Classes > Input Devices
For this assignment I wanted to start using some of the electronics I'm designing for my final project, so I'm not using AVR microcontrollers but MSP430 ones.
MSP430 is a family of microcontrollers by texas instruments, they are 16 bit, ultra-low power, and have a wide range of processors from really small to very powerful ones with lots of preripherals. But most important, they are supported by opensource tools like GCC compiler and GNU binutils, mspdebug for programming and debugging, and if you want a full-featured IDE, Eclipse supports MSP430 with all bells and whistles.
Texas Instruments are really into supporting hobbysts, so some months ago they started to sell the MSP430 Launchpad, a board with integrated programmer and debugger, power supply, usb-serial converter, and some LEDs and switches, something like a MSP430 "arduino", The board comes with two MSP430 microcontrollers, an extra crystal, male and female headers, and some documentation, all for 4.30$ including shipping. So, really nice and cheap microcontrollers, really nice and cheap prototyping platform, and open-source tools. Who can say no?
So, for my project, I'll be using the MSP430g2553 microcontroller, 16K Flash and 512bytes RAM, with uart/spi/i2c/irda, two 16bit timers, lots of analog and pwm channels, and so on. You can see the full datasheet at Ti's website. At the software side you need to install msp430-gcc and msp430 binutils and mspdebug.
My final project will be reading data from the environment in it's flight. One data I want to read is external temperature. I need a temperature sensor with a big range as at 30km up you can be at -40ºC. So first I tried using the LM35, an analog temperature sensor, with a nice range from -55 to 150ºC and a linear output of 10mV per 1ºC.
Datasheet: LM35.
So reading the temperature from the LM35 is easy, I just need to read the value from one ADC channel and use a simple calculation to get the temperature, as the sensor outputs 10mV per ºC, you just need to multiply the ADC output value for the VCC (3.6V on the launchpad) and for 100, and then divide it for 1024 (10-bit ADC resolution).
Then I wrote a simple program to read the ADC, and if the temperature is bigger than 30º I turn on the LED1 on the launchpad. I can warm the LM35 with my fingers to test the program. It worked.Code:
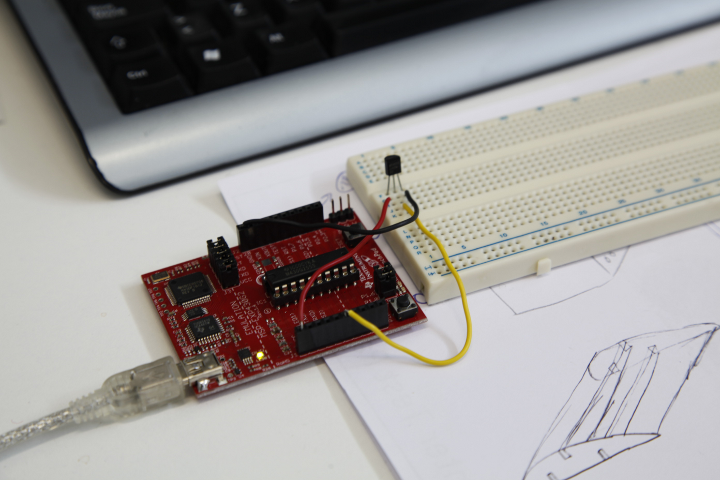
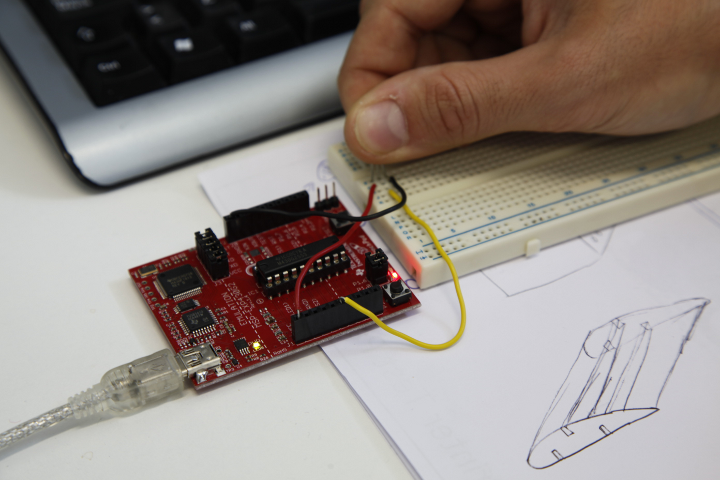
I also wanted to use a digital sensor, so I don't need to compesate an analog reading based on environmental parameters like temperature, battery voltage and so on. So I choosed the DS1624+, a temperature sensor by Maxim with a i2c interface, and with a range from -55°C to +125°C, in 0.03125°C increments (-67°F to +257°F in 0.05625°F increments).
So first I needed to talk i2c. The MSP430g2553 has hardware support for i2c communication, but as I want to use the USCI (the hardware that controls the UART/SPI/I2C...) for UART to talk to the GPS and GPRS modules of my final project, I needed to write a software i2c routine. The i2c protocol is really simple, and for controlling most of the i2c devices you don't have to implement all of the i2c protocol. So after looking at some code for software i2c for avr or pic microcontrollers, I wrote routines for my MSP430.
Then using this routines, I wrote some code for the DS1624 sensor, to read the temperature data and convert it to celsius degrees.
And finally I wrote a really simple program to test everything, that reads the temperature from the sensor and if it is higher than 25ºC, it turns on the LED1 on the launchpad board. After some fixes and some debugging using my logic analyzer, everything works as expected.
Code:
After finishing this I discovered that the USCI of the MSP430g2553 has two channels, USCIA and USCIB, so I can talk hardware UART and I2C at the same time using different pins. So I'll rewrite the i2c routines to make use of the hardware support in the microcontroller.