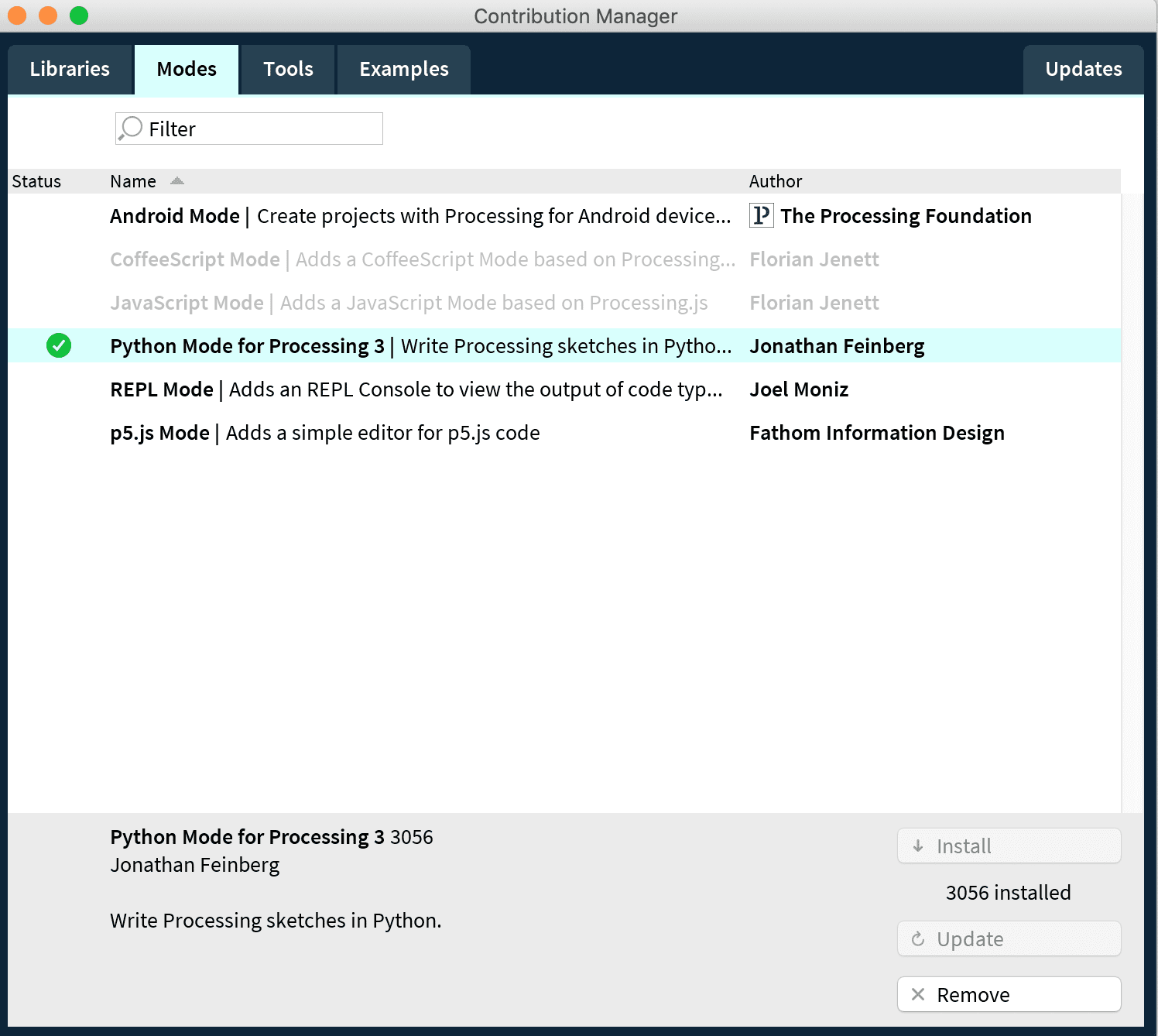
Now we can select python on the top right side and start to write code in python.
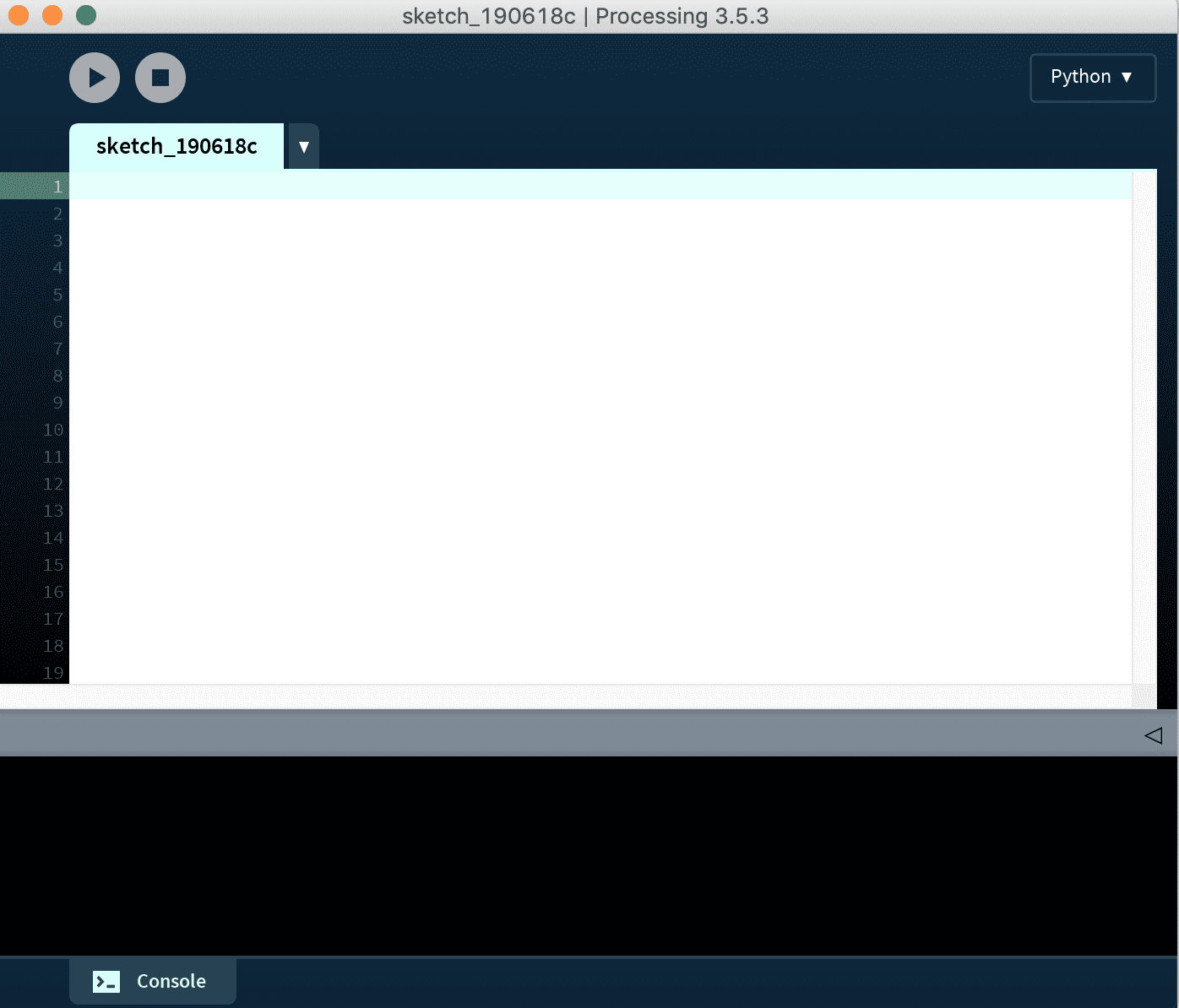
To communicate with the arduino, I needed to install the arduino library using the package installer. Just navigate to Sketch -> Library -> Add library
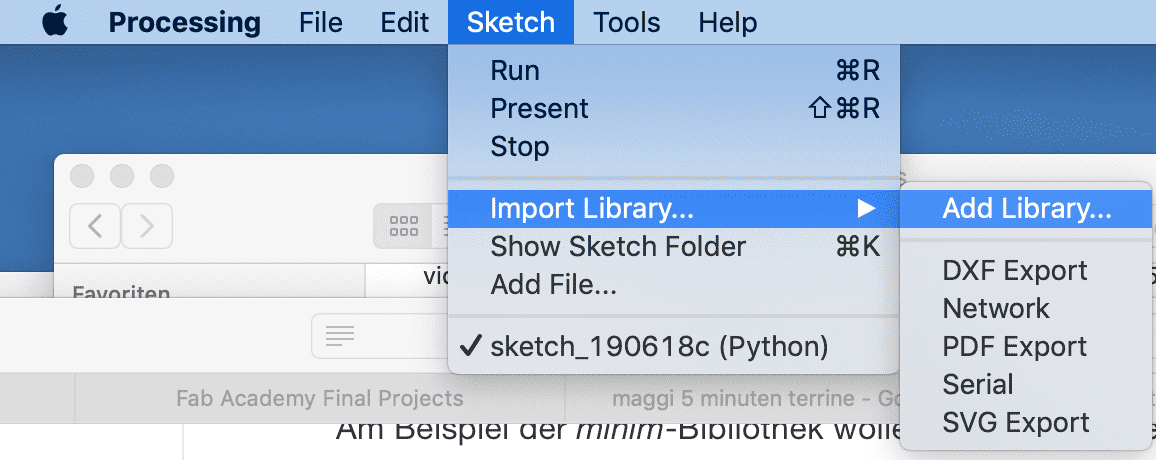
The package library manager opens where you can install the arduino package
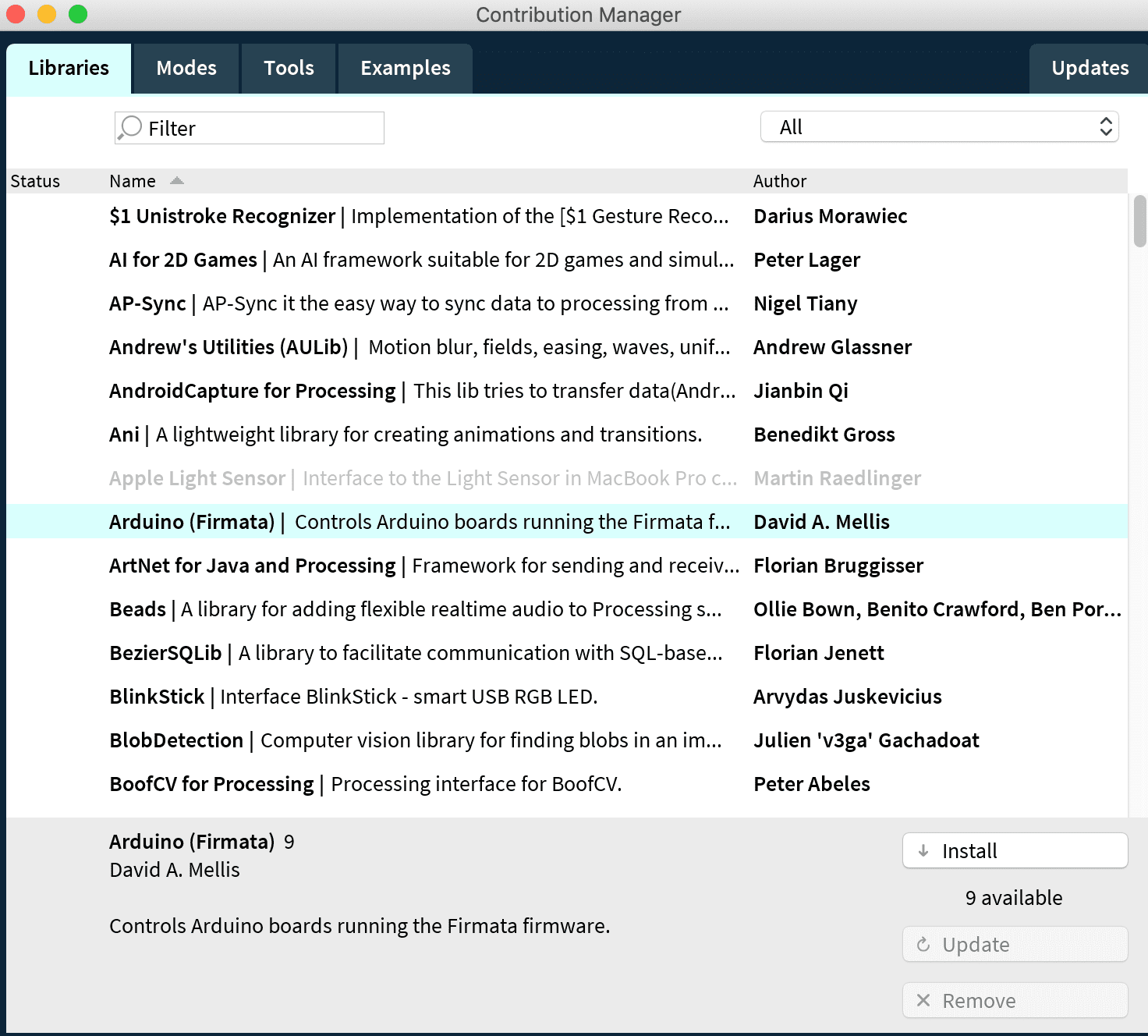
Now I wrote the code in python
add_library('serial')
add_library('arduino') #necessary libraries
flexPin = 0 //flexsensor on pin 0
a = Arduino(this, '/dev/cu.usbserial-141320',57600) #selecting the port
motor = 11 //motor on pin 11
def setup():
a.pinMode(flexPin, Arduino.INPUT) #flexpin as input
a.pinMode(motor, Arduino.OUTPUT) #motorpin as output
value = a.analogRead(flexPin) #read analog values of flex sensor
a.analogWrite(motor, value ) #write the analog value to the motor to start it
def draw():
pass
I realized that some functions such as the mapping will not work as I used them in the arduino ide, so I skipped that part and concentrated on the analog read and write.
<
In the following video you can see how the motor works using the flex sensor