WEEK 13
Interface & Application
Equipment
- Software: Arduino IDE, Processing
- Materials: Sonar Input Board
Processing
Processing is a programming language based on Java geared towards generating visual and interactive media.For my application program, I used Processing to create a sketch that would change colors based on the data received by my sonar sensor board from input week.
First, I had to change my code in Arduino IDE slightly before uploading it to my input microcontroller. Initially, it had some text such as "distance=" and "cm". These were removed so that the output would be purely numbers which could then be read by the Processing serial communication code.
Processing Code
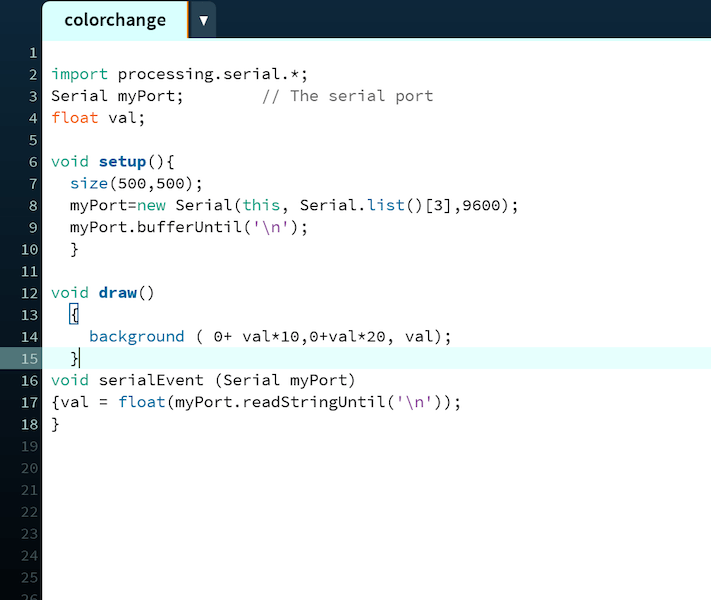
Add Serial library for Processing
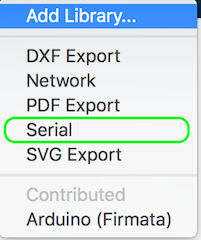
Since the sketch is reactive to the input from the sensor, we need to add the serial library in processing.
Define serial port: Serial myPort Define variables:
float val This makes sure that there are values contained within our data. Float is used in place of int when your variable contains a fractional value. val will be the data received from the sensor board via the serial port
<
void set up
myPort sets up the Serial Port and baud rate myPort.bufferUntil('\n')command waits for values to be received by the serial port. '\n' refers to new lines or new values read through the Arduino IDE Serial Monitor.void draw
This writes out what your visual output will be. In this case, I am just changing the colors of the background depending on the distance measurements of the sensor (val)void serial event
This defines our val as the string of data coming from the serial PortRotating Color Wheel
When I was using Processing the first time, I had the most trouble connecting with my ArduinoIDE to get data from the Serial Port. So when I came back to Processing this time, I thought the problem was out of the way and I could focus on just learning some more of the Processing language. I ran my old sketch again just to check all my hardware was working, and NOTHING. I hadn't changed anything from the first time I ran it, so this was pretty frustrating because this seems to keep happening to me. I checked everything again- my pin connections were fine and my serial monitor was getting readings from my sonar sensor. Since the last time my problems was naming my serial port from the serial list, I tried out different numbers. This time it was [4]. I thought I understood how to find out which port I was using, but I guess I'm missing something. At least it worked, so I continued trying to make a new sketch using the data from my sensor.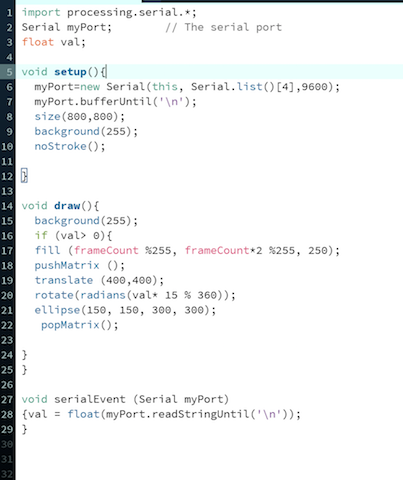
Add the serial library and set up the port that processing will be receiving serial data from.
void setup
Set up canvas size and backgroudn color. noStroke means that the shapes that I'm drawing will have just fill with no outlinevoid draw
By calling background in the beginning, this will wipe the canvas clean after every frame. If you don't call it, then all of the drawings from the the loop will remain on the canvas.If the val received from the sensor is greater than 0, it will run the following loop:
The fill color of my shape needs (R, G, B). I wanted the colors of shapes to change on a gradient, so at first I used val, data given by my sensor, but because of the sensitivity, it would get random spikes of a different color which I didn't like the effect of. The variable would need to be something sequentially stable with controlled increments, so frameCount worked well as a variable. It is based on a percentage of 255, because the color range value has a max of 255, but the frame count has no limit.
pushMatrix is used when you want to translate your grid in order to move things around on your canvas. When using translate you claim how many x, y pixels you want to move from the origin.
Then we will rotate our shape using radians. Here is where I used the variable val so that my shape would rotate along the circle based on the distance perceived by my sensor. We again use a percentage value this time in relation to the degrees along a circle. Ellipse will draw my circle shape (x, y, height, width)
popMatrix will restore the old grid
TroubleShooting
-
Gray Box when running sketch
I was running sketches with no error messages, but was just getting a gray screen. I was a bit unsure if I was defining my serialPort correctly and it took me a while to figure out this small thing. For most computers, it will use the first USB port as the serial port, which is why I found that when looking at most other people's code they defined it similarly
Serial Port [0]: Most common
Because I was using a USB hub, this was not the case for me. To find the serial port thought was actually super simple. In Arduino, you can go to the dropdown tools and literally count down to see which port it's using. Keep in mind that the first port is counted as 0, so the fourth one down will be port 3. In processing, put in the correct serial port.