In this weeks assignment I wanted to do a authentication system using a rfid scanner and little interface for this authentication. I will use this authentication system in my final project, the rfid tag will have the same function as a car key.
Arduino code
In order to use the rfid scanner as an authentication system I had to scan and save an rfid card that would be recognized when scanning that card. Like in the networking and communication week I will connect an RFID-RC522 scanner to my atmega328p board.
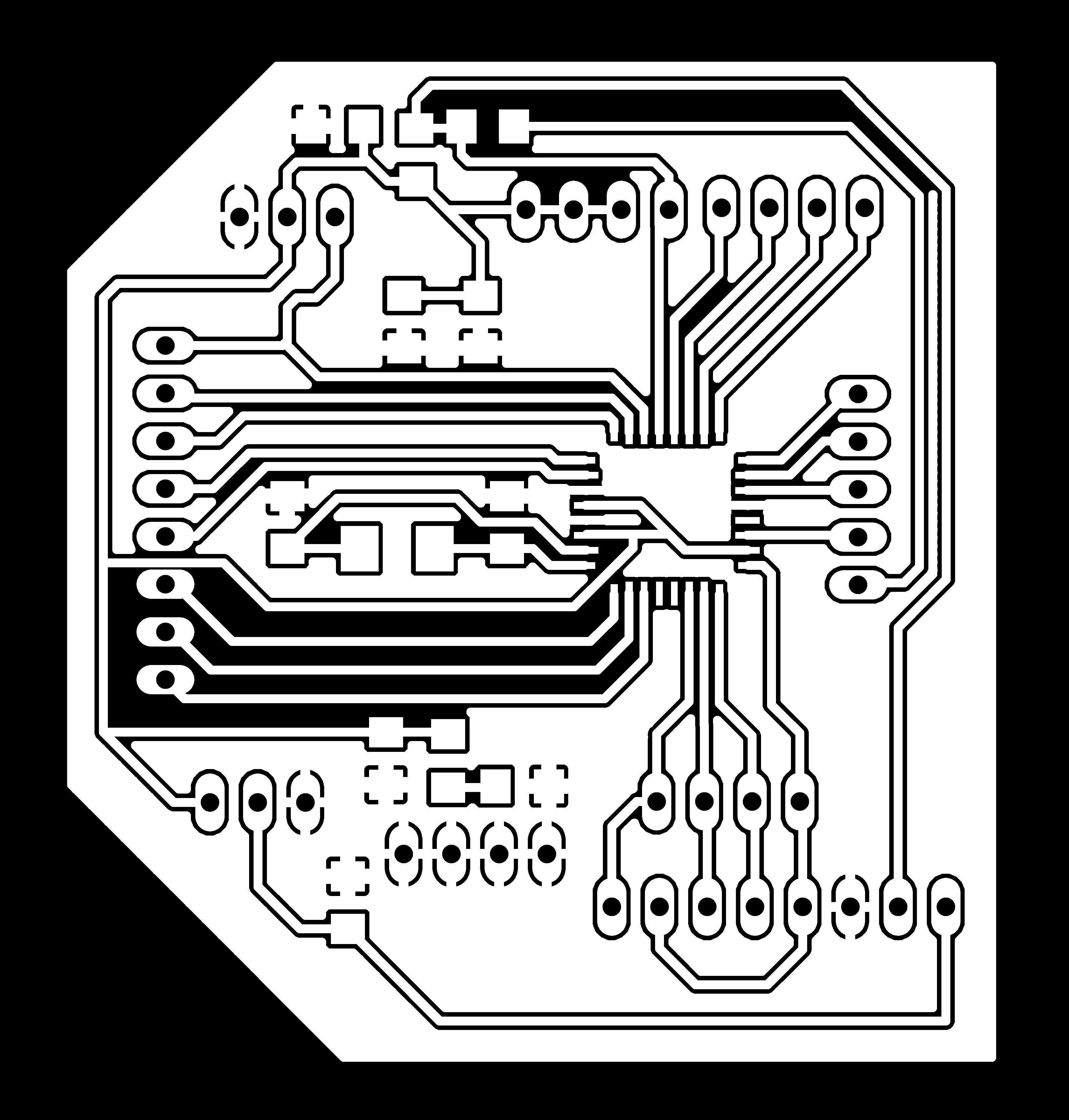
Now I was able to upload a code for the MFRC522 to check if it really workd and is connected right.
#include
#include
constexpr uint8_t RST_PIN = 9; // Configurable, see typical pin layout above
constexpr uint8_t SS_PIN = 10; // Configurable, see typical pin layout above
MFRC522 rfid(SS_PIN, RST_PIN); // Instance of the class
MFRC522::MIFARE_Key key;
// Init array that will store new NUID
byte nuidPICC[4];
void setup() {
Serial.begin(115200);
SPI.begin(); // Init SPI bus
rfid.PCD_Init(); // Init MFRC522
for (byte i = 0; i < 6; i++) {
key.keyByte[i] = 0xFF;
}
}
void loop() {
// Look for new cards
if ( ! rfid.PICC_IsNewCardPresent())
return;
// Verify if the NUID has been readed
if ( ! rfid.PICC_ReadCardSerial())
return;
// Serial.print(F("PICC type: "));
MFRC522::PICC_Type piccType = rfid.PICC_GetType(rfid.uid.sak);
//Serial.println(rfid.PICC_GetTypeName(piccType));
// Check is the PICC of Classic MIFARE type
if (piccType != MFRC522::PICC_TYPE_MIFARE_MINI &&
piccType != MFRC522::PICC_TYPE_MIFARE_1K &&
piccType != MFRC522::PICC_TYPE_MIFARE_4K) {
//Serial.println(F("Your tag is not of type MIFARE Classic."));
return;
}
//Check if the scanned tag has the same NUID by comparing the array with the actual numbers
if (rfid.uid.uidByte[0] == 201 &&
rfid.uid.uidByte[1] == 211 &&
rfid.uid.uidByte[2] == 124 &&
rfid.uid.uidByte[3] == 91 ) {
//print hello message
Serial.println(F("Hello Master!"));
// Store NUID into nuidPICC array
for (byte i = 0; i < 4; i++) {
nuidPICC[i] = rfid.uid.uidByte[i];
}
}
//Check if the scanned tag has the same NUID by comparing the array with the actual numbers
else if (rfid.uid.uidByte[0] == 36 &&
rfid.uid.uidByte[1] == 159 &&
rfid.uid.uidByte[2] == 223 &&
rfid.uid.uidByte[3] == 43 ) {
//Print hello message
Serial.println(F("Hello Mr.Rustamov!"));
// Store NUID into nuidPICC array
for (byte i = 0; i < 4; i++) {
nuidPICC[i] = rfid.uid.uidByte[i];
}
}
///Check if scanned tag has a difference to the master tags
else if (rfid.uid.uidByte[0] != 201 ||
rfid.uid.uidByte[0] != 36 ||
rfid.uid.uidByte[1] != 211 ||
rfid.uid.uidByte[1] != 159 ||
rfid.uid.uidByte[2] != 124 ||
rfid.uid.uidByte[2] != 223 ||
rfid.uid.uidByte[3] != 91 ||
rfid.uid.uidByte[3] != 43
) {
// Store NUID into nuidPICC array
for (byte i = 0; i < 4; i++) {
nuidPICC[i] = rfid.uid.uidByte[i];
}
//Giving an error message because the tag is not a master tag
Serial.println(F("Invalid Card!"));
}
//Scanning a new tag
if (rfid.uid.uidByte[0] != nuidPICC[0] ||
rfid.uid.uidByte[1] != nuidPICC[1] ||
rfid.uid.uidByte[2] != nuidPICC[2] ||
rfid.uid.uidByte[3] != nuidPICC[3] ) {
// Store NUID into nuidPICC array
for (byte i = 0; i < 4; i++) {
nuidPICC[i] = rfid.uid.uidByte[i];
}
}
// Halt PICC
rfid.PICC_HaltA();
// Stop encryption on PCD
rfid.PCD_StopCrypto1();
}
/**
* Helper routine to dump a byte array as hex values to Serial.
*/
void printHex(byte *buffer, byte bufferSize) {
for (byte i = 0; i < bufferSize; i++) {
Serial.print(buffer[i] < 0x10 ? " 0" : " ");
Serial.print(buffer[i], HEX);
}
}
/**
* Helper routine to dump a byte array as dec values to Serial.
*/
void printDec(byte *buffer, byte bufferSize) {
for (byte i = 0; i < bufferSize; i++) {
Serial.print(buffer[i] < 0x10 ? " 0" : " ");
Serial.print(buffer[i], DEC);
}
}
My code is based on the tutorial of Circuits4You.
Python code
I wanted to do a simple window which shows which tag is scanned and then shows a nice little welcome message
When executing the code, a small window appears with the text "Waiting for authentcation"
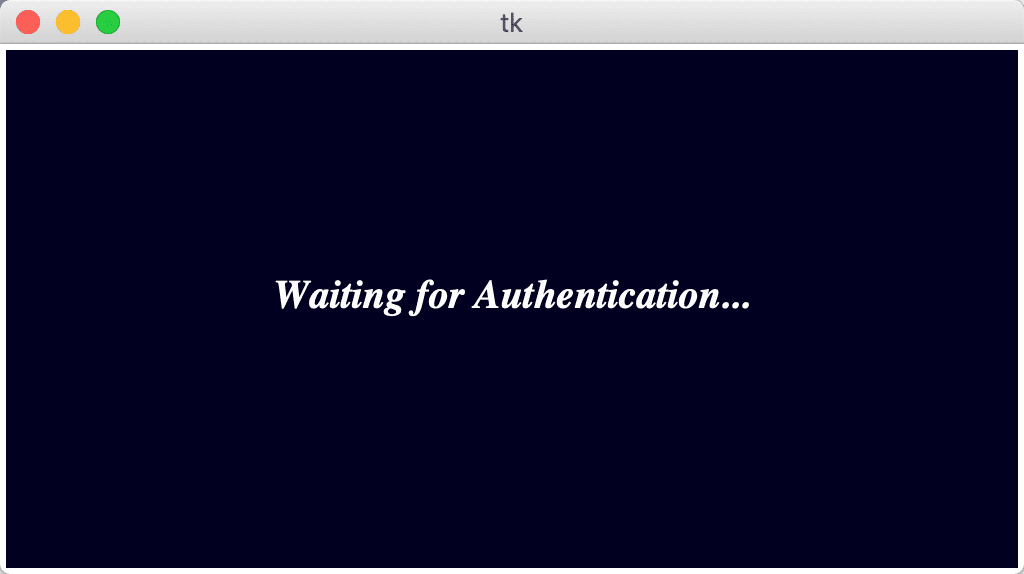
Now if I scan a random tag, the authentication fails.
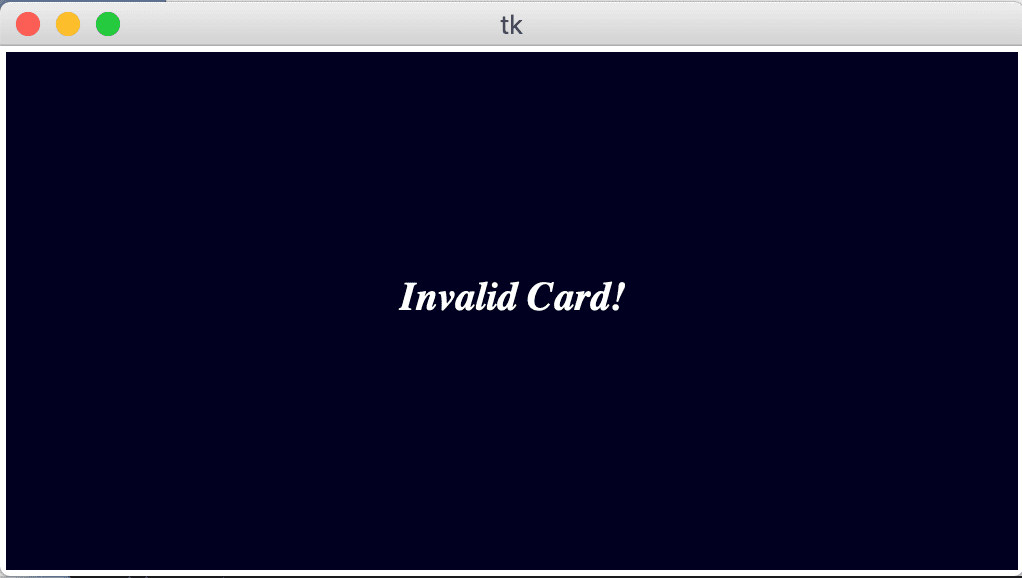
And when I scan my tag, the authentication is succesfull.
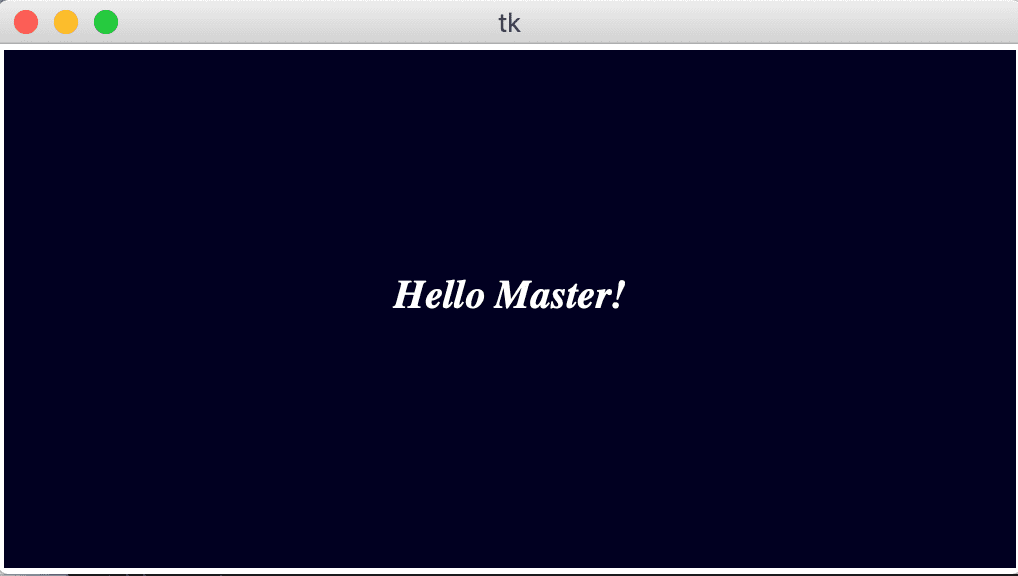
I used the python framework tkinter to display a gui.
#import serial and tkinter frameworks
import serial
from tkinter import *
#creating a plane window with tkinter
window = Tk()
#setting the size of the window
window.geometry('512x265')
#setting a canvas in window size and a color
canvas = Canvas(window, width=512, height=265, bg='#002')
canvas.pack()
#creating a text setting size, font and the written text
textc = canvas.create_text(256,125,fill="#FEFDFC",font="Times 20 italic bold",text="Waiting for Authentication...")
#adding the port on which my board is connected with the serial baud
mySerial = serial.Serial('/dev/cu.usbserial-141310',115200)
#endless loop
while True:
#updating the window
window.update()
#reading serial outcome of the port
s = mySerial.readline()
#converting serial outcome to a string
s = str(s)
#printing the string in the console
print(s)
#cleaning the String from characters we do not want to display
s = s.replace("b","").replace("'","").replace("\\r\\n","")
print(s)
#converting the text to the new string
canvas.itemconfig(textc,text=s)
In the following video you can see the whole authentication process